filmov
tv
Design HashMap | LeetCode 706 | C++, Java, Python
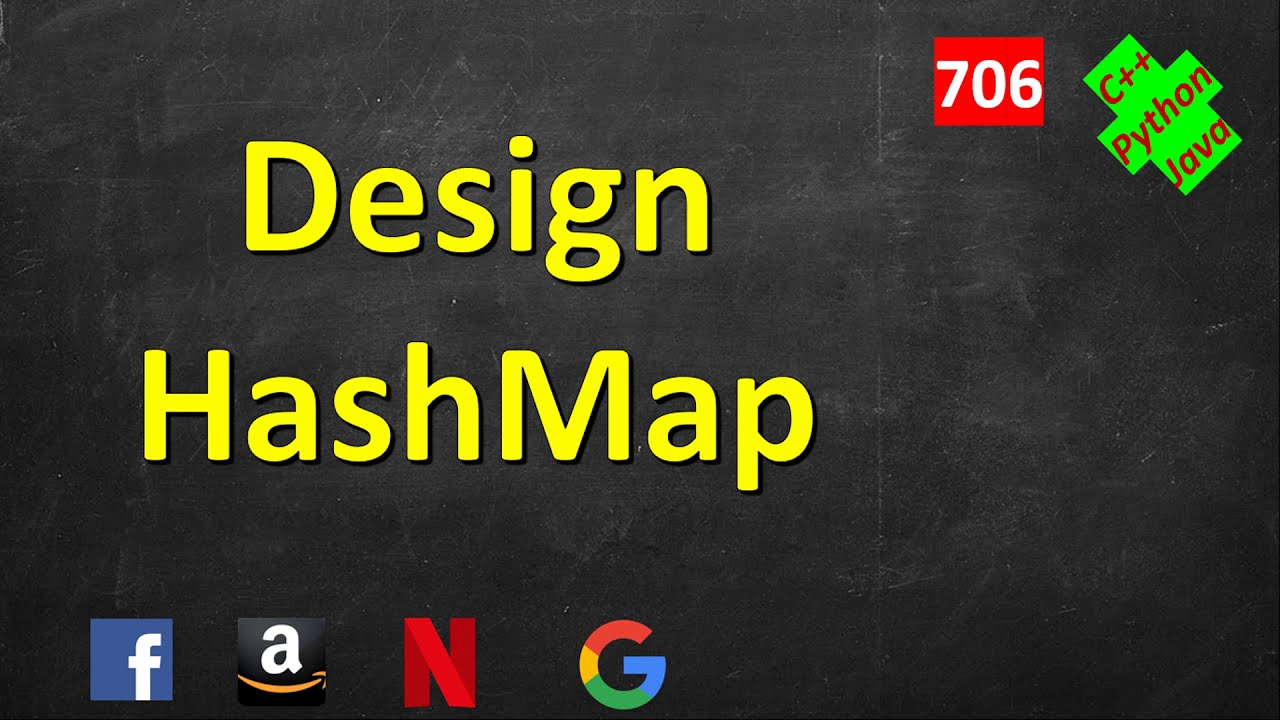
Показать описание
**** Best Books For Data Structures & Algorithms for Interviews:**********
*****************************************************************************
Design HashMap | Leetcode 706
Facebook Coding Interview question,
google coding interview question,
leetcode,
Design HashMap,
Design HashMap C++,
Design HashMap Java,
Design HashMap python,
Design HashMap solution,
706. Design HashMap,
#CodingInterview #LeetCode #Google #Amazon
Design Hashmap - Leetcode 706 - Python
Design HashMap | Live Coding with Explanation | Leetcode - 706
Design HashMap | Brute Force | Optimal | META | GOOGLE | NETFLIX | Leetcode - 706
Design HashMap | LeetCode 706 | C++, Java, Python
LeetCode 706 Design Hashmap
Design HashMap | leetcode 706
Design HashMap | LeetCode 706 | Coders Camp
706 design hashmap | 706 design hashmap leetcode
706. Design HashMap - Day 7/31 Leetcode March Challenge
Design HashMap - Leetcode 706 - Linked List - Python
Design HashMap | Leetcode - 706 | Easy | Amazon | Oracle | Google
Leetcode 706: Design HashMap
Design HashMap - Leetcode 706
LeetCode 706 : Design HashMap || EASY || C++ || JAVA || Detailed Solution
Leetcode 706. Design Hashmap
706. Design HashMap (List) | Leetcode | Python
Design HashMap - LeetCode #706 | Python, JavaScript, Java, C++
Design HashMap | leetcode 706
Design HashMap | Решение на Python | LeetCode 706
Design HashMap | Leetcode 706 | Binary Search Tree 🔥🔥 | Live coding session
706. Design HashMap | LeetCode | Beginners solution
Leetcode - Design HashMap (Python)
Leetcode | Easy | 706. Design HashMap | Javascript
Design HashMap | LeetCode 706
Комментарии