filmov
tv
NumPy Array Operations: Indexing, Slicing, and Reshaping
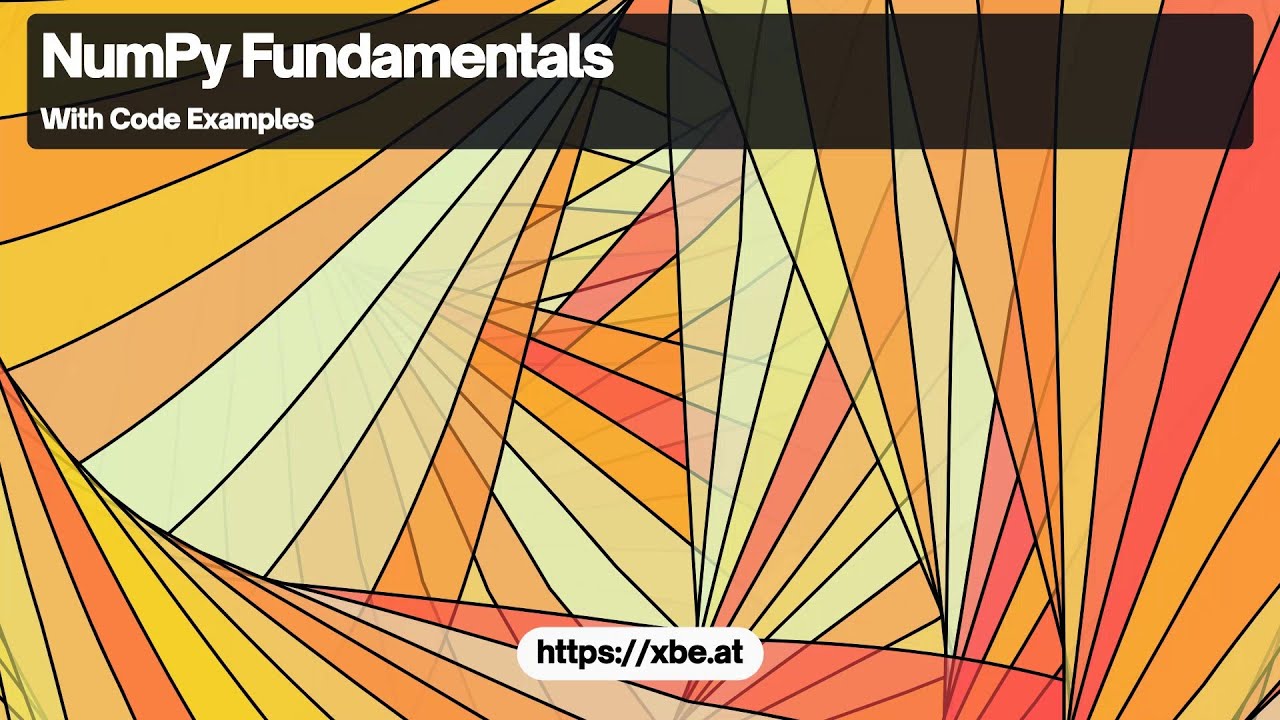
Показать описание
NumPy Array Operations: Indexing, Slicing, and Reshaping
💥💥 GET FULL SOURCE CODE AT THIS LINK 👇👇
NumPy is an open-source library for the Python programming language. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. In this description, we will cover three fundamental operations for manipulating NumPy arrays: indexing, slicing, and reshaping.
Indexing enables us to get specific values from an array based on their index position. We use integer indices, or labels, to locate data in the array.
```python
import numpy as np
print(arr[2]) # Output: 3
print(arr[0:2]) # Output: [1 2]
print(arr['all':2]) # Output: [1 2]
```
Slicing is used to extract subarrays by specifying a range of indices. The colon symbol (:) represents the entire axes of an array, whereas slices can be specified using indices and steps as well.
```python
print(arr[0:3]) # Output: [1 2 3]
print(arr[1::2]) # Output: [2 4]
```
Reshaping is used to transform an array into a different shape while preserving the data. It's useful when we need to convert data from a multidimensional form to a linear form or vice versa.
```python
print(arr_1d) # Output: [1 2 3 4 5 6 7 8 9]
print(arr_3d) # Output: array([[[1], [2], [3]],
[[4], [5], [6]],
[[7], [8], [9]]])
```
#STEM #Programming #Technology #Tutorial #numpy #array #operations #indexing #slicing #reshaping
Find this and all other slideshows for free on our website:
💥💥 GET FULL SOURCE CODE AT THIS LINK 👇👇
NumPy is an open-source library for the Python programming language. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. In this description, we will cover three fundamental operations for manipulating NumPy arrays: indexing, slicing, and reshaping.
Indexing enables us to get specific values from an array based on their index position. We use integer indices, or labels, to locate data in the array.
```python
import numpy as np
print(arr[2]) # Output: 3
print(arr[0:2]) # Output: [1 2]
print(arr['all':2]) # Output: [1 2]
```
Slicing is used to extract subarrays by specifying a range of indices. The colon symbol (:) represents the entire axes of an array, whereas slices can be specified using indices and steps as well.
```python
print(arr[0:3]) # Output: [1 2 3]
print(arr[1::2]) # Output: [2 4]
```
Reshaping is used to transform an array into a different shape while preserving the data. It's useful when we need to convert data from a multidimensional form to a linear form or vice versa.
```python
print(arr_1d) # Output: [1 2 3 4 5 6 7 8 9]
print(arr_3d) # Output: array([[[1], [2], [3]],
[[4], [5], [6]],
[[7], [8], [9]]])
```
#STEM #Programming #Technology #Tutorial #numpy #array #operations #indexing #slicing #reshaping
Find this and all other slideshows for free on our website: