filmov
tv
Understanding TypeError: cannot unpack non-iterable function object in Python
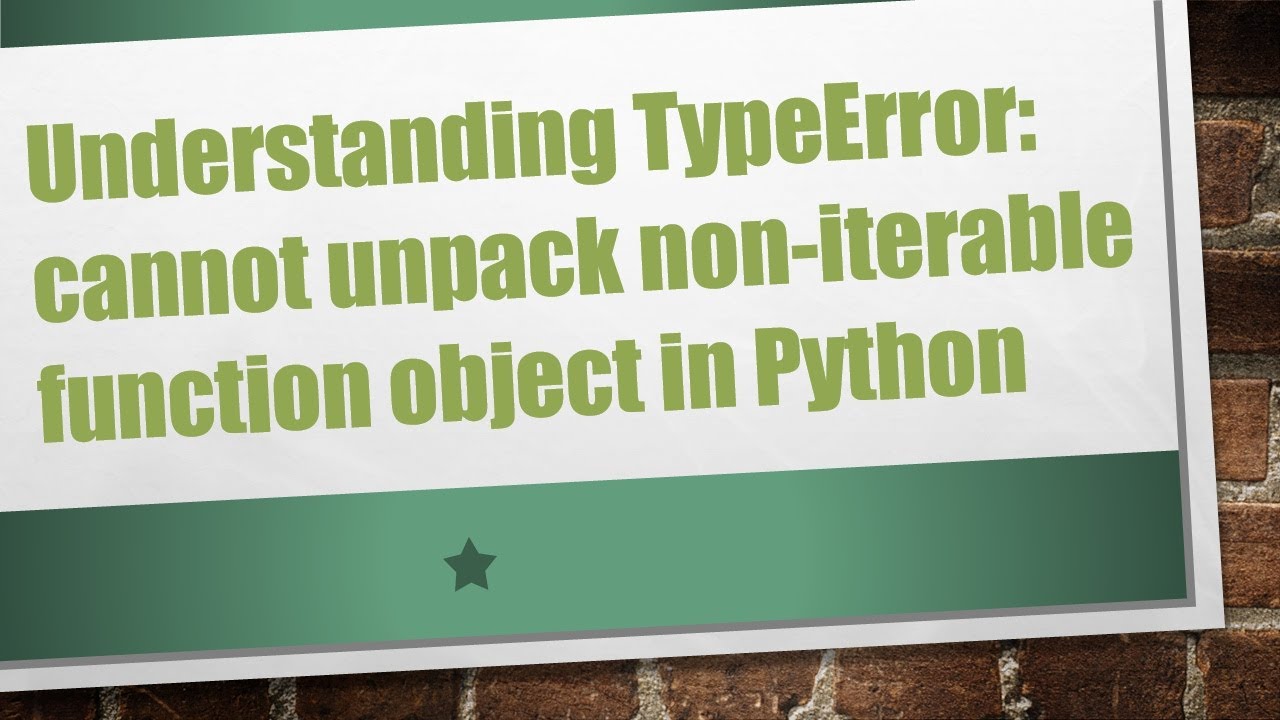
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to resolve the common Python error "TypeError: cannot unpack non-iterable function object" with practical examples for better debugging.
---
Understanding TypeError: cannot unpack non-iterable function object in Python
Introduction
If you've been working with Python, you might have encountered the error: TypeError: cannot unpack non-iterable function object. This error can be quite confusing, especially for beginners. This guide will help you understand why this error occurs and how you can resolve it with practical examples.
What Causes the Error?
The error "TypeError: cannot unpack non-iterable function object" typically appears when you try to unpack an object that is not iterable. In Python, iterables are objects like lists, tuples, and strings that can be looped over. Unpacking refers to assigning items from an iterable to multiple variables.
Consider the following code snippet:
[[See Video to Reveal this Text or Code Snippet]]
This will result in:
[[See Video to Reveal this Text or Code Snippet]]
Here, my_function returns a single string "hi", which is not iterable in the context of tuple unpacking to a and b.
How to Fix It?
Return an Iterable:
Ensure the function returns an iterable when you expect to unpack it:
[[See Video to Reveal this Text or Code Snippet]]
Direct Assignment:
If you're only dealing with a single value, assign it directly rather than unpacking:
[[See Video to Reveal this Text or Code Snippet]]
Check the Return Value:
Always ensure the function's return value aligns with your unpacking expectations. Debugging with print statements or using a debugger can help you ascertain what the function actually returns.
[[See Video to Reveal this Text or Code Snippet]]
Common Pitfalls
Returning None:
If a function does not have a return statement, it implicitly returns None, leading to a similar unpacking issue:
[[See Video to Reveal this Text or Code Snippet]]
This will throw:
[[See Video to Reveal this Text or Code Snippet]]
Solution: Ensure your function returns the expected iterable:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
The TypeError: cannot unpack non-iterable function object is a common issue that arises due to misunderstanding of what a function returns and how it should be handled. By ensuring that your functions return the expected iterables and aligning your unpacking logic accordingly, you can avoid this error and write cleaner, more efficient Python code.
Happy Coding!
---
Summary: Learn how to resolve the common Python error "TypeError: cannot unpack non-iterable function object" with practical examples for better debugging.
---
Understanding TypeError: cannot unpack non-iterable function object in Python
Introduction
If you've been working with Python, you might have encountered the error: TypeError: cannot unpack non-iterable function object. This error can be quite confusing, especially for beginners. This guide will help you understand why this error occurs and how you can resolve it with practical examples.
What Causes the Error?
The error "TypeError: cannot unpack non-iterable function object" typically appears when you try to unpack an object that is not iterable. In Python, iterables are objects like lists, tuples, and strings that can be looped over. Unpacking refers to assigning items from an iterable to multiple variables.
Consider the following code snippet:
[[See Video to Reveal this Text or Code Snippet]]
This will result in:
[[See Video to Reveal this Text or Code Snippet]]
Here, my_function returns a single string "hi", which is not iterable in the context of tuple unpacking to a and b.
How to Fix It?
Return an Iterable:
Ensure the function returns an iterable when you expect to unpack it:
[[See Video to Reveal this Text or Code Snippet]]
Direct Assignment:
If you're only dealing with a single value, assign it directly rather than unpacking:
[[See Video to Reveal this Text or Code Snippet]]
Check the Return Value:
Always ensure the function's return value aligns with your unpacking expectations. Debugging with print statements or using a debugger can help you ascertain what the function actually returns.
[[See Video to Reveal this Text or Code Snippet]]
Common Pitfalls
Returning None:
If a function does not have a return statement, it implicitly returns None, leading to a similar unpacking issue:
[[See Video to Reveal this Text or Code Snippet]]
This will throw:
[[See Video to Reveal this Text or Code Snippet]]
Solution: Ensure your function returns the expected iterable:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
The TypeError: cannot unpack non-iterable function object is a common issue that arises due to misunderstanding of what a function returns and how it should be handled. By ensuring that your functions return the expected iterables and aligning your unpacking logic accordingly, you can avoid this error and write cleaner, more efficient Python code.
Happy Coding!