filmov
tv
Session 06: Mastering Python Basics | Data Types, Variables, Boolean Operations
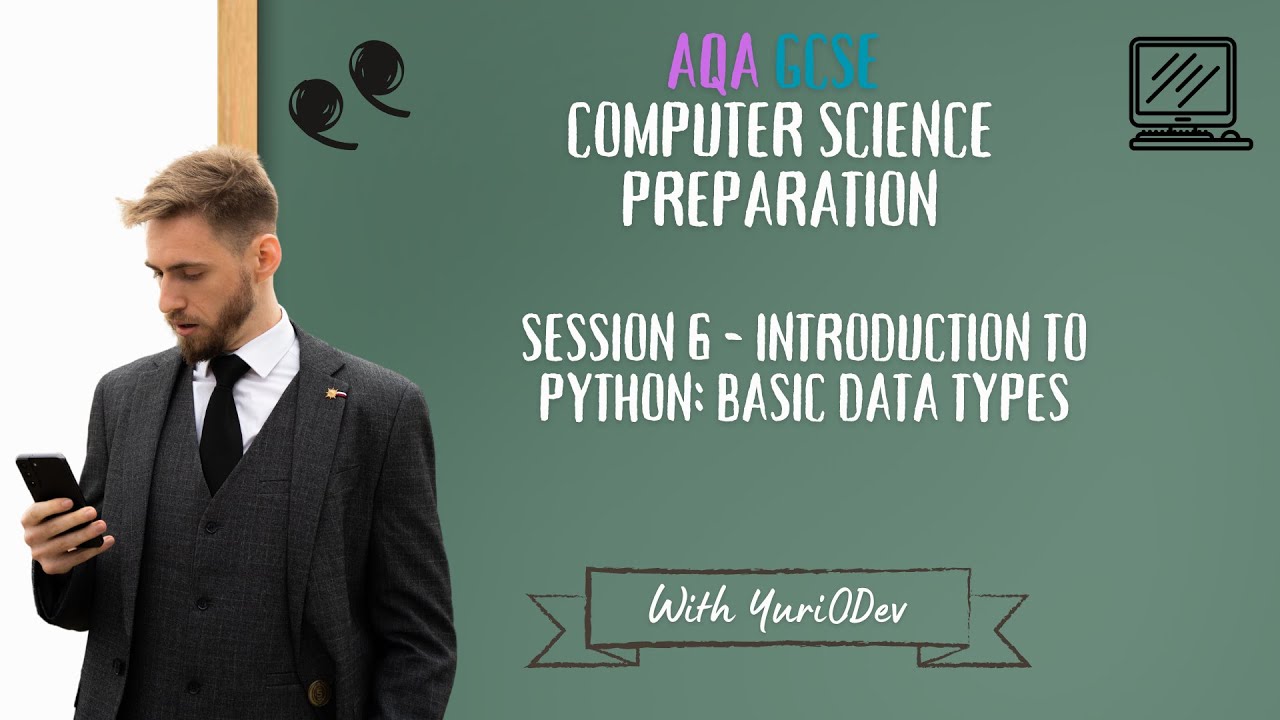
Показать описание
🎓 Session 06: Mastering Python Basics | Data Types, Variables, Boolean Operations
Welcome to the 6th session of our GCSE Computer Science series! In this video, we dive into advanced data types in Python, focusing on their unique properties, usage, and importance in programming. This session will give you a deeper understanding of how to use booleans, strings, and other data types efficiently and effectively.
Topics Covered:
🔢 Booleans: Understand true/false logic, operators, and conditions.
📝 Strings: Learn advanced string manipulation techniques and formatting with f-strings.
🧠 Type Conversion: Explore converting between integers, floats, and strings seamlessly.
🎛️ Practical Examples: Apply each data type in real-world coding scenarios.
💡 Key Concepts:
- Data types and their impact on memory and performance.
- Working with logical expressions in Python.
- Using formatted strings (f-strings) to dynamically embed variables.
- Practical insights into choosing the right data type for specific tasks.
Useful Resources:
🔗 Join the Community:
✨ Next Session: Control Structures in Python (Part 1) 📈
- 🧵 Sequence and Basic Syntax: Structuring code in logical sequences
- 🔀 Selection: Understanding if, elif, and else statements for decision-making
- 🌲 Nested Selection Structures: Building complex conditional logic with nested statements
👍 Don’t forget to subscribe for updates!
#PythonProgramming #Booleans #Strings #DataTypes #PythonTips #ComputerScience #PythonCoding #GCSE #PythonBasics #PythonCodingTutorials #LearnPython
⏰ Time codes:
0:00:00 Introduction to Python basics for GCSE
0:00:18 Lesson overview (objects, variables, data types)
0:00:24 True/False data types and keyboard input
0:00:34 Python IDE options (Google Colab, VS Code)
0:00:55 Data storage and memory
0:01:17 Memory for numbers vs. text
0:02:03 id() function and variable identities
0:02:34 Mutable vs. immutable objects
0:03:03 Python as a dynamic language
0:03:36 Using type() for data types
0:04:13 Objects in Python
0:04:48 Object identity for variables
0:05:29 Swapping variable values
0:06:32 Numbers and arithmetic operations
0:07:20 Exponentiation and bit-shifting
0:07:56 Floating-point numbers
0:08:39 Automatic conversion to float
0:09:06 Data type conversion (int to float, etc.)
0:10:24 Rounding experiments
0:11:48 Overview of mathematical functions
0:12:02 Using round() function
0:13:12 Finding min and max
0:13:25 Using abs() function
0:13:34 Strings and defining methods
0:14:02 String operations (concatenation, indexing)
0:14:20 Reserved keywords (e.g., sum)
0:15:09 Avoiding reserved words
0:15:48 Using str and common issues
0:16:03 Handling single and double quotes
0:17:00 Multi-line strings with triple quotes
0:18:02 Concatenating strings with +
0:18:42 String repetition with *
0:19:26 .format() string formatting
0:19:53 f-strings for formatting
0:20:43 Boolean data type (True, False)
0:21:03 Boolean assignment and capitalization
0:22:03 Mutable vs. immutable reminder
0:22:10 Comparison operators
0:24:01 Type casting (int to bool)
0:24:43 Non-zero values as True in Boolean
0:25:15 Converting strings to integers
0:26:00 Converting floats to integers
0:27:01 Boolean to string/integer conversion
0:28:00 Boolean and string conversion
0:29:47 Getting input with input()
0:30:14 Converting input data types
0:30:48 Input without conversion
0:31:46 Using comments in code (# and """ """)
0:32:58 Consistent spacing (PEP 8)
0:33:10 Compound assignments (+=, etc.)
0:33:32 f-strings for debugging
0:34:10 Compound assignments beyond addition
0:34:36 Exercise: Sum of digits
0:36:05 Exercise: Find larger number
0:39:07 Homework and giveaway announcement
0:40:01 Giveaway details (entry requirements)
0:41:01 Session summary (data types, input, giveaway)
Welcome to the 6th session of our GCSE Computer Science series! In this video, we dive into advanced data types in Python, focusing on their unique properties, usage, and importance in programming. This session will give you a deeper understanding of how to use booleans, strings, and other data types efficiently and effectively.
Topics Covered:
🔢 Booleans: Understand true/false logic, operators, and conditions.
📝 Strings: Learn advanced string manipulation techniques and formatting with f-strings.
🧠 Type Conversion: Explore converting between integers, floats, and strings seamlessly.
🎛️ Practical Examples: Apply each data type in real-world coding scenarios.
💡 Key Concepts:
- Data types and their impact on memory and performance.
- Working with logical expressions in Python.
- Using formatted strings (f-strings) to dynamically embed variables.
- Practical insights into choosing the right data type for specific tasks.
Useful Resources:
🔗 Join the Community:
✨ Next Session: Control Structures in Python (Part 1) 📈
- 🧵 Sequence and Basic Syntax: Structuring code in logical sequences
- 🔀 Selection: Understanding if, elif, and else statements for decision-making
- 🌲 Nested Selection Structures: Building complex conditional logic with nested statements
👍 Don’t forget to subscribe for updates!
#PythonProgramming #Booleans #Strings #DataTypes #PythonTips #ComputerScience #PythonCoding #GCSE #PythonBasics #PythonCodingTutorials #LearnPython
⏰ Time codes:
0:00:00 Introduction to Python basics for GCSE
0:00:18 Lesson overview (objects, variables, data types)
0:00:24 True/False data types and keyboard input
0:00:34 Python IDE options (Google Colab, VS Code)
0:00:55 Data storage and memory
0:01:17 Memory for numbers vs. text
0:02:03 id() function and variable identities
0:02:34 Mutable vs. immutable objects
0:03:03 Python as a dynamic language
0:03:36 Using type() for data types
0:04:13 Objects in Python
0:04:48 Object identity for variables
0:05:29 Swapping variable values
0:06:32 Numbers and arithmetic operations
0:07:20 Exponentiation and bit-shifting
0:07:56 Floating-point numbers
0:08:39 Automatic conversion to float
0:09:06 Data type conversion (int to float, etc.)
0:10:24 Rounding experiments
0:11:48 Overview of mathematical functions
0:12:02 Using round() function
0:13:12 Finding min and max
0:13:25 Using abs() function
0:13:34 Strings and defining methods
0:14:02 String operations (concatenation, indexing)
0:14:20 Reserved keywords (e.g., sum)
0:15:09 Avoiding reserved words
0:15:48 Using str and common issues
0:16:03 Handling single and double quotes
0:17:00 Multi-line strings with triple quotes
0:18:02 Concatenating strings with +
0:18:42 String repetition with *
0:19:26 .format() string formatting
0:19:53 f-strings for formatting
0:20:43 Boolean data type (True, False)
0:21:03 Boolean assignment and capitalization
0:22:03 Mutable vs. immutable reminder
0:22:10 Comparison operators
0:24:01 Type casting (int to bool)
0:24:43 Non-zero values as True in Boolean
0:25:15 Converting strings to integers
0:26:00 Converting floats to integers
0:27:01 Boolean to string/integer conversion
0:28:00 Boolean and string conversion
0:29:47 Getting input with input()
0:30:14 Converting input data types
0:30:48 Input without conversion
0:31:46 Using comments in code (# and """ """)
0:32:58 Consistent spacing (PEP 8)
0:33:10 Compound assignments (+=, etc.)
0:33:32 f-strings for debugging
0:34:10 Compound assignments beyond addition
0:34:36 Exercise: Sum of digits
0:36:05 Exercise: Find larger number
0:39:07 Homework and giveaway announcement
0:40:01 Giveaway details (entry requirements)
0:41:01 Session summary (data types, input, giveaway)
Комментарии