filmov
tv
Understanding Python argparse Command Line Flags Without Arguments
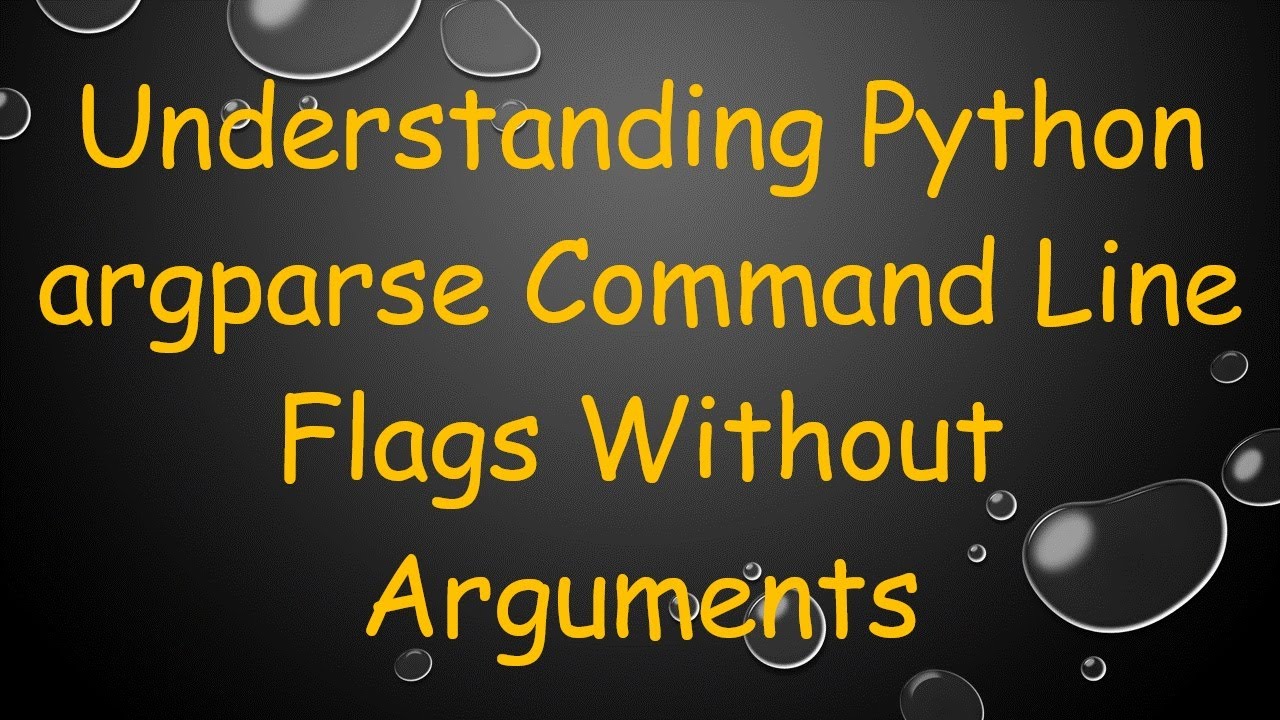
Показать описание
Learn how to effectively use Python's argparse module to handle command line flags without arguments, enhancing your command-line interface applications.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Understanding Python argparse Command Line Flags Without Arguments
Python's argparse module is a powerful tool for creating command-line interfaces. It simplifies the parsing of command-line arguments, making it easier to handle user inputs. One common requirement is handling command line flags that do not take any arguments. These flags, often referred to as "boolean flags" or "switches," can be used to toggle certain behaviors on or off.
What Are Command Line Flags Without Arguments?
Command line flags without arguments are used to indicate a binary choice: true or false, on or off, enabled or disabled. When a flag is present in the command line input, it typically signifies True, and its absence signifies False.
For example, in a script that processes files, you might want to include a flag like --verbose to indicate whether to run in verbose mode. If the user includes --verbose in the command line, verbose mode is enabled; otherwise, it is not.
Using argparse to Handle Flags
To define a command line flag using argparse, you use the add_argument method with the action parameter set to 'store_true' or 'store_false'. Here's how it works:
Step-by-Step Guide
Import the argparse module:
[[See Video to Reveal this Text or Code Snippet]]
Create an ArgumentParser object:
[[See Video to Reveal this Text or Code Snippet]]
Add a flag:
[[See Video to Reveal this Text or Code Snippet]]
Parse the arguments:
[[See Video to Reveal this Text or Code Snippet]]
Use the flag in your code:
[[See Video to Reveal this Text or Code Snippet]]
Complete Example
Here's a complete example demonstrating how to use argparse to handle a --verbose flag:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
action='store_true': This tells argparse to store True if the flag is present and False if it is not.
help='increase output verbosity': This provides a description for the flag, which is displayed when the user runs the script with the -h or --help option.
Other Actions
While store_true is commonly used for flags without arguments, argparse provides several other actions that might be useful:
store_false: Stores False if the flag is present and True if it is not.
count: Counts the number of times a flag appears. Useful for flags that can be repeated to increase a count (e.g., -vv for verbosity level).
Conclusion
Using argparse to handle command line flags without arguments is straightforward and enhances the functionality of your command-line interfaces. By defining flags like --verbose, you can easily control the behavior of your script based on user inputs. Whether you're building simple scripts or complex applications, understanding how to implement these flags will make your tools more flexible and user-friendly.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Understanding Python argparse Command Line Flags Without Arguments
Python's argparse module is a powerful tool for creating command-line interfaces. It simplifies the parsing of command-line arguments, making it easier to handle user inputs. One common requirement is handling command line flags that do not take any arguments. These flags, often referred to as "boolean flags" or "switches," can be used to toggle certain behaviors on or off.
What Are Command Line Flags Without Arguments?
Command line flags without arguments are used to indicate a binary choice: true or false, on or off, enabled or disabled. When a flag is present in the command line input, it typically signifies True, and its absence signifies False.
For example, in a script that processes files, you might want to include a flag like --verbose to indicate whether to run in verbose mode. If the user includes --verbose in the command line, verbose mode is enabled; otherwise, it is not.
Using argparse to Handle Flags
To define a command line flag using argparse, you use the add_argument method with the action parameter set to 'store_true' or 'store_false'. Here's how it works:
Step-by-Step Guide
Import the argparse module:
[[See Video to Reveal this Text or Code Snippet]]
Create an ArgumentParser object:
[[See Video to Reveal this Text or Code Snippet]]
Add a flag:
[[See Video to Reveal this Text or Code Snippet]]
Parse the arguments:
[[See Video to Reveal this Text or Code Snippet]]
Use the flag in your code:
[[See Video to Reveal this Text or Code Snippet]]
Complete Example
Here's a complete example demonstrating how to use argparse to handle a --verbose flag:
[[See Video to Reveal this Text or Code Snippet]]
Explanation
action='store_true': This tells argparse to store True if the flag is present and False if it is not.
help='increase output verbosity': This provides a description for the flag, which is displayed when the user runs the script with the -h or --help option.
Other Actions
While store_true is commonly used for flags without arguments, argparse provides several other actions that might be useful:
store_false: Stores False if the flag is present and True if it is not.
count: Counts the number of times a flag appears. Useful for flags that can be repeated to increase a count (e.g., -vv for verbosity level).
Conclusion
Using argparse to handle command line flags without arguments is straightforward and enhances the functionality of your command-line interfaces. By defining flags like --verbose, you can easily control the behavior of your script based on user inputs. Whether you're building simple scripts or complex applications, understanding how to implement these flags will make your tools more flexible and user-friendly.