filmov
tv
Using Bit Operations to Toggle Last Two Flags in a 32-bit Integer
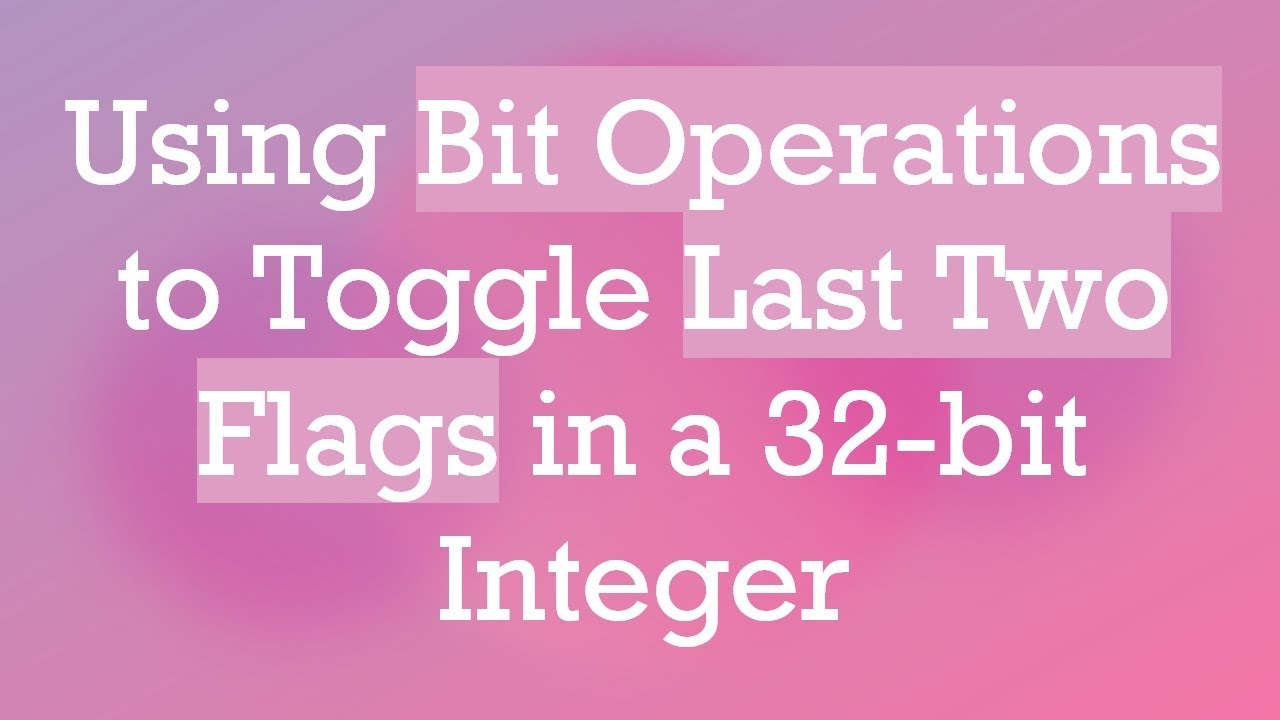
Показать описание
Explore how to effectively use bit operations in C programming to toggle the last two flags of a 32-bit integer.
---
Bit manipulation is a powerful technique in programming that allows you to perform efficient operations at the bit level. One common application is toggling specific bits, or flags, within an integer. This process can be particularly useful when you want to change the state of certain features or options represented by bits in a 32-bit integer.
When dealing with a 32-bit integer, each bit can be thought of as a flag that is either 'on' (1) or 'off' (0). Toggling a flag involves flipping its value: if the bit is 1, it becomes 0, and vice versa. For example, to toggle the last two bits (flags) in a 32-bit integer, you can utilize the XOR bitwise operation in C. This operation works by comparing corresponding bits of two operands and setting the result to 1 if the bits differ, and 0 if they are the same.
Step-by-Step Guide to Toggle the Last Two Bits
Understand the Binary Representation: Consider the binary representation of your 32-bit integer. For example, if your integer is n, the last two bits can be accessed or changed without affecting the other bits.
Use XOR Operation: The XOR operation is very effective for toggling bits. To toggle the last two bits, you use a mask where the last two bits are 1. The mask for this operation will be 0b11, which is equivalent to the decimal number 3.
Apply the XOR Mask: By performing n = n ^ 0b11 (or equivalently n = n ^ 3), you effectively toggle the last two bits of n. If the last two bits of n were 00, they become 11. If they were 01, they become 10, and so on.
Example in C Code
Here's a practical example to illustrate how you can toggle the last two bits using the XOR operation:
[[See Video to Reveal this Text or Code Snippet]]
In this code snippet, the initial number 0xA (binary 1010) becomes 0x9 (binary 1001) after toggling the last two bits.
Benefits of Using Bit Operations
Using bit operations is fast and efficient, often resulting in optimized performance in code execution. It's particularly useful in scenarios with limited resources, where every bit counts—such as in embedded systems or game development.
By mastering these operations, you can manipulate data more effectively, handle binary data formats, and improve the performance of your applications.
Understanding and applying these concepts will increase your versatility as a programmer, allowing you to manage data representation and control at a foundational level with precision and skill.
---
Bit manipulation is a powerful technique in programming that allows you to perform efficient operations at the bit level. One common application is toggling specific bits, or flags, within an integer. This process can be particularly useful when you want to change the state of certain features or options represented by bits in a 32-bit integer.
When dealing with a 32-bit integer, each bit can be thought of as a flag that is either 'on' (1) or 'off' (0). Toggling a flag involves flipping its value: if the bit is 1, it becomes 0, and vice versa. For example, to toggle the last two bits (flags) in a 32-bit integer, you can utilize the XOR bitwise operation in C. This operation works by comparing corresponding bits of two operands and setting the result to 1 if the bits differ, and 0 if they are the same.
Step-by-Step Guide to Toggle the Last Two Bits
Understand the Binary Representation: Consider the binary representation of your 32-bit integer. For example, if your integer is n, the last two bits can be accessed or changed without affecting the other bits.
Use XOR Operation: The XOR operation is very effective for toggling bits. To toggle the last two bits, you use a mask where the last two bits are 1. The mask for this operation will be 0b11, which is equivalent to the decimal number 3.
Apply the XOR Mask: By performing n = n ^ 0b11 (or equivalently n = n ^ 3), you effectively toggle the last two bits of n. If the last two bits of n were 00, they become 11. If they were 01, they become 10, and so on.
Example in C Code
Here's a practical example to illustrate how you can toggle the last two bits using the XOR operation:
[[See Video to Reveal this Text or Code Snippet]]
In this code snippet, the initial number 0xA (binary 1010) becomes 0x9 (binary 1001) after toggling the last two bits.
Benefits of Using Bit Operations
Using bit operations is fast and efficient, often resulting in optimized performance in code execution. It's particularly useful in scenarios with limited resources, where every bit counts—such as in embedded systems or game development.
By mastering these operations, you can manipulate data more effectively, handle binary data formats, and improve the performance of your applications.
Understanding and applying these concepts will increase your versatility as a programmer, allowing you to manage data representation and control at a foundational level with precision and skill.