filmov
tv
Converting a 3 Level Nested List to a 3 Level Nested Map in Java
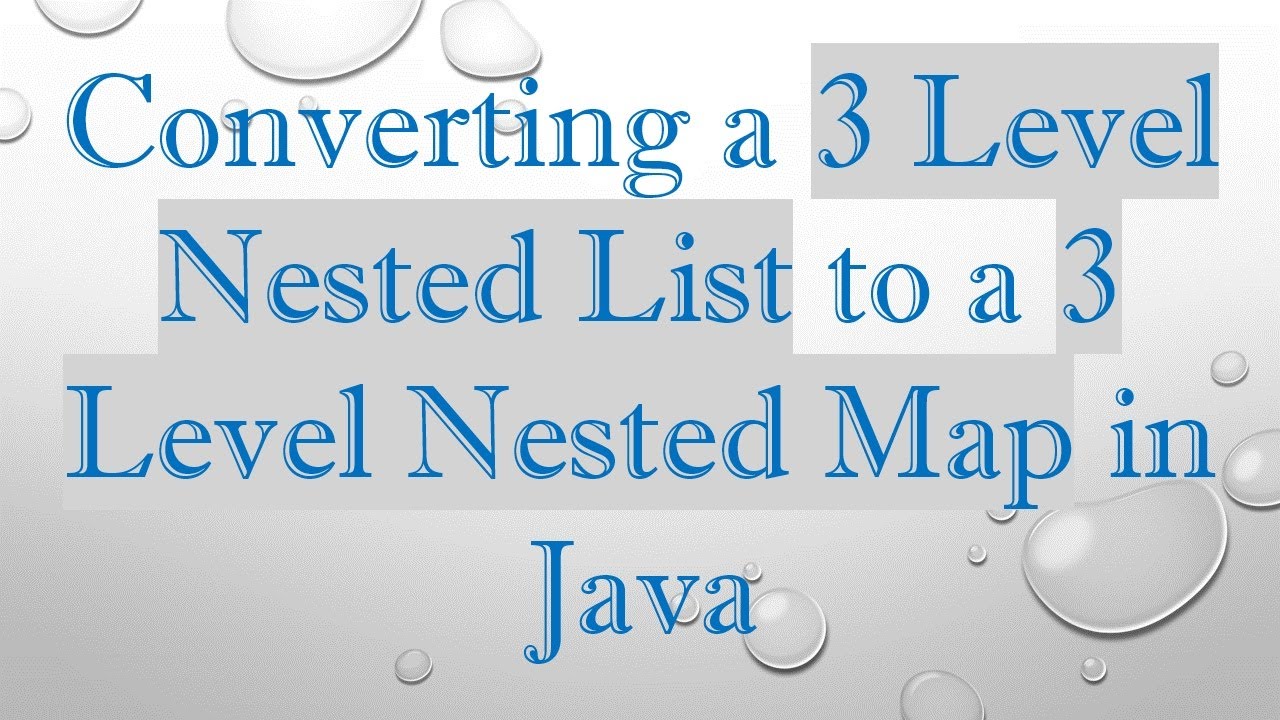
Показать описание
A step-by-step guide on how to convert a three-level nested list of countries, states, cities, and towns into a three-level nested map using Java streams.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Java 3 level nested list to 3 level nested map
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Converting a 3 Level Nested List to a 3 Level Nested Map in Java
If you're working on a Java project that involves managing hierarchical data structures, you might find yourself needing to convert a complex, nested list into maps for easier data manipulation and access. This guide explores how to convert a three-level nested list of countries, states, cities, and towns into a nested map structure using Java streams.
Understanding the Problem
Imagine you have a list structure representing countries, each containing states, each state containing cities, and each city containing towns. You want to convert this multi-level list structure into a more straightforward map format. This conversion will allow you to access the data more efficiently.
Data Structure
Here's a quick breakdown of the classes we are working with:
Country: Contains a name and a list of states.
State: Contains a name and a list of cities.
City: Contains a name and a list of towns.
Town: Contains a name and a population.
Desired Map Structure
You want to create maps in two different formats:
Using the whole object as keys:
1st level: State → Cities
2nd level: City → Towns
Using names as keys:
1st level: State's name → City's name
2nd level: City's name → Towns
The Solution
Step 1: Setting Up Your Classes
You should have classes defined for Country, State, City, and Town. Below is a simplified overview of how these might look in Java:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Converting Nested Lists to Maps
Using Java streams, you can accomplish the transformation between the nested list and map structure seamlessly.
Using Whole Objects as Keys
Here’s how to convert the nested list to a map where the keys are the whole state and city objects:
[[See Video to Reveal this Text or Code Snippet]]
Using Names as Keys
For the second format where you're using names as keys, the solution looks similar but focuses on the names:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Example Input and Output
You can use the following input to test your conversion logic:
[[See Video to Reveal this Text or Code Snippet]]
The output for the maps will be structured, and you can print them to check the integrity:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Converting a three-level nested list to a nested map in Java using streams allows for more manageable data access patterns. This approach not only clarifies your data structure but also enhances your application's performance. By leveraging these methods, you can streamline the process of handling complex datasets in your Java applications.
Feel free to implement and test the above code snippets in your projects and remember to keep your data structures organized!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Java 3 level nested list to 3 level nested map
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Converting a 3 Level Nested List to a 3 Level Nested Map in Java
If you're working on a Java project that involves managing hierarchical data structures, you might find yourself needing to convert a complex, nested list into maps for easier data manipulation and access. This guide explores how to convert a three-level nested list of countries, states, cities, and towns into a nested map structure using Java streams.
Understanding the Problem
Imagine you have a list structure representing countries, each containing states, each state containing cities, and each city containing towns. You want to convert this multi-level list structure into a more straightforward map format. This conversion will allow you to access the data more efficiently.
Data Structure
Here's a quick breakdown of the classes we are working with:
Country: Contains a name and a list of states.
State: Contains a name and a list of cities.
City: Contains a name and a list of towns.
Town: Contains a name and a population.
Desired Map Structure
You want to create maps in two different formats:
Using the whole object as keys:
1st level: State → Cities
2nd level: City → Towns
Using names as keys:
1st level: State's name → City's name
2nd level: City's name → Towns
The Solution
Step 1: Setting Up Your Classes
You should have classes defined for Country, State, City, and Town. Below is a simplified overview of how these might look in Java:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Converting Nested Lists to Maps
Using Java streams, you can accomplish the transformation between the nested list and map structure seamlessly.
Using Whole Objects as Keys
Here’s how to convert the nested list to a map where the keys are the whole state and city objects:
[[See Video to Reveal this Text or Code Snippet]]
Using Names as Keys
For the second format where you're using names as keys, the solution looks similar but focuses on the names:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Example Input and Output
You can use the following input to test your conversion logic:
[[See Video to Reveal this Text or Code Snippet]]
The output for the maps will be structured, and you can print them to check the integrity:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Converting a three-level nested list to a nested map in Java using streams allows for more manageable data access patterns. This approach not only clarifies your data structure but also enhances your application's performance. By leveraging these methods, you can streamline the process of handling complex datasets in your Java applications.
Feel free to implement and test the above code snippets in your projects and remember to keep your data structures organized!