filmov
tv
Javascript Freecodecamp Algorithm #27: Find the Symmetric Difference
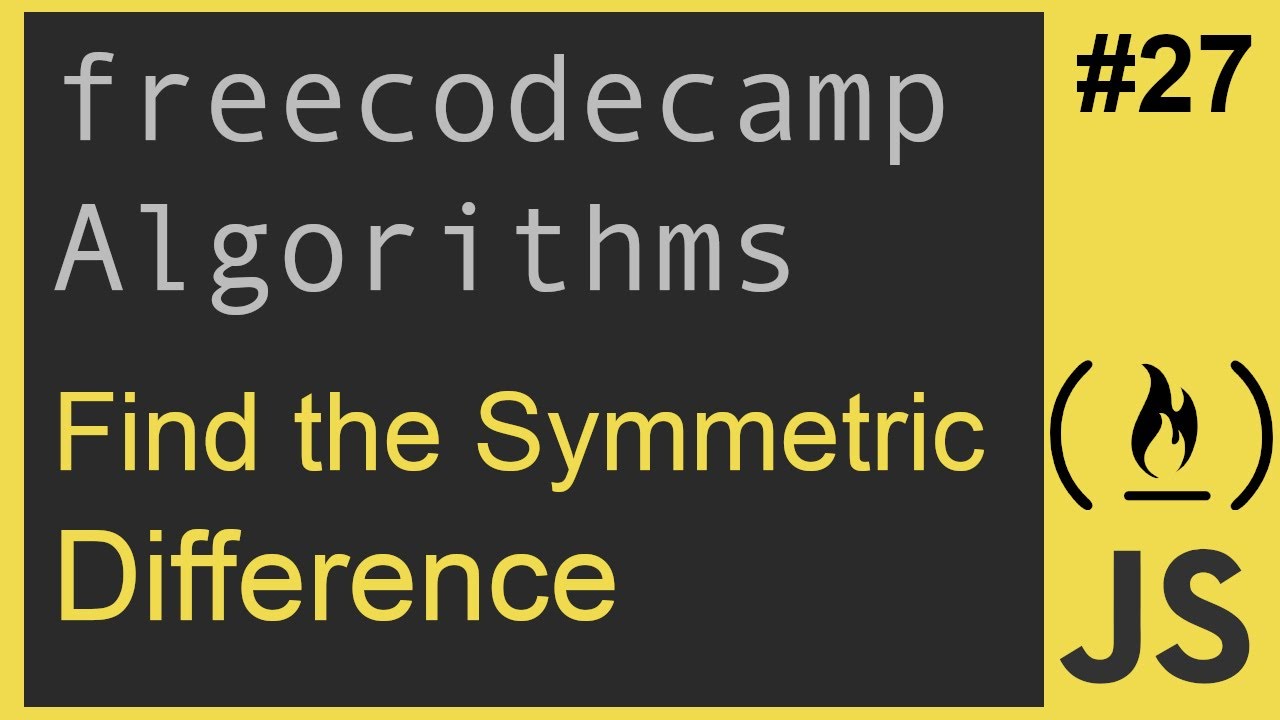
Показать описание
Learn how to solve freecodecamp javascript algorithms in various ways! This series is up-to-date with all ES6 and beyond javascript notations
🗄 Resources:
#javascript #algorithms #freecodecamp
🗄 Resources:
#javascript #algorithms #freecodecamp
Javascript Freecodecamp Algorithm #27: Find the Symmetric Difference
JavaScript Basic 27: Escape Squences | FreeCodeCamp | JS Algorithms and Data Structures
freeCodeCamp solutions - Basic JavaScript (27 - 55)
Javascript Freecodecamp Algorithm #28: Inventory Update
Data Structures and Algorithms in JavaScript - Full Course for Beginners
JavaScript Basic Algorithm Scripting | FreeCodeCamp
The HARDEST part about programming 🤦♂️ #code #programming #technology #tech #software #developer...
Free Code Camp Walkthrough 26 | JavaScript - Basic Algorithm Scripting
Free Code Camp Walkthrough 29 | JavaScript - Intermediate Algorithm Scripting
Javascript Freecodecamp Algorithm #3: Seek and Destroy
Intermediate Algorithm Scripting in Javascript - Freecodecamp
Javascript Freecodecamp Algorithm #6: Pig Latin
Intermediate Algorithm Scripting in Javascript - Freecodecamp
Basic Algorithm Scripting | FreeCodeCamp
Learn JavaScript - Full Course for Beginners
Coding for 1 Month Versus 1 Year #shorts #coding
freeCodeCamp solutions - Basic Algorithm Scripting (1 - 8)
Basic Algorithm Scripting (1/16) | Convert Celsius to Fahrenheit | freeCodeCamp
freeCodeCamp - All the solutions to JavaScript Algorithms and Data Structures (part 1)
IQ TEST
03 Prevent Object Mutation- FreeCodeCamp Solution - ES6 Javascript Algorithms & Data Structures ...
JavaScript Basic 45: The pop() Method | FreeCodeCamp | JS Algorithms and Data Structures
07 Match Anything with Wildcard Period - RegEx - FreeCodeCamp EXPLAINED - JavaScript Algorithms Data
Symmetric Difference Algorithm in 3 Steps: Advanced FreeCodeCamp Algorithm Tutorial (Part 1)
Комментарии