filmov
tv
C++ Tutorial 10: Pointers Explained (Linked Lists vs Arrays Example)
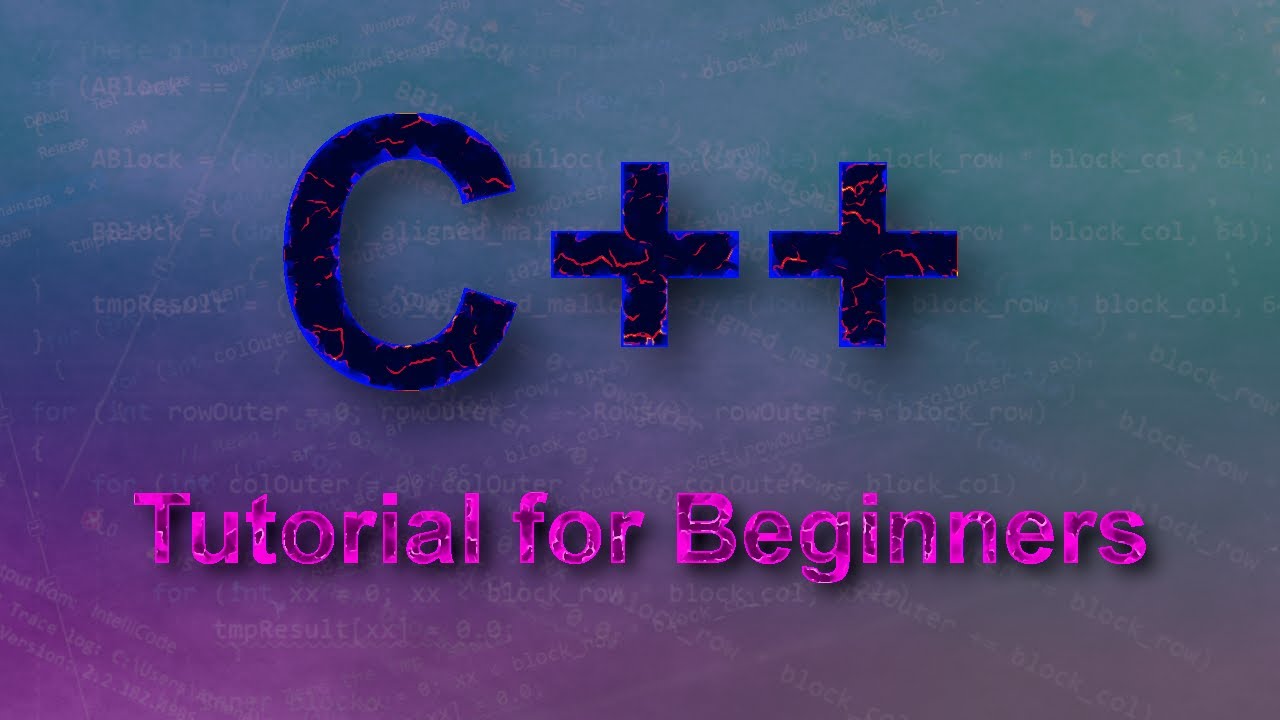
Показать описание
Today we'll look at basic pointers. We'll go through the concept of a pointer and the syntax but we're really only scratching the surface here. We'll look at pointer arithmetic and passing by reference and that sort of stuff in a later tutorial. My main goal is to show that pointers are more than a way for experienced programmers to show off to newbies, they are an integral and very powerful part of C++. They are not vague, magical or difficult to understand, they're just variables which hold the address of another variable.
C++ POINTERS (2025) - Introduction to C++ pointers (for beginners) PROGRAMMING TUTORIAL
C Programming Tutorial for Beginners 23 - Pointer in C programming | C Pointers (With Examples)
C Programming Tutorial for Beginners
C Language Tutorial for Beginners (with Notes & Practice Questions)
C Programmieren für Anfänger Tutorial #24 - Pointer auf Structures
Programmieren in C Tutorial #15 - Zeiger (Pointer)
C_62 Strings in C - part 1 | C programming tutorials
🔥 How to Trade Binary Options - LIVE SESSION with a TIPS, TUTORIALS and VIP SIGNALS
FS25 Map Making - Simple Powerlines - GE10 Tutorial
C Language Tutorial for Beginners (With Notes) 🔥
C_01 Introduction to C Language | C Programming Tutorials
C Tutorial Deutsch | Lerne C in 90 Minuten
C++ Tutorial for Beginners 21 - Pointers in C++
C++ Tutorial for Beginners - Full Course
C_10 Data Types in C - Part 1 | C Programming Tutorials for Beginners
#Q10 C Programming Quiz: Essential Multiple-Choice Questions for Beginners | Test Your Knowledge!
C++ Introduction | C ++ Tutorial | Mr. Kishore
C++ Tutorial For Beginners | C++ Programming | C++ | C++ Basics | C++ For Beginners | Simplilearn
2.4 Linked List Implementation in C/C++ | Creation and Display | DSA Tutorials
Curve 💫 or Knuckleball 💥 #shorts #footballskills #footballskills#neymar
Graffiti Tutorial #shorts
Learn C# with these 9 LINES OF CODE - Unity Tutorial!
Tutorial with Voiceover: Nails at home with Polygel with Dual Forms Easy Way #shorts #polygel #nails
MINI SHUFFLE TUTORIAL with Slow Motion by Viva Vici
Комментарии