filmov
tv
C String Library and String Copy Function - strcpy()
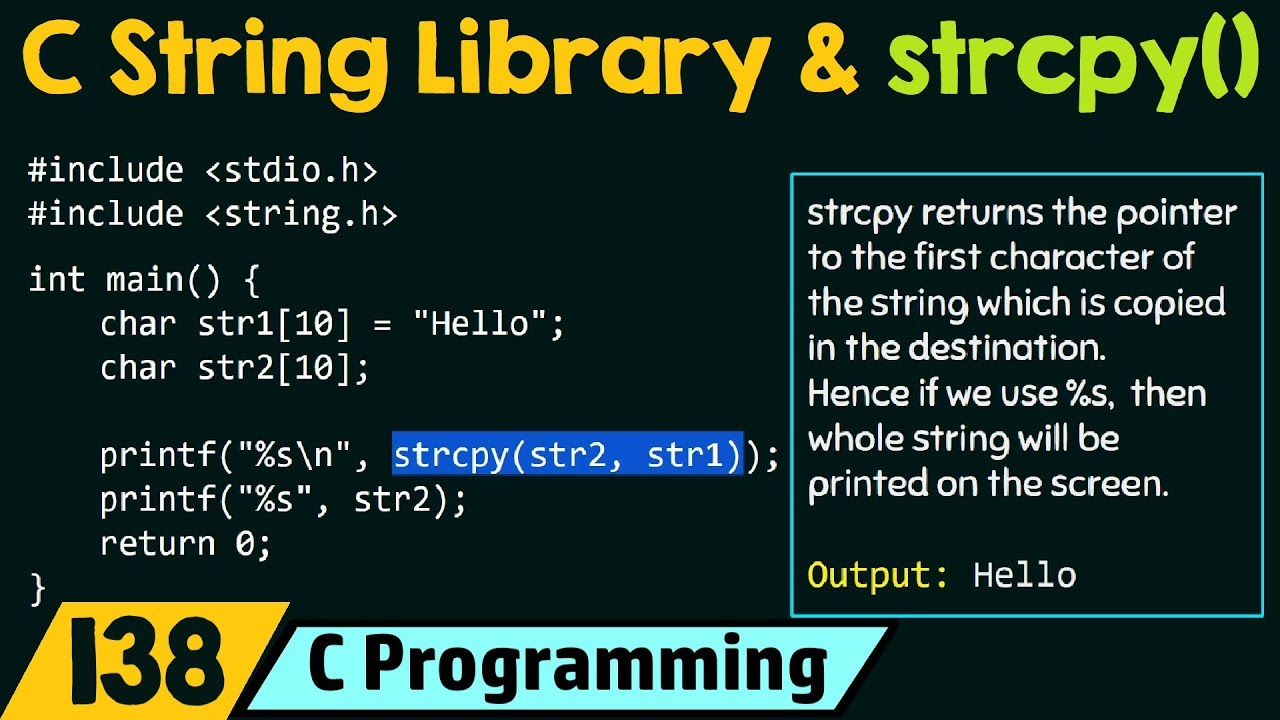
Показать описание
C Programming: C String Library and strcpy() Function in C Programming.
Topics discussed:
1) Introduction to C string library.
2) String Copy function (strcpy).
3) The prototype of strcpy() function.
4) Example use of strcpy() function.
5) The prototype of strncpy() function.
6) Example use of strncpy() function.
Music:
Axol x Alex Skrindo - You [NCS Release]
#CProgrammingByNeso #CProgramming #Strings #StringLibrary #strcpy #StringsInC
Topics discussed:
1) Introduction to C string library.
2) String Copy function (strcpy).
3) The prototype of strcpy() function.
4) Example use of strcpy() function.
5) The prototype of strncpy() function.
6) Example use of strncpy() function.
Music:
Axol x Alex Skrindo - You [NCS Release]
#CProgrammingByNeso #CProgramming #Strings #StringLibrary #strcpy #StringsInC
C string functions 🔠
C String Library and String Copy Function - strcpy()
String In Char Array VS. Pointer To String Literal | C Programming Tutorial
#22 C String Functions | C Programming For Beginners
C-Programmierung #17: Strings und die Bibliothek string.h
Creating My Own String Library in C
String Basics | C Programming Tutorial
String Functions in C Programming | strcat(), strcpy(), strcmp(), strstr(), strlen(), strchr() etc.
3335. Total Characters in String After Transformations I (Leetcode Medium)
string library functions in c | strncmp, strrev, strstr |
C-string functions: strlen, strcpy, strcat, strncpy, strncat, strcmp, strstr
strcpy in c programming | string library function |
String Comparison Function - strcmp()
Learn Standard String Library Functions in C Language
strcat in c programming | string library function |
[Arabic] Fundamentals Of Programming With C++ #045 - String - What Is A String
String in C programming | Discuss about string functions and it's use
String Functions In C & string.h Library: C Tutorial In Hindi #35
String Length Function - strlen()
[ Library Of Ruina ] String Theocracy
Mili - String Theocracy / 'Library of Ruina' theme song
C++20 String Formatting Library: An Overview and Use with Custom Types - Marc Gregoire - CppCon 2020
Count The Letters In A String | C Programming Example
How To Return A String From A Function | C Programming Tutorial
Комментарии