filmov
tv
Longest Substring with At Least K Repeating Characters | Leetcode 395 | Nov2020 Day 26 Challeneg
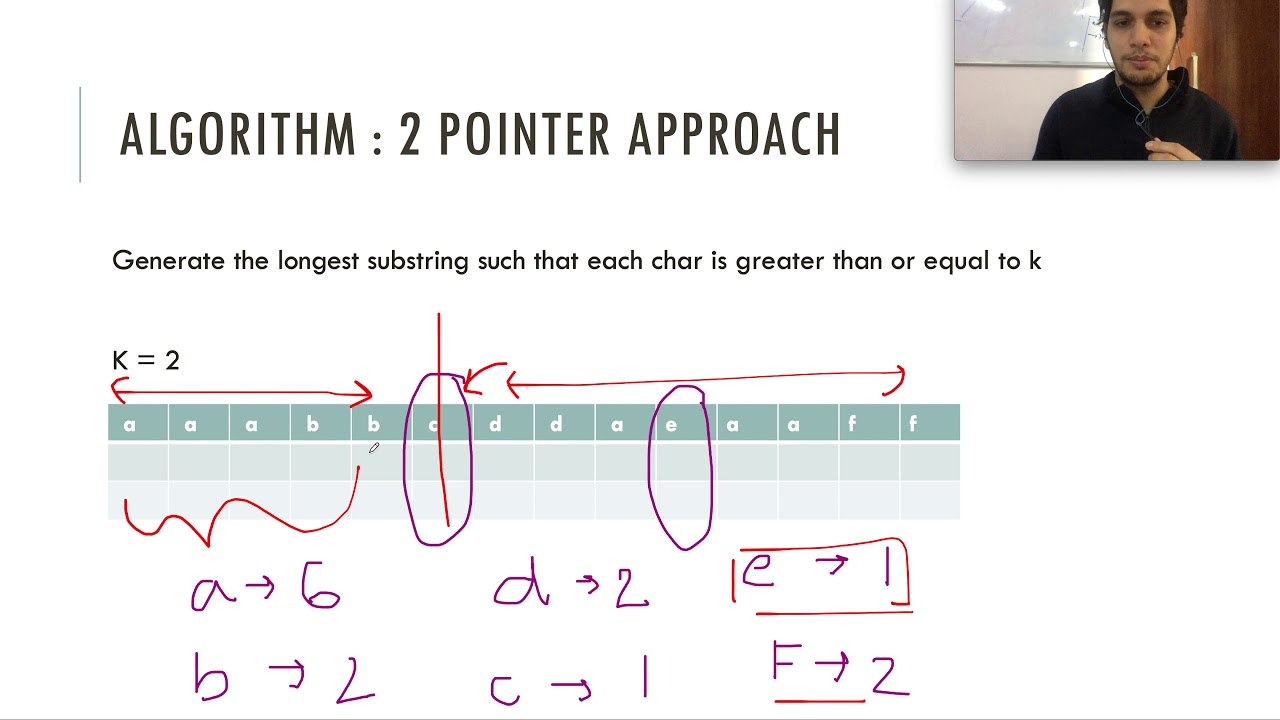
Показать описание
1) 0:00 Explaining the problem out loud
2) 1:16 Algorithm walkthrough
3) 3:30 Coding it up
4) 8:22 Time Complexity
TC : O(n2)
SC : O(1)
2) 1:16 Algorithm walkthrough
3) 3:30 Coding it up
4) 8:22 Time Complexity
TC : O(n2)
SC : O(1)
Longest Substring Without Repeating Characters - Leetcode 3 - Python
Sliding Window Algorithm Explained Clearly | Longest Substring Without Repeating Characters Leetcode
Longest Repeating Character Replacement - Leetcode 424 - Python
Longest Substring with At Least K Repeating Characters | Leetcode 395 | Nov2020 Day 26 Challeneg
Longest Substring with At Least K Repeating Characters | LeetCode 395 | C++, Java, Python
Find the Longest Substring Containing Vowels in Even Counts - Leetcode 1371 - Python
LeetCode Longest Substring Without Repeating Characters Solution Explained - Java
Longest Substring with At Least K Repeating Characters | Live Coding with Explanation | Leetcode#395
395. Longest Substring with At Least K Repeating Characters - Day 26/30 Leetcode November Challenge
Longest Substring with At Least K Repeating Characters | Leetcode 395
LeetCode 395. Longest Substring with At Least K Repeating Characters
Longest Substring Without Repeating Characters - Leetcode 3 - Sliding Window (Python)
LeetCode Exercise In Java - Longest Substring Without Repeating Characters - FAST Solution
Longest Substring with At Least K Repeating Characters - Two Pointers
Longest Substring with At Least K Repeating Characters | Sliding Window Technique | HashMap | Java
L3. Longest Substring Without Repeating Characters | 2 Pointers and Sliding Window Playlist
Longest Substring with At Least K Repeating Characters - LeetCode Problem 395
L6. Longest Substring With At Most K Distinct Characters | 2 Pointers and Sliding Window Playlist
395. Longest Substring with At Least K Repeating Characters
LeetCode 395. Longest Substring with At Least K Repeating Characters - Nov 26th - LeetCoding Challen
Longest Substring with At Least K Repeating Characters: Explained
Longest Common Subsequence - Dynamic Programming - Leetcode 1143
Find Longest Special Substring That Occurs Thrice I and II | Leetcode 2982 | Leetcode 2981
Amazon - Longest Substring Without Repeating Characters - Leetcode 3
Комментарии