filmov
tv
Java serialization 🥣
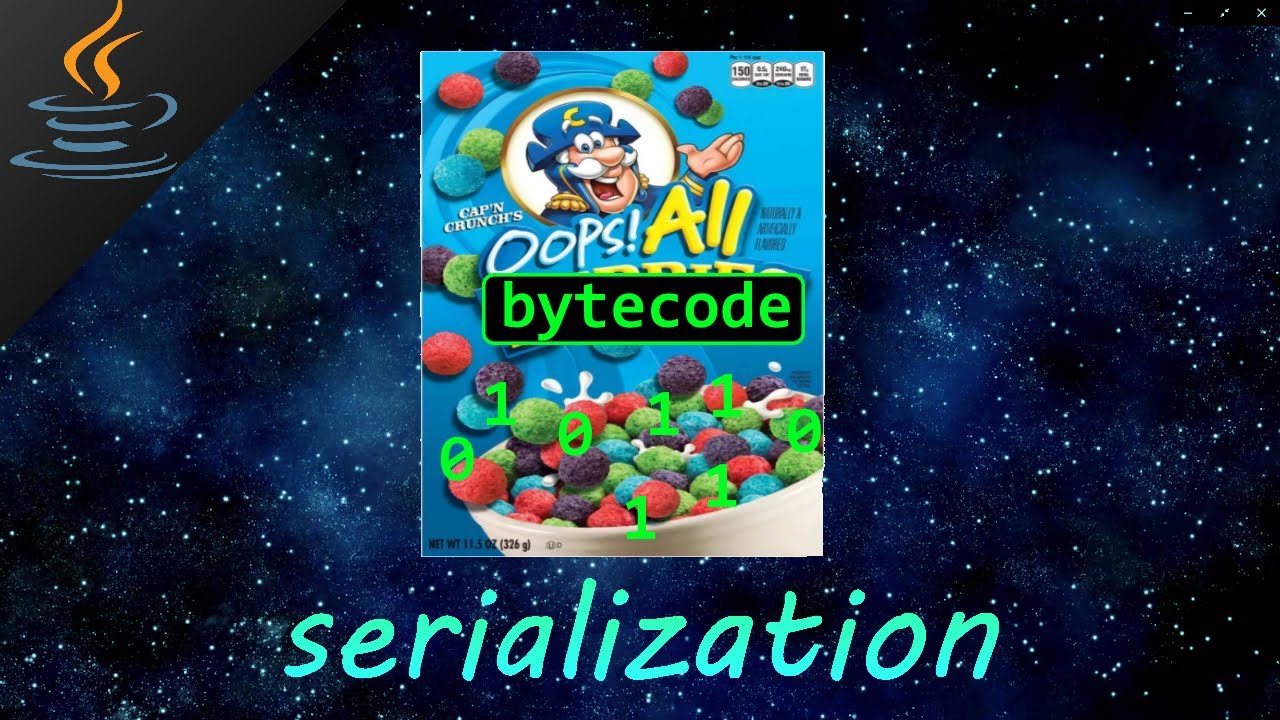
Показать описание
Java serialization tutorial for beginners
#Java #serialization #tutorial
00:19 serialize
08:47 deserialize
13:40 advanced stuff
Coding boot camps hate him! See how he can teach you to code with this one simple trick...
Bro Code is the self-proclaimed #1 tutorial series on coding in various programming languages and other how-to videos in the known universe.
#Java #serialization #tutorial
00:19 serialize
08:47 deserialize
13:40 advanced stuff
Coding boot camps hate him! See how he can teach you to code with this one simple trick...
Bro Code is the self-proclaimed #1 tutorial series on coding in various programming languages and other how-to videos in the known universe.
Java serialization 🥣
Java - Serialization & Deserialization
12.3 Object Serialization in java | Serializable Interface
Serialization Explained in 3 minutes | Tech Primers
Object Serialization in Java | Serialization Interface | Java Tutorial | Edureka
Serialization - A New Hope
Java Serialization was a Horrible Mistake
Why We Hate Java Serialization And What We're Doing About It by Brian Goetz & Stuart Marks
Java - Serialization
What is Serialization? - Cracking the Java Coding Interview
Java Serialisierung von Objekten | Java Tutorial für Fortgeschrittene
Serialization - A Crash Course
Сериализация с примером на пальцах - JAVA #java #serialization #deserialization...
When Java Records Met Serialization: A Happy Tale
Serialization Deserialization & Externalization | Java Interview Questions and Answer | Code Dec...
Java Fundamentals - Lesson 61 - Serialization
Java Tutorial For Beginners | Serialization In Java | Java Serialization Tutorial | SimpliCode
Java File Input/Output - It's Way Easier Than You Think
Java Interview Shorts 23 - what is the need of serialization in java ? #javainterviewquestions
Object Serialization In Java - Easy explanation
Serialization and De-Serialization in Java | Pradeep Nailwal
Java Serialization
43- OOP|| Serialization in Java
#076 [JAVA] - File Handling (Serialization and Deserialization ,Read & Write multiple objects)
Комментарии