filmov
tv
How to Calculate Sum of Multiple Input Values in JavaScript with Thymeleaf
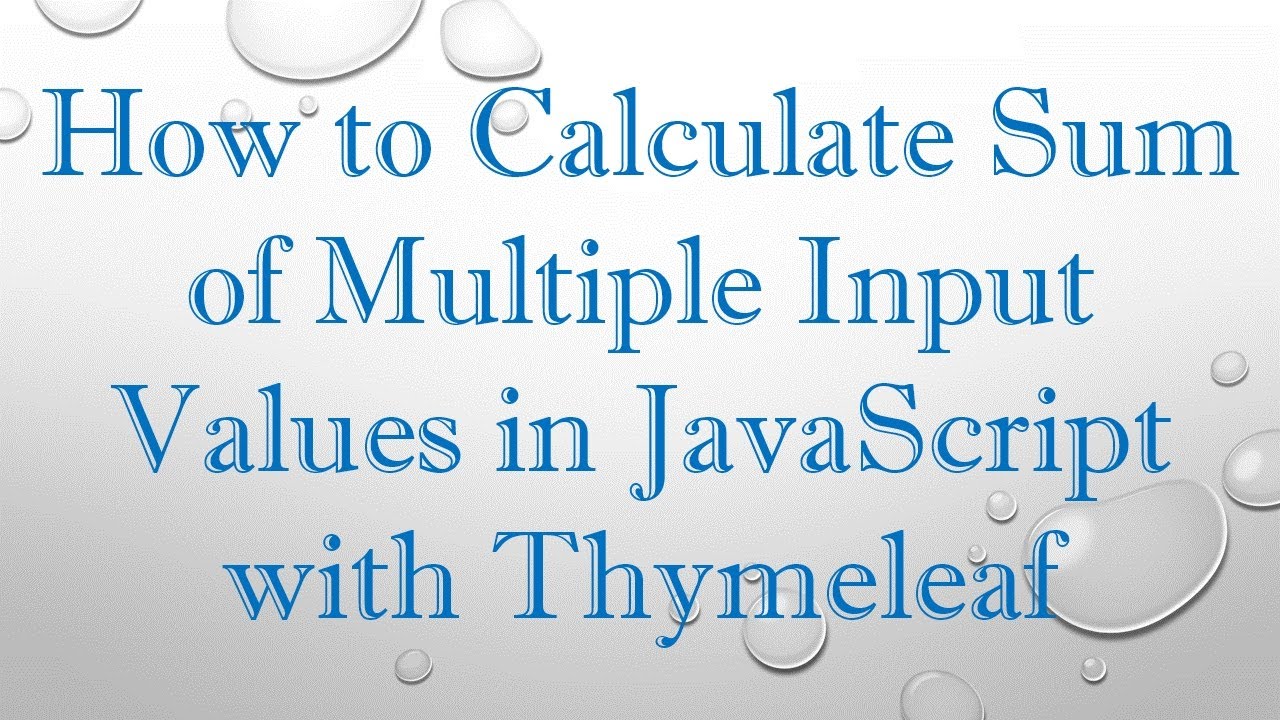
Показать описание
Discover how to efficiently sum values from multiple input fields using JavaScript and Thymeleaf. Learn to avoid common pitfalls like using duplicate IDs and how to implement effective class selectors.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Can't get values of input with js
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
A Common JavaScript Challenge: Summing Values from Input Fields
If you’re working with dynamic forms in JavaScript, particularly with frameworks like Thymeleaf, you might face challenges when trying to sum values from multiple input fields. A common issue that arises is not being able to retrieve the values correctly for all iterations of a loop. Instead, only the first value is returned, leading to confusion and frustrating debugging sessions. In this post, we will explore why this happens and how to effectively solve it.
Understanding the Problem
In your code, you're iterating through a list of persons and rendering an input field for each individual's age. The goal is to capture the values from all these age inputs and calculate the total when any of the fields change. Here's a recap of your setup:
[[See Video to Reveal this Text or Code Snippet]]
Your JavaScript script attempts to calculate the sum by using the ID selector for the age input.
[[See Video to Reveal this Text or Code Snippet]]
Why It Doesn't Work
The key issue in your code is the use of id for the age input fields. According to HTML specifications, IDs must be unique within a page. Consequently, the jQuery $("# age") selector only returns the first element it finds with that ID. This results in calculations that only consider the input field's value associated with the first iteration. When you hover or interact with any other fields, they remain ignored.
The Solution: Use Classes Instead
To fix this issue, we can replace the id with a class for the age inputs. By doing this, we conform to the HTML standards and allow for multiple elements to share the same class without conflicts.
Step-by-Step Fix
Change the Input Field: Update the input tag to use a class selector instead of an id.
[[See Video to Reveal this Text or Code Snippet]]
Modify the JavaScript: Update your JavaScript to select elements by class name.
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
jQuery Selector: By selecting with $(".age"), you capture all elements with that class.
Event Listener: The event listener reacts to any input in any of the fields with that class.
Sum Calculation: The calculateSum() function loops through all .age elements and aggregates their values to produce a total, which is then displayed in the # sum input field.
Conclusion
When developing dynamic forms with JavaScript, paying attention to the proper use of IDs and classes is crucial. By switching from ID selectors to class selectors, you can ensure that all relevant input fields are considered in your calculations. Remember, the key takeaway here is: Use unique IDs and prefer classes when dealing with multiple elements. This approach will prevent similar issues in the future and make your code more robust and maintainable.
With these changes, you should now be able to get the desired sum from all input fields as users interact with them. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Can't get values of input with js
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
A Common JavaScript Challenge: Summing Values from Input Fields
If you’re working with dynamic forms in JavaScript, particularly with frameworks like Thymeleaf, you might face challenges when trying to sum values from multiple input fields. A common issue that arises is not being able to retrieve the values correctly for all iterations of a loop. Instead, only the first value is returned, leading to confusion and frustrating debugging sessions. In this post, we will explore why this happens and how to effectively solve it.
Understanding the Problem
In your code, you're iterating through a list of persons and rendering an input field for each individual's age. The goal is to capture the values from all these age inputs and calculate the total when any of the fields change. Here's a recap of your setup:
[[See Video to Reveal this Text or Code Snippet]]
Your JavaScript script attempts to calculate the sum by using the ID selector for the age input.
[[See Video to Reveal this Text or Code Snippet]]
Why It Doesn't Work
The key issue in your code is the use of id for the age input fields. According to HTML specifications, IDs must be unique within a page. Consequently, the jQuery $("# age") selector only returns the first element it finds with that ID. This results in calculations that only consider the input field's value associated with the first iteration. When you hover or interact with any other fields, they remain ignored.
The Solution: Use Classes Instead
To fix this issue, we can replace the id with a class for the age inputs. By doing this, we conform to the HTML standards and allow for multiple elements to share the same class without conflicts.
Step-by-Step Fix
Change the Input Field: Update the input tag to use a class selector instead of an id.
[[See Video to Reveal this Text or Code Snippet]]
Modify the JavaScript: Update your JavaScript to select elements by class name.
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
jQuery Selector: By selecting with $(".age"), you capture all elements with that class.
Event Listener: The event listener reacts to any input in any of the fields with that class.
Sum Calculation: The calculateSum() function loops through all .age elements and aggregates their values to produce a total, which is then displayed in the # sum input field.
Conclusion
When developing dynamic forms with JavaScript, paying attention to the proper use of IDs and classes is crucial. By switching from ID selectors to class selectors, you can ensure that all relevant input fields are considered in your calculations. Remember, the key takeaway here is: Use unique IDs and prefer classes when dealing with multiple elements. This approach will prevent similar issues in the future and make your code more robust and maintainable.
With these changes, you should now be able to get the desired sum from all input fields as users interact with them. Happy coding!