filmov
tv
Can Place Flowers - Leetcode 605 - Python
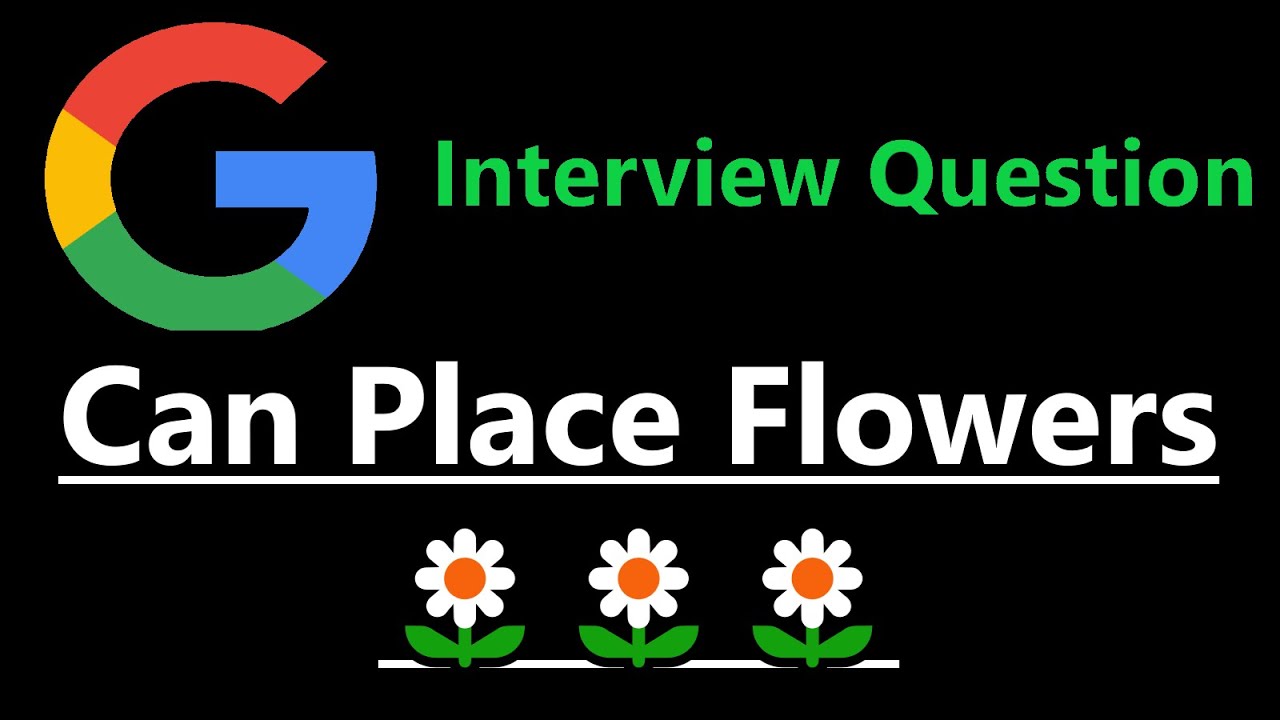
Показать описание
0:00 - Read the problem
1:37 - Drawing Explanation
6:27 - Coding Explanation
leetcode 605
#google #interview #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Can Place Flowers - Leetcode 605 - Python
Can Place Flowers | Live Coding with Explanation | Leetcode - 605
605. Can Place Flowers | LeetCode 75 #4 | Array / String
Leetcode 605. Can Place Flowers [Java]
Can Place Flowers - (Leetcode - 605) - (GOOGLE) : Explanation ➕ Live Coding
Can Place Flowers - Leetcode 605 - Python
Leetcode 605 | Can place flowers | 4th from LC 75 | 90 days SE prep
CAN PLACE FLOWERS LEETCODE
Can Place Flowers🔥 | Leetcode 605 | Greedy
Leetcode | 605. Can Place Flowers | Easy | Java Solution
605. Can Place Flowers - LeetCode Java Solution
605. Can Place Flowers ( Leetcode Problem)
Solving the 'Can Place Flowers' Problem in Java | LeetCode 75 Study Plan
605. Can Place Flowers | JavaScript | Array | LeetCode Daily Challenge
Can Place Flowers | Leetcode 605 | Live coding session 🔥🔥🔥🔥| Easy Peasy O(n)
Can Place Flowers - LeetCode 605 - JavaScript
Can Place Flowers 💐 | can place flowers 💐 | leetcode 605 | array
Can Place Flowers - Leetcode 605 - Python
LeetCode 605. Can Place Flowers C++ Code Simple Solution
Can Place Flowers - LeetCode 605 - Python #leetcode #leetcode75
(Remade) Leetcode 605 - Can Place Flowers | Array
LeetCode 605 - Can Place Flowers [Daily Challenge]
LeetCode 605 - Can Place Flowers | LeetCode 75 | Davi Codes - JavaScript
Can Place Flowers | Leetcode | Arrays | Programming in Tamil | data structures | coding | c++ | DSA
Комментарии