filmov
tv
React Hooks Project Tutorial - Game of Life
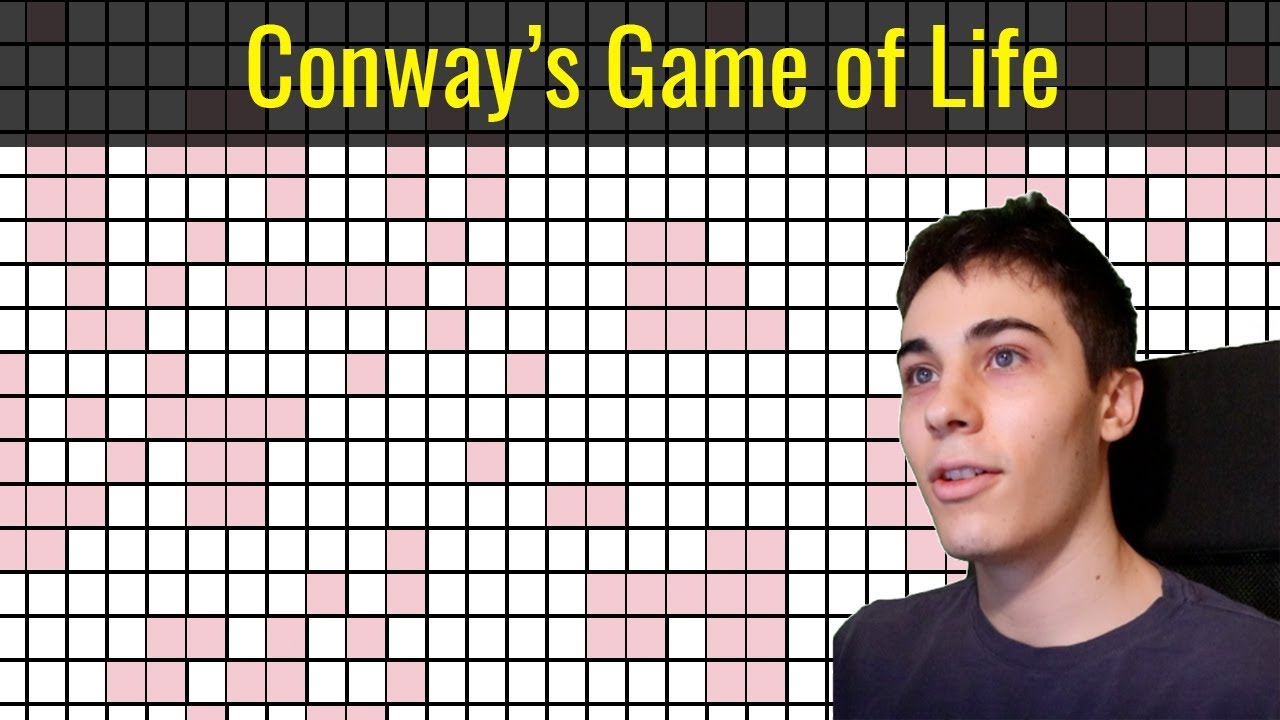
Показать описание
Learn how to build Conway's Game of Life using React hooks in this project tutorial.
----
#benawad
----
#benawad
10 React Hooks Explained // Plus Build your own from Scratch
Learn useState In 15 Minutes - React Hooks Explained
React Hooks Course - All React Hooks Explained
Learn React With This One Project
All React Hooks Explained in 2 Hours | Complete React Hooks Tutorial with Example 2024
Learn React Hooks: useEffect - Simply Explained!
Learn React Hooks: useRef - Simply Explained!
10 React Antipatterns to Avoid - Code This, Not That!
2 Intro to React Hooks and Components
React Hooks Project Tutorial - Game of Life
Full React Tutorial #14 - useEffect Hook (the basics)
React Hooks in ONE Video 2022 [ EASIEST Explanation ] | React JS Tutorial
5 Custom React Hooks You Need In Every Project
Learn React Hooks: useCallback - Simply Explained!
React Hooks Tutorial | React Hooks Explained | React Hooks Project 2022 | React Tutorial|Simplilearn
Learn React Hooks: useState - Simply Explained!
Top 5 React JS projects for Beginners
React Hooks Tutorial
Full React Tutorial #20 - Making a Custom Hook
Learn React Hooks: useMemo - Simply Explained!
Full React Tutorial #8 - Using State (useState hook)
React useMemo Hook in 1 Minute | React Hooks Series - Part 2
useContext In 2 Minutes - the React Hooks series
Why you need hooks and project
Комментарии