filmov
tv
Rust Book Club #3: Common Concepts
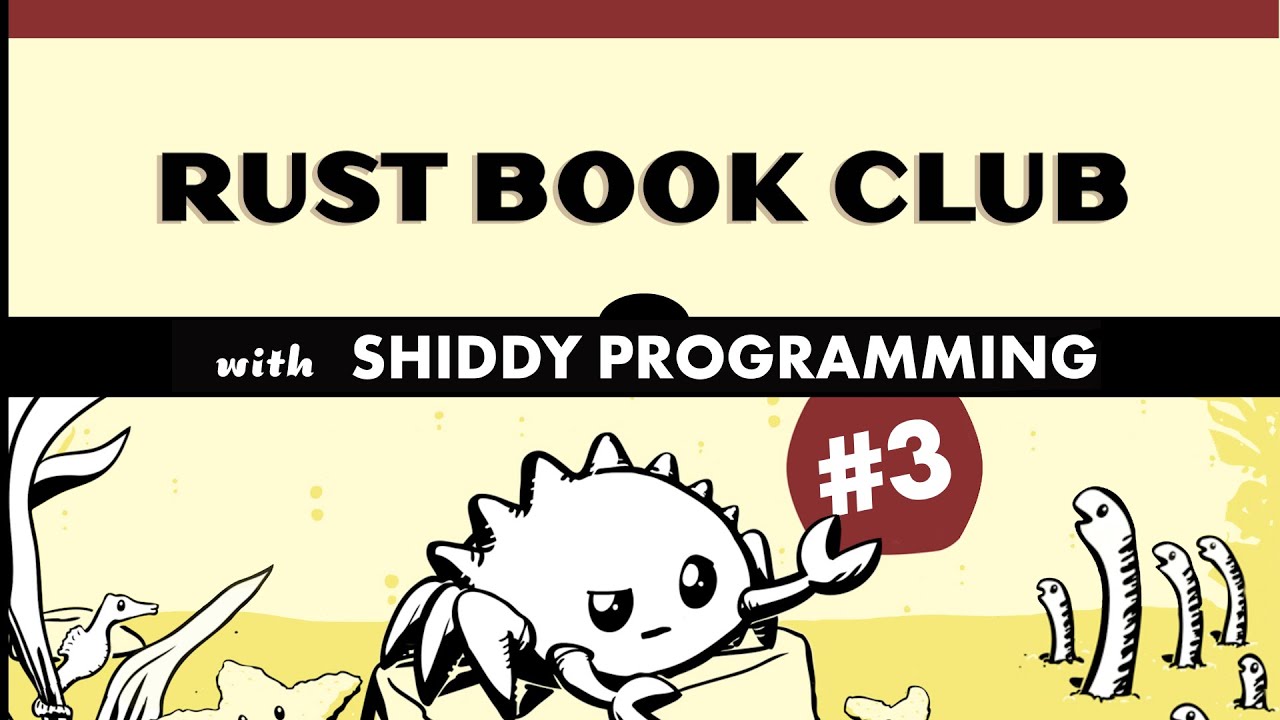
Показать описание
Watch someone stumble through a technical book without the polish you expect. The perfect exercise of schadenfreude and/or masochism.
If you want to learn rust, but don't have friends to talk about it with along the way, hopefully this helps you and you can feel more confident in your learning process.
Rad! 4K and a better camera mount for less shaky footage! This includes a fair bit of efforts on the editing too. As always if you have any comments that can help me improve let me know.
0:00 - Intro
0:30 - The Strength of "Shadow"
3:26 - Constants Vs Immutable Variables
4:50 - Overflowing in Production
5:58 - Unicode as a default
6:44 - Compound crash-course
8:28 - Is Vec a bad default for me?
9:10 - Unexpected Compound Syntax
9:28 - Control Flow & Polymorphism
10:21 - Expressions Vs Statements
12:40 - Implicit and Explicit Returns
13:08 - Control Flow loops are Expressions
13:34 - Changing Iterables during an Iteration
15:42 - Modulos
16:16 - Compiled Language Advantages
17:26 - Truthyness
18:10 - TERMINAL TIME
18:24 - Can we always shadow variables?
19:57 - Can we use polymorphic functions?
20:01 - Purposely Thrashing the Borrow Checker
23:45 - Accept the Borrow Checker warnings
25:15 - Outro
_ Source Material _
The Rust Programming Language by Steve Klabnik and Carol Nichols
______ Links ______
If you want to learn rust, but don't have friends to talk about it with along the way, hopefully this helps you and you can feel more confident in your learning process.
Rad! 4K and a better camera mount for less shaky footage! This includes a fair bit of efforts on the editing too. As always if you have any comments that can help me improve let me know.
0:00 - Intro
0:30 - The Strength of "Shadow"
3:26 - Constants Vs Immutable Variables
4:50 - Overflowing in Production
5:58 - Unicode as a default
6:44 - Compound crash-course
8:28 - Is Vec a bad default for me?
9:10 - Unexpected Compound Syntax
9:28 - Control Flow & Polymorphism
10:21 - Expressions Vs Statements
12:40 - Implicit and Explicit Returns
13:08 - Control Flow loops are Expressions
13:34 - Changing Iterables during an Iteration
15:42 - Modulos
16:16 - Compiled Language Advantages
17:26 - Truthyness
18:10 - TERMINAL TIME
18:24 - Can we always shadow variables?
19:57 - Can we use polymorphic functions?
20:01 - Purposely Thrashing the Borrow Checker
23:45 - Accept the Borrow Checker warnings
25:15 - Outro
_ Source Material _
The Rust Programming Language by Steve Klabnik and Carol Nichols
______ Links ______
Комментарии