filmov
tv
Solve Your useEffect Problem in React
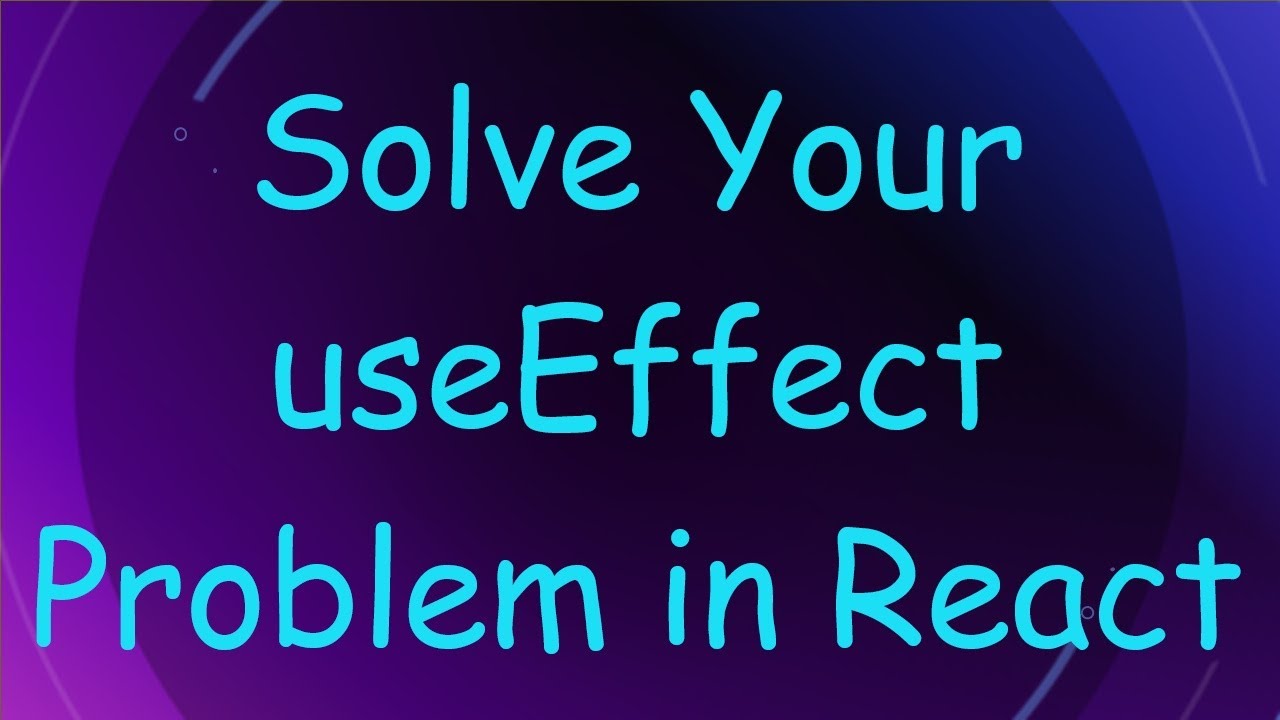
Показать описание
Learn how to resolve issues with React's `useEffect` and `useState` hooks. This guide provides practical insights to help you manage asynchronous data fetching effectively.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: useEffect is not called unless a not related useState is set to true
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the useEffect Issue in React
If you're new to React, you might find yourself confronting a puzzling problem: Why is the useEffect not being called unless a disconnected useState is triggered? Many developers, especially beginners, encounter this scenario while trying to fetch data from an API. Let’s break down the issue and explore a solution that can help streamline your data fetching in React.
The Problem with useEffect
You have a React component that tries to fetch a list of wines from an API when the component mounts. The code looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
In this code, you noticed that useEffect never gets triggered until you force a state change by setting the initial isDataLoading state to true. Why does this happen? Let's look at the solution.
Solving the useEffect Issue
Step 1: Move Your Async Function Outside of useEffect
One common mistake is nesting the asynchronous function within the useEffect. Instead, you can define the fetchWine function outside of it. This slight restructuring lets useEffect focus solely on executing the function on mount:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Call the Function Inside useEffect
Now, keep useEffect focused on calling this function:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Set Loading State Effectively
You also want to manage the loading state correctly. Set isDataLoading to true only when you start fetching the data, and set it back to false once the data has been loaded:
[[See Video to Reveal this Text or Code Snippet]]
Updated Full Code Example
Here’s how your full component would look after these changes:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By following these steps, you can avoid common pitfalls with useEffect and useState in React. Properly structuring your asynchronous calls ensures that your component behaves predictably and efficiently. Remember, the key is to separate your function and structure the useEffect to simply call this function upon the component’s mount. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: useEffect is not called unless a not related useState is set to true
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the useEffect Issue in React
If you're new to React, you might find yourself confronting a puzzling problem: Why is the useEffect not being called unless a disconnected useState is triggered? Many developers, especially beginners, encounter this scenario while trying to fetch data from an API. Let’s break down the issue and explore a solution that can help streamline your data fetching in React.
The Problem with useEffect
You have a React component that tries to fetch a list of wines from an API when the component mounts. The code looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
In this code, you noticed that useEffect never gets triggered until you force a state change by setting the initial isDataLoading state to true. Why does this happen? Let's look at the solution.
Solving the useEffect Issue
Step 1: Move Your Async Function Outside of useEffect
One common mistake is nesting the asynchronous function within the useEffect. Instead, you can define the fetchWine function outside of it. This slight restructuring lets useEffect focus solely on executing the function on mount:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Call the Function Inside useEffect
Now, keep useEffect focused on calling this function:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Set Loading State Effectively
You also want to manage the loading state correctly. Set isDataLoading to true only when you start fetching the data, and set it back to false once the data has been loaded:
[[See Video to Reveal this Text or Code Snippet]]
Updated Full Code Example
Here’s how your full component would look after these changes:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By following these steps, you can avoid common pitfalls with useEffect and useState in React. Properly structuring your asynchronous calls ensures that your component behaves predictably and efficiently. Remember, the key is to separate your function and structure the useEffect to simply call this function upon the component’s mount. Happy coding!