filmov
tv
Code Katas #5 - Solving Code Wars Katas and Talking Through My Process
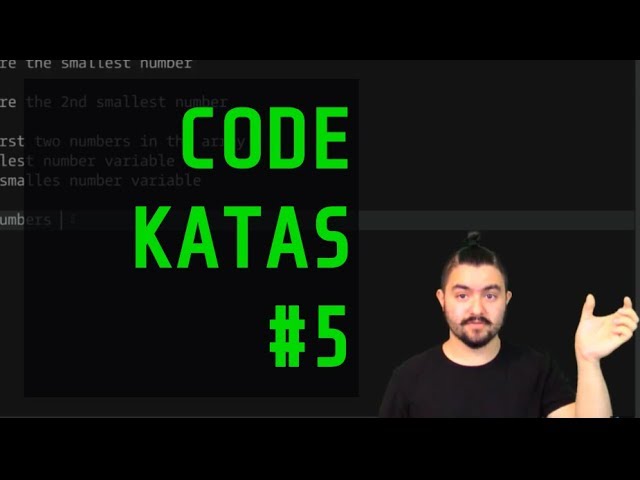
Показать описание
Show starts at 2:34
Code Katas #5 - Solving Code Wars Katas and Talking Through My Process
Codewars katas 5
Code Katas #7 - Solving Code Wars Katas Live and Talking Through My Process
Code Katas #15 - Solving Code Wars Code Katas and talking through my process
Code Katas #10 - Solving Code Wars Katas and Talking Through My Process
💪 | Code Katas #46 | Solving Code Wars Code Katas
Code Katas #13 - Solving Code Wars Katas and Talking Through My Process
Code Katas #6 - Watch me solve code katas live and talk through my process
Programming Coding Kata #5 Exercise
💪 | Code Katas #43 - Solving Code Wars Code katas
Code Katas #12 - Solving Code Wars Code Katas and Talking Through My Process
Kata 5 on Codewars: Human Readable Time (Javascript)
Sum of Digits/Digital Root - Codewars Popular Katas SOLVED #5
Code Katas #25 - Solving Code Wars Katas and Talking Through My Process
Finding Objects / Swift Code Katas #5: Tennis Score
code wars code katas part 5
python - primes in numbers (kata 5) codewars challenge solution using python | python programming
Code Katas #14 - Solving Code Wars Code Katas and talking through my process
python - the bee (kata 5) codewars challenge solution using python #shorts #viral
Code Review: Code Kata - Berlin Clock (5 Solutions!!)
Code kata within 5 mins
Code Katas #17: Solving Code Wars Katas and Talking Through My Process
Codewars in C#: Beginner Series Episode 1 (Level 8 Katas - 5 Challenges)
Code Katas #9 - Solving Code Wars Katas and Talking through my process
Комментарии