filmov
tv
Debugging Multi-threaded Applications with System.Diagnostics in C#
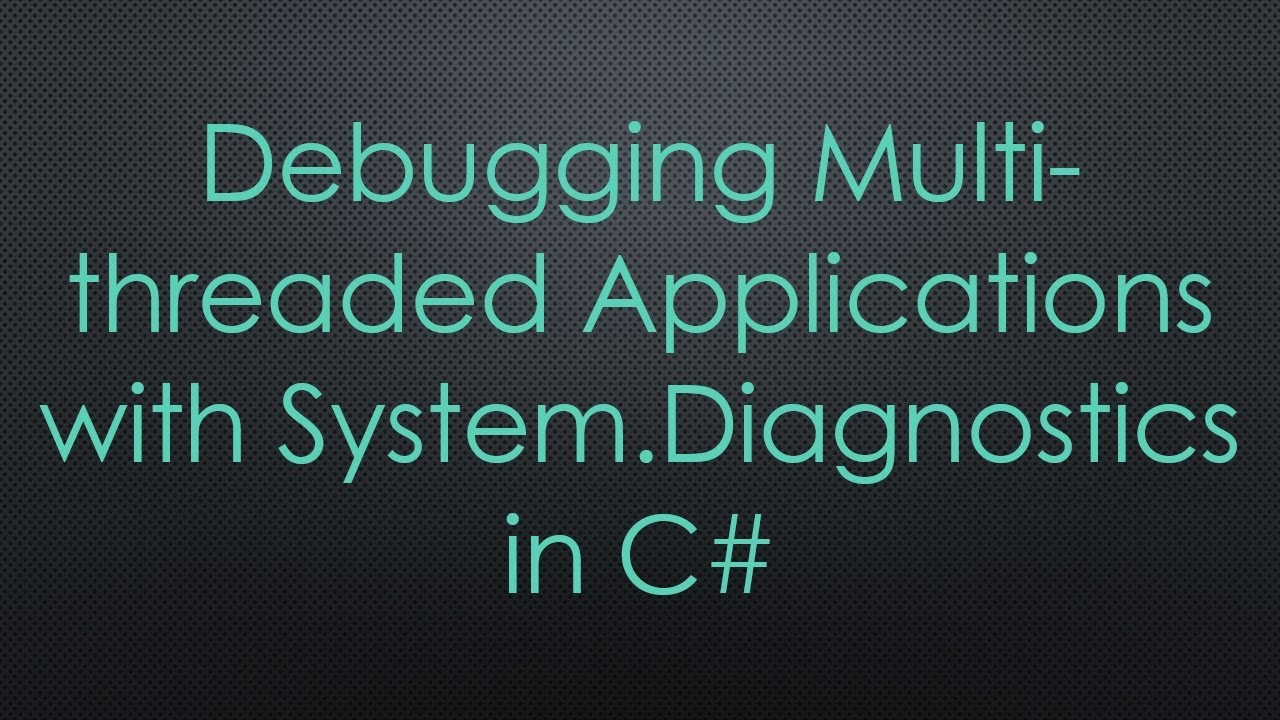
Показать описание
Learn how to effectively debug multi-threaded applications in C# using the System.Diagnostics namespace for enhanced monitoring and troubleshooting. Explore techniques to identify and resolve common issues encountered in concurrent programming.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Multi-threaded programming in C can significantly enhance the performance and responsiveness of applications. However, it also introduces complexities and challenges, particularly in debugging and troubleshooting. Bugs in multi-threaded applications can be elusive and difficult to reproduce, making them notoriously tricky to resolve. Thankfully, the System.Diagnostics namespace in C provides powerful tools and techniques for debugging multi-threaded applications effectively.
Using System.Diagnostics for Debugging
The System.Diagnostics namespace in C offers a range of classes and tools to aid in debugging and performance monitoring. One of the key components for debugging multi-threaded applications is the Debugger class, which allows developers to inspect and manipulate threads during runtime.
Breakpoints and Stepping Through Code
Setting breakpoints in Visual Studio or using the System.Diagnostics.Debugger.Break() method allows you to pause the execution of your multi-threaded application at specific points in the code. This enables you to inspect variables, thread states, and identify potential issues.
When debugging multi-threaded applications, it's crucial to step through code sequentially to understand the flow of execution across different threads. Visual Studio provides features like stepping into, stepping over, and stepping out of code segments, which are invaluable for tracing the behavior of concurrent threads.
Thread Monitoring and Control
The System.Diagnostics namespace offers various properties and methods to monitor and control threads during debugging. For example, the Thread.CurrentThread property allows you to access the currently executing thread, while the Thread.Name property enables you to assign descriptive names to threads for easier identification in debugging sessions.
Additionally, the Thread.Sleep() method can be used strategically to introduce delays in thread execution, facilitating the observation of race conditions and synchronization issues.
Debugging Synchronization Issues
Debugging multi-threaded applications often involves tackling synchronization issues such as race conditions, deadlocks, and thread contention. The System.Diagnostics namespace provides tools to diagnose and address these challenges effectively.
By analyzing thread states, lock acquisitions, and resource access patterns, developers can pinpoint areas of contention and implement appropriate synchronization mechanisms such as locks, mutexes, or semaphores to ensure thread safety.
Logging and Tracing
In addition to traditional debugging techniques, logging and tracing are invaluable tools for diagnosing issues in multi-threaded applications. The System.Diagnostics namespace offers classes like Trace and Debug for emitting diagnostic messages and tracing information to log files or the console.
By strategically instrumenting your code with logging statements, you can capture valuable insights into the behavior of concurrent threads, helping to identify patterns of execution and diagnose elusive bugs.
Conclusion
Debugging multi-threaded applications in C requires a systematic approach and the effective use of tools provided by the System.Diagnostics namespace. By leveraging breakpoints, thread monitoring, synchronization analysis, and logging techniques, developers can identify and resolve issues in concurrent programs more efficiently.
While debugging multi-threaded applications can be challenging, mastering the tools and techniques offered by System.Diagnostics empowers developers to build robust and reliable concurrent software.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Multi-threaded programming in C can significantly enhance the performance and responsiveness of applications. However, it also introduces complexities and challenges, particularly in debugging and troubleshooting. Bugs in multi-threaded applications can be elusive and difficult to reproduce, making them notoriously tricky to resolve. Thankfully, the System.Diagnostics namespace in C provides powerful tools and techniques for debugging multi-threaded applications effectively.
Using System.Diagnostics for Debugging
The System.Diagnostics namespace in C offers a range of classes and tools to aid in debugging and performance monitoring. One of the key components for debugging multi-threaded applications is the Debugger class, which allows developers to inspect and manipulate threads during runtime.
Breakpoints and Stepping Through Code
Setting breakpoints in Visual Studio or using the System.Diagnostics.Debugger.Break() method allows you to pause the execution of your multi-threaded application at specific points in the code. This enables you to inspect variables, thread states, and identify potential issues.
When debugging multi-threaded applications, it's crucial to step through code sequentially to understand the flow of execution across different threads. Visual Studio provides features like stepping into, stepping over, and stepping out of code segments, which are invaluable for tracing the behavior of concurrent threads.
Thread Monitoring and Control
The System.Diagnostics namespace offers various properties and methods to monitor and control threads during debugging. For example, the Thread.CurrentThread property allows you to access the currently executing thread, while the Thread.Name property enables you to assign descriptive names to threads for easier identification in debugging sessions.
Additionally, the Thread.Sleep() method can be used strategically to introduce delays in thread execution, facilitating the observation of race conditions and synchronization issues.
Debugging Synchronization Issues
Debugging multi-threaded applications often involves tackling synchronization issues such as race conditions, deadlocks, and thread contention. The System.Diagnostics namespace provides tools to diagnose and address these challenges effectively.
By analyzing thread states, lock acquisitions, and resource access patterns, developers can pinpoint areas of contention and implement appropriate synchronization mechanisms such as locks, mutexes, or semaphores to ensure thread safety.
Logging and Tracing
In addition to traditional debugging techniques, logging and tracing are invaluable tools for diagnosing issues in multi-threaded applications. The System.Diagnostics namespace offers classes like Trace and Debug for emitting diagnostic messages and tracing information to log files or the console.
By strategically instrumenting your code with logging statements, you can capture valuable insights into the behavior of concurrent threads, helping to identify patterns of execution and diagnose elusive bugs.
Conclusion
Debugging multi-threaded applications in C requires a systematic approach and the effective use of tools provided by the System.Diagnostics namespace. By leveraging breakpoints, thread monitoring, synchronization analysis, and logging techniques, developers can identify and resolve issues in concurrent programs more efficiently.
While debugging multi-threaded applications can be challenging, mastering the tools and techniques offered by System.Diagnostics empowers developers to build robust and reliable concurrent software.