filmov
tv
How to Create an SQLite Database Once on Startup in React Native
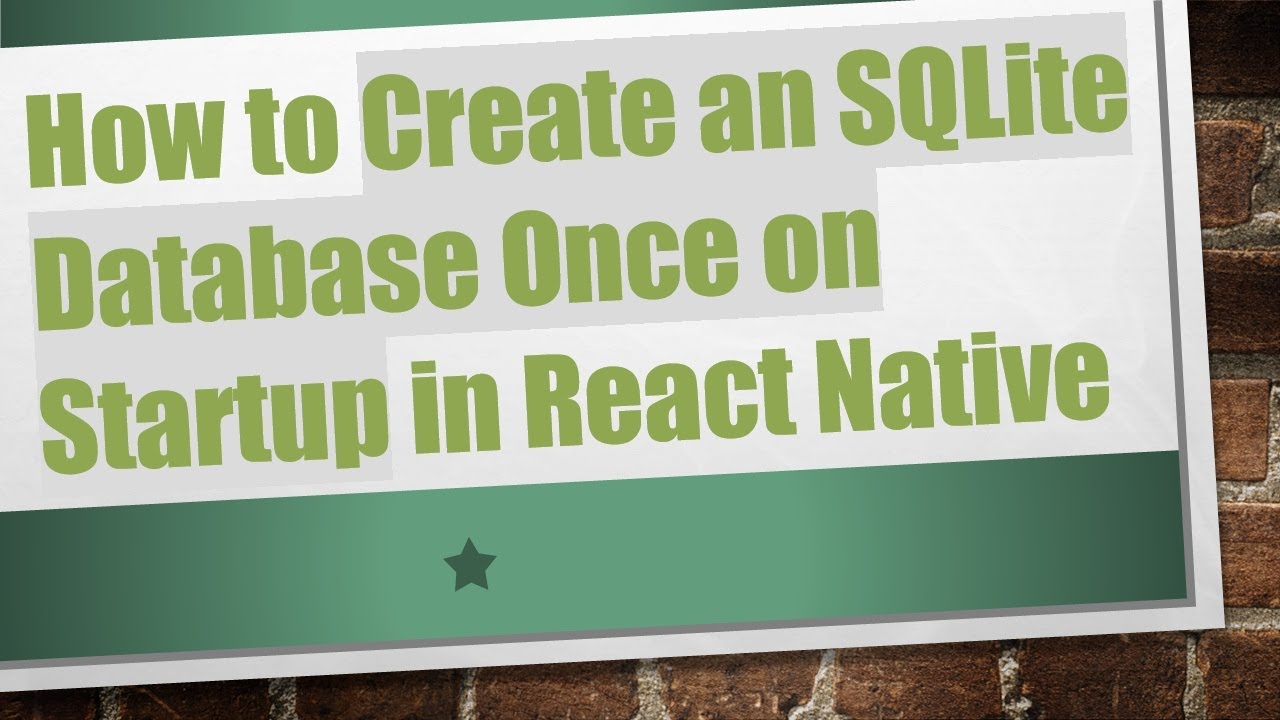
Показать описание
Learn how to create an SQLite database for your React Native application on startup. This guide walks you through avoiding overwriting tables and keeping your records intact.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Create sqlite database once on startup
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Create an SQLite Database Once on Startup in React Native
Setting up your first database in a React Native application can be challenging, especially when you want to ensure that your database tables are created once at startup and do not get overwritten. If you've ever faced the frustration of losing your data every time your application restarts, you're not alone! In this guide, we'll delve into the best practices for managing your SQLite database in React Native effectively.
The Problem
Imagine you are building an application for a hackathon and have just integrated an SQLite database. You want to create table structures when the landing page is opened, but every time you restart your app, the tables are recreated, wiping out all user data. This can be particularly frustrating when you want users to be able to sign up and log in without losing existing records.
Understanding the Logic Behind the SQLite Setup
The crucial component here is ensuring that your table creation logic accommodates the state of existing tables. In your case, you're utilizing SQL commands to check the existence of tables, but there's a mistake in your logic.
The Issue with Your Query
The current code that checks for the existence of both tables is as follows:
[[See Video to Reveal this Text or Code Snippet]]
The problem is that this query will never return results as it checks for name='user' AND name='data' in one row, which is impossible.
The Correction You Need
To correct this, you should modify your SQL statement to check for either table's existence using the OR operator:
[[See Video to Reveal this Text or Code Snippet]]
With this adjustment, you'll be able to determine if either of the tables exists successfully.
Simplifying Your Table Creation Logic
After fixing the query, you'll realize that you might not even need this check. The most straightforward way to handle table creation in SQLite is by using CREATE TABLE IF NOT EXISTS.... This command creates a table only if it doesn’t already exist, eliminating the need for your checks entirely.
Here's what you should implement:
Remove the Existence Query: You can skip the initial check completely.
Create Tables as Needed:
[[See Video to Reveal this Text or Code Snippet]]
Final Code Snippet
Here’s how the implementation will look in your Welcome component:
[[See Video to Reveal this Text or Code Snippet]]
Summary
By understanding the oversight in the query logic and simplifying the table creation, you not only save yourself from unnecessary complexity but also ensure that user records remain intact throughout the app sessions. Always remember to verify if there’s any additional code that might unintentionally delete your database files upon app restarts.
Now you are well-equipped to integrate SQLite smoothly into your React Native application without any data loss. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Create sqlite database once on startup
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Create an SQLite Database Once on Startup in React Native
Setting up your first database in a React Native application can be challenging, especially when you want to ensure that your database tables are created once at startup and do not get overwritten. If you've ever faced the frustration of losing your data every time your application restarts, you're not alone! In this guide, we'll delve into the best practices for managing your SQLite database in React Native effectively.
The Problem
Imagine you are building an application for a hackathon and have just integrated an SQLite database. You want to create table structures when the landing page is opened, but every time you restart your app, the tables are recreated, wiping out all user data. This can be particularly frustrating when you want users to be able to sign up and log in without losing existing records.
Understanding the Logic Behind the SQLite Setup
The crucial component here is ensuring that your table creation logic accommodates the state of existing tables. In your case, you're utilizing SQL commands to check the existence of tables, but there's a mistake in your logic.
The Issue with Your Query
The current code that checks for the existence of both tables is as follows:
[[See Video to Reveal this Text or Code Snippet]]
The problem is that this query will never return results as it checks for name='user' AND name='data' in one row, which is impossible.
The Correction You Need
To correct this, you should modify your SQL statement to check for either table's existence using the OR operator:
[[See Video to Reveal this Text or Code Snippet]]
With this adjustment, you'll be able to determine if either of the tables exists successfully.
Simplifying Your Table Creation Logic
After fixing the query, you'll realize that you might not even need this check. The most straightforward way to handle table creation in SQLite is by using CREATE TABLE IF NOT EXISTS.... This command creates a table only if it doesn’t already exist, eliminating the need for your checks entirely.
Here's what you should implement:
Remove the Existence Query: You can skip the initial check completely.
Create Tables as Needed:
[[See Video to Reveal this Text or Code Snippet]]
Final Code Snippet
Here’s how the implementation will look in your Welcome component:
[[See Video to Reveal this Text or Code Snippet]]
Summary
By understanding the oversight in the query logic and simplifying the table creation, you not only save yourself from unnecessary complexity but also ensure that user records remain intact throughout the app sessions. Always remember to verify if there’s any additional code that might unintentionally delete your database files upon app restarts.
Now you are well-equipped to integrate SQLite smoothly into your React Native application without any data loss. Happy coding!