filmov
tv
Build a Genetic Algorithm From Scratch In C++ 🧬 💻
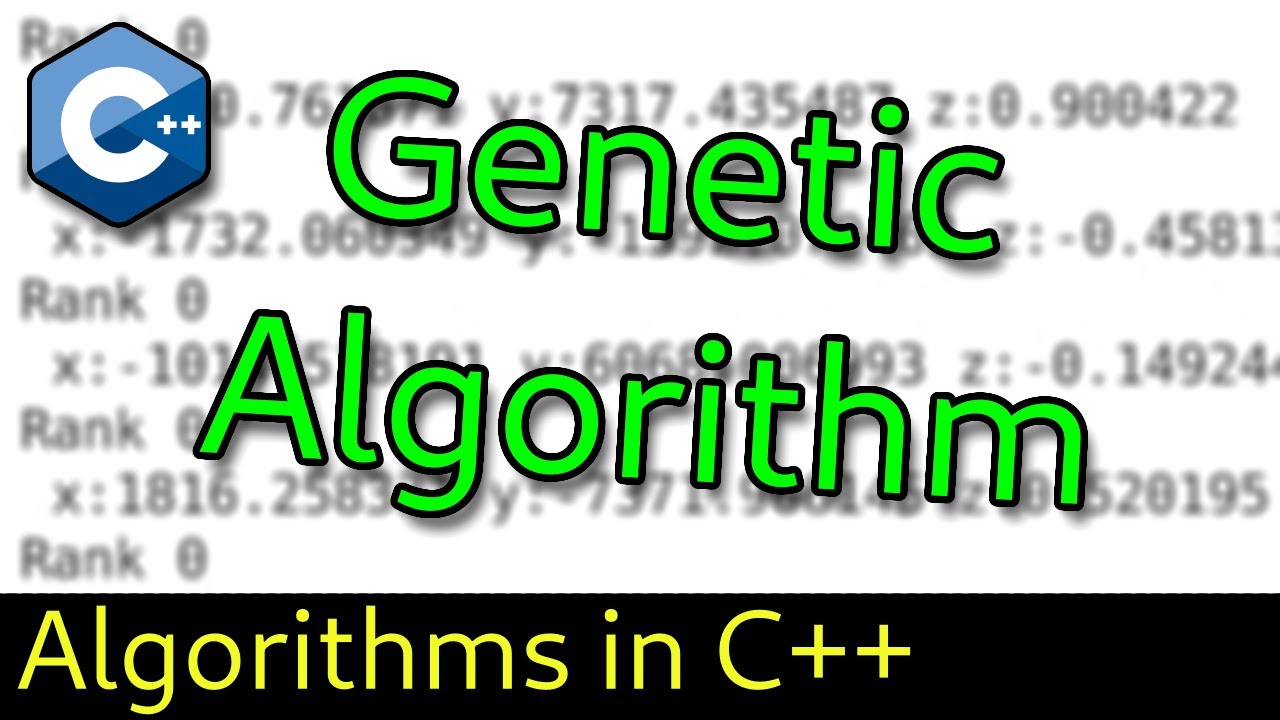
Показать описание
Genetic Algorithms are a family of evolutionary algorithms which can be implemented in any language (including C++) they solve problems which have no clear solution by generating random solutions and picking the best results then applying a crossover and a mutation to the best solutions before starting the process again.
Due to the "evolving" nature of this algorithm, the search space is greatly reduced in comparison to exhaustive search.
To learn more about Genetic Algorithms:
00:00 Intro
03:20 Initial solutions
06:40 Calculate fitness
07:20 Sort solutions
08:50 Print top initial solutions
12:40 Select top solutions
14:32 Mutation
15:57 Cross over
18:12 Review
22:12 Demo
#c #algorithm #geneticalgorithms #machinelearning
Due to the "evolving" nature of this algorithm, the search space is greatly reduced in comparison to exhaustive search.
To learn more about Genetic Algorithms:
00:00 Intro
03:20 Initial solutions
06:40 Calculate fitness
07:20 Sort solutions
08:50 Print top initial solutions
12:40 Select top solutions
14:32 Mutation
15:57 Cross over
18:12 Review
22:12 Demo
#c #algorithm #geneticalgorithms #machinelearning
Build a Genetic Algorithm From Scratch In C++ 🧬 💻
Genetic Algorithm In Python Super Basic Example
What are Genetic Algorithms?
Genetic Algorithm from Scratch in Python (tutorial with code)
Genetic algorithms explained in 6 minutes (...and 28 seconds)
Genetic Algorithm from Scratch in Python -- Full Walkthrough
How Artificial intelligence learns | Genetic Algorithm explained
Artificial intelligence for engineering design: Optimizing window sizes using a genetic algorithm
The Algorithm Whisperers - Particle Swarm Optimization in Search Engine Models
9.x: Genetic Algorithms and Evolutionary Computing - The Nature of Code
Genetic Algorithm Tutorial - How to Code a Genetic Algorithm
Genetic Algorithms In Trading: How To Automatically Generate Profitable Strategies! [FREE TRIAL]
Genetic Algorithms - Learn Python for Data Science #6
The Knapsack Problem & Genetic Algorithms - Computerphile
How Do Genetic Algorithms Work? | Two Minute Papers #32
Genetic Algorithms Explained By Example
AI Learns to be a Car using a Genetic Algorithm
Training a Neural Network using Genetic Algorithm
Genetic Algorithm Tutorial - An Overview of Genetic Algorithms
Genetic Algorithm in Python generates Music (code included)
Genetic Algorithm Learns How To Play Super Mario Bros!
314 - How to code the genetic algorithm in python?
Machine Learning Control: Genetic Algorithms
Unpacking the Genetic Algorithm
Комментарии