filmov
tv
How to Efficiently Convert an Array of Objects to an Object in JavaScript
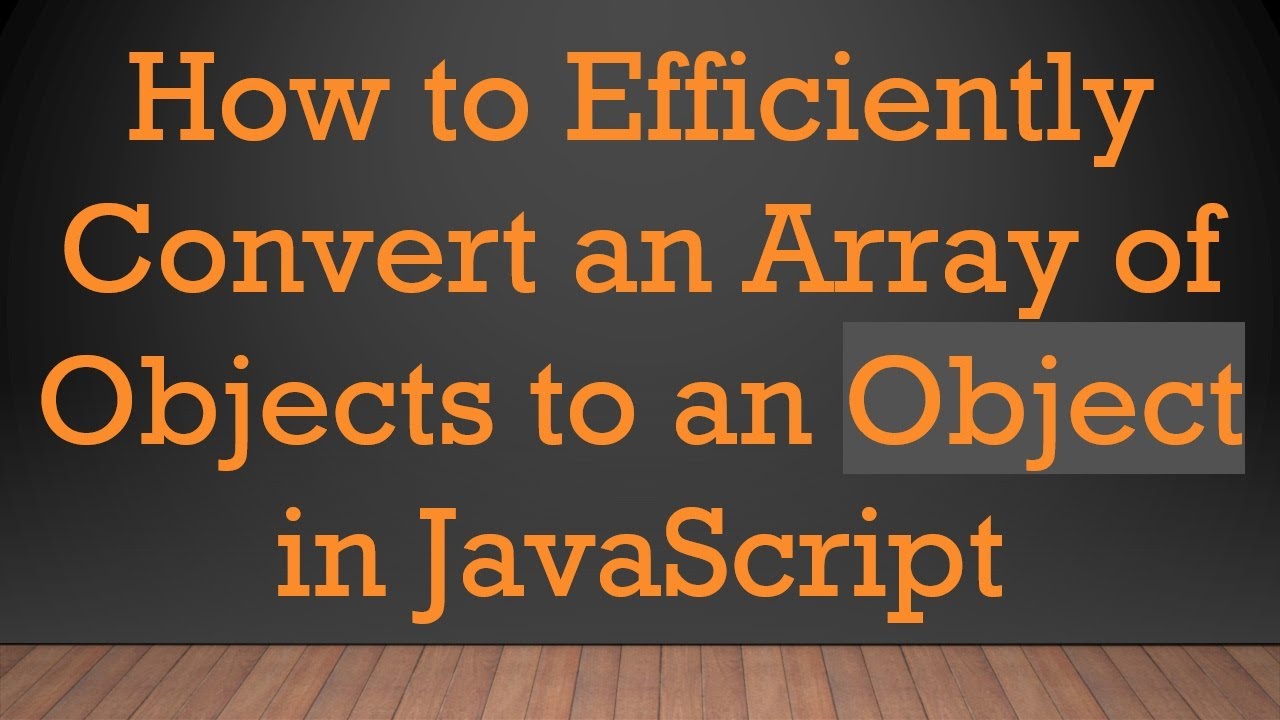
Показать описание
Struggling to convert an array of objects into a single JavaScript object? This guide provides a clear step-by-step approach with code examples to help you achieve this transformation seamlessly!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to convert an array of objects to object
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Efficiently Convert an Array of Objects to an Object in JavaScript
If you're working with JavaScript, chances are you've encountered arrays of objects and needed to transform them into a single object. This can be a common scenario, especially when handling data structures in applications. In this post, we'll explore an example and provide a clear, step-by-step guide on how to achieve this transformation.
Understanding the Problem
Let's say you have an array that looks like this:
[[See Video to Reveal this Text or Code Snippet]]
And you want to convert it to a single object like this:
[[See Video to Reveal this Text or Code Snippet]]
This transformation allows you to access the nested objects more easily, but how can we accomplish this with JavaScript?
The Solution
To solve this problem, we can use the reduce method available on arrays. This method helps us transform the array into an object by accumulating the results. Let’s break down the solution step-by-step.
Step 1: Utilize the reduce Method
The reduce method iterates over each element of an array and reduces it to a single output value — in this case, an object.
Step 2: Create the Accumulator Function
We will create an accumulator function that takes two parameters:
acc: The accumulator, which holds our resulting object.
x: The current element being processed in the array.
Step 3: Merging Objects
In the callback function provided to reduce, we can merge the accumulator with the current object x. We achieve this using the spread operator (...).
Step 4: Complete Code Example
Here’s how you can implement the solution in JavaScript:
[[See Video to Reveal this Text or Code Snippet]]
How This Works
Initialization: The reduce method starts with the first element of the array.
Spread Operator: The ... operator allows us to merge the properties of the current object (x) into our accumulator (acc).
Final Output: After completing the iteration, reduce returns a single object combining all the elements.
Why Use This Approach?
Using reduce with the spread operator offers several advantages:
Conciseness: The solution is compact and easy to read.
Efficiency: This method processes the array in a single pass.
Maintainability: Keeping the code clear enhances maintainability and extendibility for future adjustments.
Conclusion
Transforming an array of objects into a single object in JavaScript can be efficiently achieved using the reduce method along with the spread operator. By following the approach outlined above, you can handle data transformations with ease and clarity.
The next time you find yourself in need of this solution, remember to leverage these techniques for a smooth coding experience!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to convert an array of objects to object
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Efficiently Convert an Array of Objects to an Object in JavaScript
If you're working with JavaScript, chances are you've encountered arrays of objects and needed to transform them into a single object. This can be a common scenario, especially when handling data structures in applications. In this post, we'll explore an example and provide a clear, step-by-step guide on how to achieve this transformation.
Understanding the Problem
Let's say you have an array that looks like this:
[[See Video to Reveal this Text or Code Snippet]]
And you want to convert it to a single object like this:
[[See Video to Reveal this Text or Code Snippet]]
This transformation allows you to access the nested objects more easily, but how can we accomplish this with JavaScript?
The Solution
To solve this problem, we can use the reduce method available on arrays. This method helps us transform the array into an object by accumulating the results. Let’s break down the solution step-by-step.
Step 1: Utilize the reduce Method
The reduce method iterates over each element of an array and reduces it to a single output value — in this case, an object.
Step 2: Create the Accumulator Function
We will create an accumulator function that takes two parameters:
acc: The accumulator, which holds our resulting object.
x: The current element being processed in the array.
Step 3: Merging Objects
In the callback function provided to reduce, we can merge the accumulator with the current object x. We achieve this using the spread operator (...).
Step 4: Complete Code Example
Here’s how you can implement the solution in JavaScript:
[[See Video to Reveal this Text or Code Snippet]]
How This Works
Initialization: The reduce method starts with the first element of the array.
Spread Operator: The ... operator allows us to merge the properties of the current object (x) into our accumulator (acc).
Final Output: After completing the iteration, reduce returns a single object combining all the elements.
Why Use This Approach?
Using reduce with the spread operator offers several advantages:
Conciseness: The solution is compact and easy to read.
Efficiency: This method processes the array in a single pass.
Maintainability: Keeping the code clear enhances maintainability and extendibility for future adjustments.
Conclusion
Transforming an array of objects into a single object in JavaScript can be efficiently achieved using the reduce method along with the spread operator. By following the approach outlined above, you can handle data transformations with ease and clarity.
The next time you find yourself in need of this solution, remember to leverage these techniques for a smooth coding experience!