filmov
tv
How to Display Form Data Using JavaScript Alerts Effectively
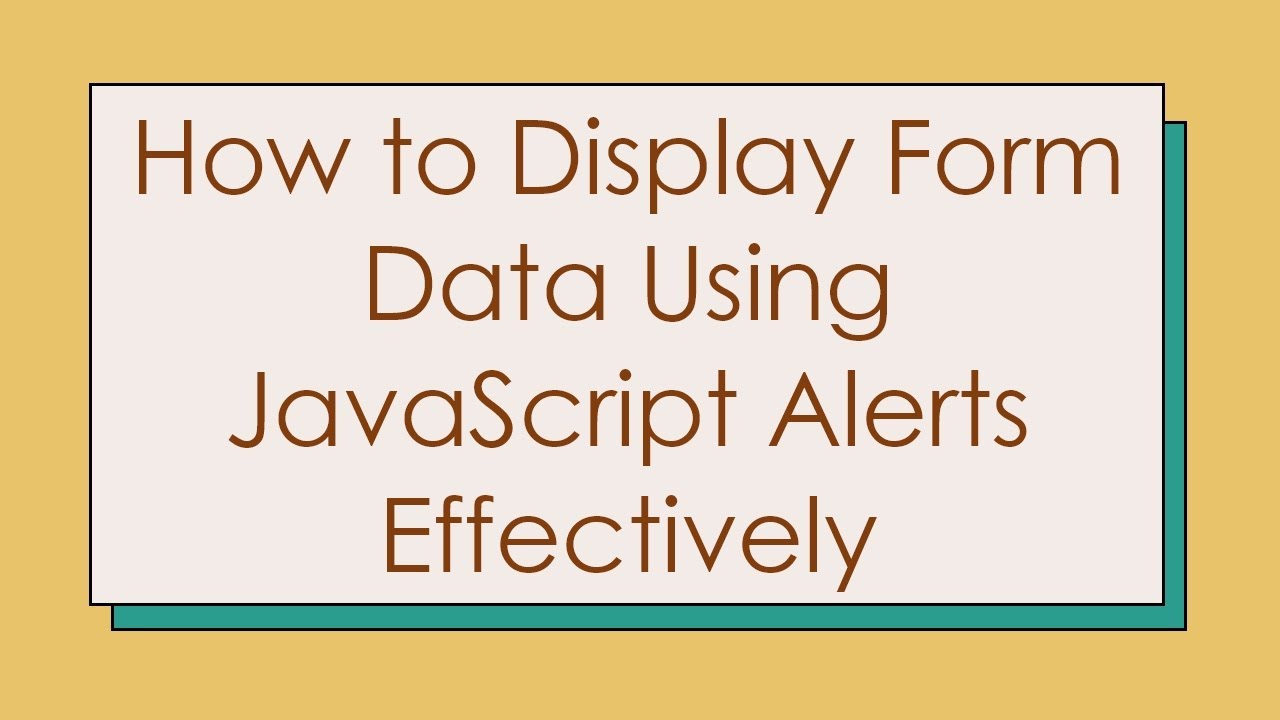
Показать описание
Learn how to properly display form information in alerts using JavaScript, including common mistakes and best practices for better code clarity.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Display form info on alert (html and javascript)
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Displaying Form Data in Alerts Using JavaScript
Creating interactive web forms can be a rewarding experience, especially when reaching out to users to gather information. However, developers, both novice and experienced, can encounter issues when trying to display user input from forms. If you've ever wondered why your alert doesn't show the expected form data when a button is clicked, you're not alone. In this guide, we will explore a common scenario and offer an effective solution to ensure your form data displays correctly in an alert box.
The Problem
Imagine you have a form with fields for user name, age, and a message. After filling out the form and clicking a submit button, you expect to see an alert showcasing this information. However, nothing happens. This is a common issue that can arise due to incorrect handling of form submission in your JavaScript code.
Sample Form Structure
Here is an example of the HTML form structure that might have led to confusion:
[[See Video to Reveal this Text or Code Snippet]]
Common Mistakes
Direct JavaScript function call on submit: Placing the onClick attribute directly in the submit button using onClick="submitForm()" doesn't handle the form submission correctly. This approach can lead to issues, especially if the function doesn't prevent the default submission behavior.
Using global variable references: Accessing form elements directly via getElementById can lead to errors if the form is not structured correctly or the element IDs are not unique.
The Solution
To effectively capture data from form fields and display it, we need to modify both the HTML form and the JavaScript function. Below are our updated versions that ensure everything works seamlessly.
HTML Form Update
First, we need to update the form to have a unique ID and modify the submit button to prevent the default submission.
[[See Video to Reveal this Text or Code Snippet]]
JavaScript Function Update
Now let's write the JavaScript function that will capture the data upon form submission while preventing the default behavior of the form:
[[See Video to Reveal this Text or Code Snippet]]
How It Works
Submit Event Handling: We attach an onsubmit event listener to the form. When the form is submitted, it executes our arrow function which does the following:
Displays the collected data in an alert.
Conclusion
By following the steps outlined above, you can successfully display form data via alerts in your JavaScript web applications. Avoid common pitfalls such as improperly binding event handlers and ensure you are correctly capturing the values of your input fields. These best practices not only help in writing better code but also enhance the overall user experience on your website.
Happy coding! If you have any questions or need further assistance, feel free to leave a comment below!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Display form info on alert (html and javascript)
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Displaying Form Data in Alerts Using JavaScript
Creating interactive web forms can be a rewarding experience, especially when reaching out to users to gather information. However, developers, both novice and experienced, can encounter issues when trying to display user input from forms. If you've ever wondered why your alert doesn't show the expected form data when a button is clicked, you're not alone. In this guide, we will explore a common scenario and offer an effective solution to ensure your form data displays correctly in an alert box.
The Problem
Imagine you have a form with fields for user name, age, and a message. After filling out the form and clicking a submit button, you expect to see an alert showcasing this information. However, nothing happens. This is a common issue that can arise due to incorrect handling of form submission in your JavaScript code.
Sample Form Structure
Here is an example of the HTML form structure that might have led to confusion:
[[See Video to Reveal this Text or Code Snippet]]
Common Mistakes
Direct JavaScript function call on submit: Placing the onClick attribute directly in the submit button using onClick="submitForm()" doesn't handle the form submission correctly. This approach can lead to issues, especially if the function doesn't prevent the default submission behavior.
Using global variable references: Accessing form elements directly via getElementById can lead to errors if the form is not structured correctly or the element IDs are not unique.
The Solution
To effectively capture data from form fields and display it, we need to modify both the HTML form and the JavaScript function. Below are our updated versions that ensure everything works seamlessly.
HTML Form Update
First, we need to update the form to have a unique ID and modify the submit button to prevent the default submission.
[[See Video to Reveal this Text or Code Snippet]]
JavaScript Function Update
Now let's write the JavaScript function that will capture the data upon form submission while preventing the default behavior of the form:
[[See Video to Reveal this Text or Code Snippet]]
How It Works
Submit Event Handling: We attach an onsubmit event listener to the form. When the form is submitted, it executes our arrow function which does the following:
Displays the collected data in an alert.
Conclusion
By following the steps outlined above, you can successfully display form data via alerts in your JavaScript web applications. Avoid common pitfalls such as improperly binding event handlers and ensure you are correctly capturing the values of your input fields. These best practices not only help in writing better code but also enhance the overall user experience on your website.
Happy coding! If you have any questions or need further assistance, feel free to leave a comment below!