filmov
tv
Resolving ValueError: could not convert string to float in Python DataFrames
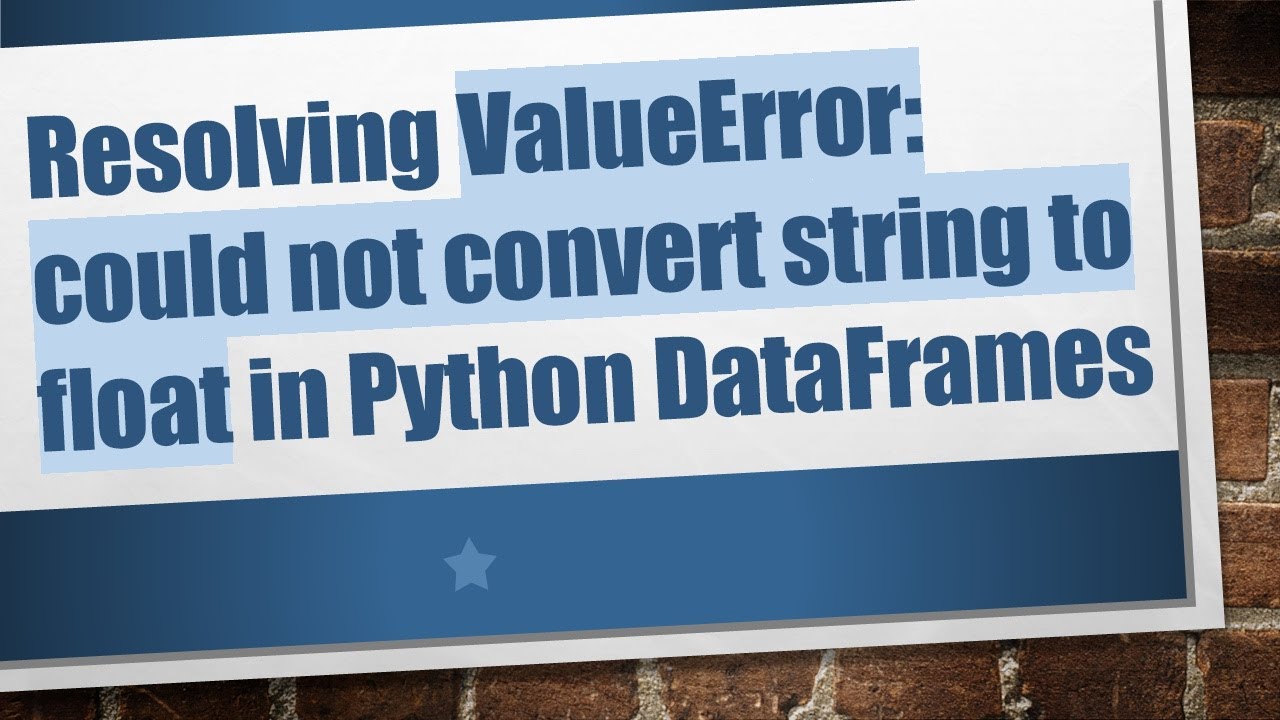
Показать описание
Learn how to efficiently handle fractions in Python Pandas DataFrames that cause ValueErrors during float conversions.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: ValueError: could not convert string to float while converting fractions
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Resolve the ValueError: could not convert string to float in Python DataFrames
When working with data, especially with odds or numerical values in string format, you might encounter frustrating errors. One of the more common ones is the ValueError: could not convert string to float—a challenge particularly prevalent when trying to convert fractions within a DataFrame. In this post, we’ll take you through an example of this problem and provide a structured approach to solve it.
The Problem: Encountering ValueError in DataFrame Operations
Imagine you have a Pandas DataFrame containing odds in a fraction format (like '167/50'). Ideally, you want to convert these strings into float values for analysis, but doing so directly raises a ValueError. As an example, here's a snippet of the data you're working with:
[[See Video to Reveal this Text or Code Snippet]]
When you try to convert these fractions using the following function:
[[See Video to Reveal this Text or Code Snippet]]
You receive the error:
[[See Video to Reveal this Text or Code Snippet]]
Let's dive into how to effectively resolve this issue.
The Solution: Utilizing applymap Instead of apply
To correct this situation, instead of using the apply method—which applies your function to a row or column—you should use applymap. This allows your function to treat each element of the DataFrame individually.
Revised Function Implementation
The revised version of the conversion function should include error handling to manage any potential issues during conversion. Here’s the updated function you can use:
[[See Video to Reveal this Text or Code Snippet]]
Applying the Function with applymap
Now, you can effectively apply this function to your DataFrame’s columns containing odds:
[[See Video to Reveal this Text or Code Snippet]]
This change ensures that every fraction is accurately converted to its float equivalent. Additionally, if any element in your DataFrame cannot be converted, it will now replace that element with None, which can be helpful for later checks.
Checking for NaN Values
To identify any rows that may have resulted in NaN values after conversion, you can print those rows for further inspection:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Handling data in Pandas can become tricky, especially with custom formats like fractions. By switching from apply to applymap and incorporating error handling, you can prevent the common ValueError and ensure your data conversion proceeds smoothly. This approach not only makes your code more robust but also maintains the integrity of your analysis.
By implementing the above suggestions, you can confidently manage odd formats in a DataFrame without encountering conversion errors.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: ValueError: could not convert string to float while converting fractions
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Resolve the ValueError: could not convert string to float in Python DataFrames
When working with data, especially with odds or numerical values in string format, you might encounter frustrating errors. One of the more common ones is the ValueError: could not convert string to float—a challenge particularly prevalent when trying to convert fractions within a DataFrame. In this post, we’ll take you through an example of this problem and provide a structured approach to solve it.
The Problem: Encountering ValueError in DataFrame Operations
Imagine you have a Pandas DataFrame containing odds in a fraction format (like '167/50'). Ideally, you want to convert these strings into float values for analysis, but doing so directly raises a ValueError. As an example, here's a snippet of the data you're working with:
[[See Video to Reveal this Text or Code Snippet]]
When you try to convert these fractions using the following function:
[[See Video to Reveal this Text or Code Snippet]]
You receive the error:
[[See Video to Reveal this Text or Code Snippet]]
Let's dive into how to effectively resolve this issue.
The Solution: Utilizing applymap Instead of apply
To correct this situation, instead of using the apply method—which applies your function to a row or column—you should use applymap. This allows your function to treat each element of the DataFrame individually.
Revised Function Implementation
The revised version of the conversion function should include error handling to manage any potential issues during conversion. Here’s the updated function you can use:
[[See Video to Reveal this Text or Code Snippet]]
Applying the Function with applymap
Now, you can effectively apply this function to your DataFrame’s columns containing odds:
[[See Video to Reveal this Text or Code Snippet]]
This change ensures that every fraction is accurately converted to its float equivalent. Additionally, if any element in your DataFrame cannot be converted, it will now replace that element with None, which can be helpful for later checks.
Checking for NaN Values
To identify any rows that may have resulted in NaN values after conversion, you can print those rows for further inspection:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Handling data in Pandas can become tricky, especially with custom formats like fractions. By switching from apply to applymap and incorporating error handling, you can prevent the common ValueError and ensure your data conversion proceeds smoothly. This approach not only makes your code more robust but also maintains the integrity of your analysis.
By implementing the above suggestions, you can confidently manage odd formats in a DataFrame without encountering conversion errors.