filmov
tv
How to Fix the TypeError: 'NoneType' object is not iterable in Python Code
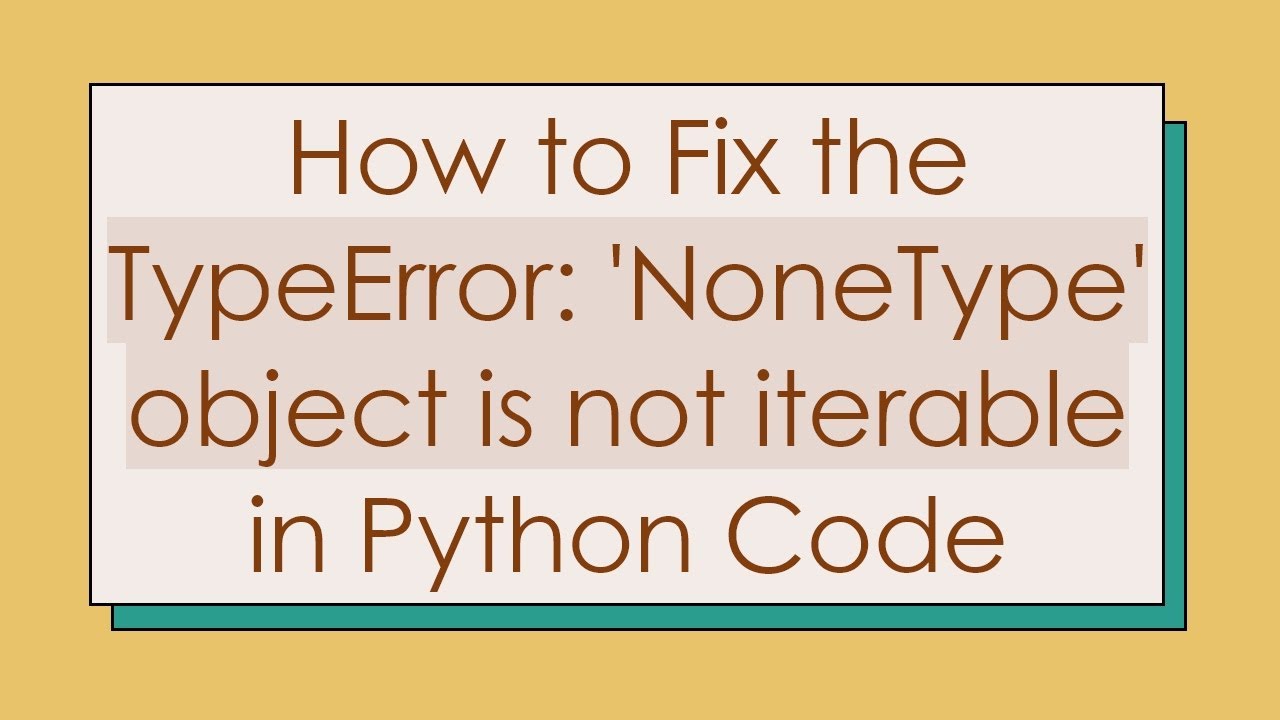
Показать описание
Discover how to resolve the frustrating `TypeError` caused by returning `None` instead of your list. Learn to fix your Python code and ensure it runs smoothly with practical solutions!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Not sure what's wrong here, no matter what I try it returns the TypeError: 'NoneType' object is not iterable
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Troubleshooting the TypeError in Your Python Code
If you've ever encountered the dreaded TypeError: 'NoneType' object is not iterable, you know how frustrating it can be. This particular error usually indicates that you are trying to loop through something that doesn't hold any iterable values, often because of returning None from a function. In this guide, we'll explore the root cause of this error in the context of a Python program that handles monthly sales data, and how to resolve it effectively.
The Problem
You have a Python program designed to manage the monthly sales, where you can view, edit, and summarize data. However, when trying to call the monthly function after editing values, you encounter the TypeError. Despite your efforts to debug, the issue persists, leading you to wonder if you've missed something in the code.
Understanding the Code Structure
Before diving into the solution, let’s revisit the key functions present in your program:
Monthly: Displays monthly sales.
Yearly: Summarizes total sales and the average.
Edit: Modifies sales for a particular month based on user input.
While the first two functions seem to work fine, you've identified that the Edit function is likely where the problem lies.
The Root Cause of the Error
Inside your Edit function, after successfully modifying the sales figure, you do not return the modified list mSales. Consequently, when you call monthlySales = Func.Edit(monthlySales), the variable monthlySales ends up being None. This None value causes the subsequent call to the Monthly function to fail, since None is not iterable.
Solution
To fix the issue, a simple line of code needs to be added to your Edit function. Here’s how you can do it:
Modify the Edit Function:
Add the line return mSales at the end of the Edit function. This change ensures that the modified monthly sales list is returned and can be used in the subsequent function calls.
Here’s the updated function:
[[See Video to Reveal this Text or Code Snippet]]
Recheck Your Main Loop:
Once you make this addition, ensure that your main method handles the input and output correctly:
[[See Video to Reveal this Text or Code Snippet]]
This line effectively captures the updated list of monthly sales after editing, preventing the NoneType error from occurring.
Conclusion
By ensuring the Edit function returns the modified sales list, you can avoid the TypeError: 'NoneType' object is not iterable. This change helps maintain the integrity of the monthlySales data throughout the program, allowing you to perform the desired operations seamlessly.
Remember, debugging often involves checking return statements and ensuring the data flow in your program is preserved. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Not sure what's wrong here, no matter what I try it returns the TypeError: 'NoneType' object is not iterable
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Troubleshooting the TypeError in Your Python Code
If you've ever encountered the dreaded TypeError: 'NoneType' object is not iterable, you know how frustrating it can be. This particular error usually indicates that you are trying to loop through something that doesn't hold any iterable values, often because of returning None from a function. In this guide, we'll explore the root cause of this error in the context of a Python program that handles monthly sales data, and how to resolve it effectively.
The Problem
You have a Python program designed to manage the monthly sales, where you can view, edit, and summarize data. However, when trying to call the monthly function after editing values, you encounter the TypeError. Despite your efforts to debug, the issue persists, leading you to wonder if you've missed something in the code.
Understanding the Code Structure
Before diving into the solution, let’s revisit the key functions present in your program:
Monthly: Displays monthly sales.
Yearly: Summarizes total sales and the average.
Edit: Modifies sales for a particular month based on user input.
While the first two functions seem to work fine, you've identified that the Edit function is likely where the problem lies.
The Root Cause of the Error
Inside your Edit function, after successfully modifying the sales figure, you do not return the modified list mSales. Consequently, when you call monthlySales = Func.Edit(monthlySales), the variable monthlySales ends up being None. This None value causes the subsequent call to the Monthly function to fail, since None is not iterable.
Solution
To fix the issue, a simple line of code needs to be added to your Edit function. Here’s how you can do it:
Modify the Edit Function:
Add the line return mSales at the end of the Edit function. This change ensures that the modified monthly sales list is returned and can be used in the subsequent function calls.
Here’s the updated function:
[[See Video to Reveal this Text or Code Snippet]]
Recheck Your Main Loop:
Once you make this addition, ensure that your main method handles the input and output correctly:
[[See Video to Reveal this Text or Code Snippet]]
This line effectively captures the updated list of monthly sales after editing, preventing the NoneType error from occurring.
Conclusion
By ensuring the Edit function returns the modified sales list, you can avoid the TypeError: 'NoneType' object is not iterable. This change helps maintain the integrity of the monthlySales data throughout the program, allowing you to perform the desired operations seamlessly.
Remember, debugging often involves checking return statements and ensuring the data flow in your program is preserved. Happy coding!