filmov
tv
Mastering the Nested Object Loop in JavaScript
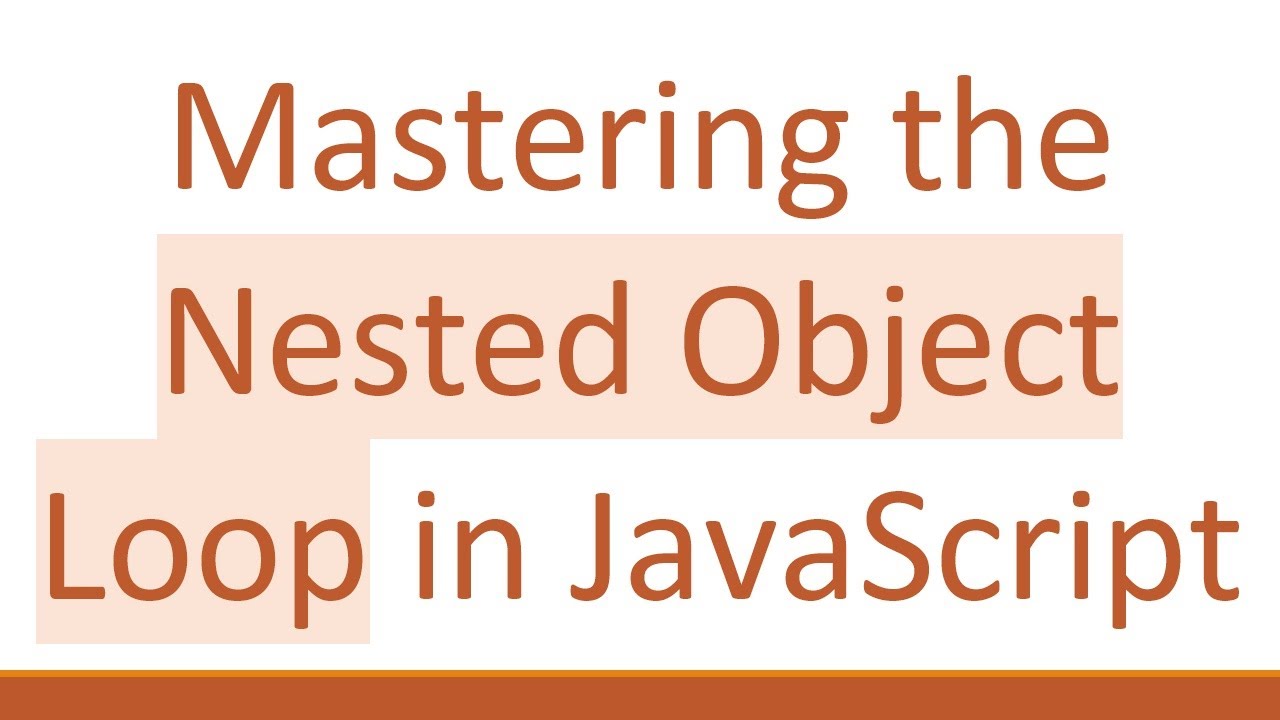
Показать описание
Discover how to effectively handle nested objects in JavaScript. Learn essential techniques and best practices to simplify your code and avoid common pitfalls.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Nested Object Loop in JS
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering the Nested Object Loop in JavaScript: A Comprehensive Guide
When working with JavaScript, we often encounter complex data structures like nested objects. These objects can be incredibly powerful for organizing data but can also be tricky to manipulate, especially when adding new values. In this post, we'll address a common issue faced by developers when trying to loop through a nested object and add values correctly. Let's break it down and find a solution that works!
The Challenge
You might come across a situation where you need to write a function to manage nested objects in JavaScript. You could see code similar to this:
[[See Video to Reveal this Text or Code Snippet]]
Upon examining the function write, you may notice that it's failing to properly add values to the settings object nested under the provided id. The error arises due to incorrect attempts to access object properties.
Understanding the Problem
In the original code, values is an object containing key-value pairs. The mistake occurs because values[x] does not return the key correctly for nested object manipulation; instead, it directly interprets the index x, which leads to an undefined value.
Common Mistake:
Accessing values[x] assumes values can be indexed like an array, which is incorrect when values is actually an object.
The Solution
To address this issue, we need to access the properties of the values object using its keys correctly. Here’s how to do it in a more effective way:
Updated Code:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of Changes:
Loop through Keys: Instead of indexing the values directly, we now loop through the keys array to access the properties correctly.
Assign Values: During the assignment, we now correctly reference the keys of values to set the corresponding values in the settings object.
What This Achieves:
With this updated approach, when calling the write function with the example parameters, you get a properly nested object with the values assigned correctly under the specified id. The console log will show you the structure of settings, which should look like this:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Navigating nested objects in JavaScript can be daunting, but with a clear understanding of how to access object properties with their keys, you can manage them effectively. By employing the solution provided, you can now confidently handle nested objects and avoid common pitfalls that come with improperly accessing object data.
By applying these principles, you can build more robust JavaScript applications that handle complex data efficiently. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Nested Object Loop in JS
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering the Nested Object Loop in JavaScript: A Comprehensive Guide
When working with JavaScript, we often encounter complex data structures like nested objects. These objects can be incredibly powerful for organizing data but can also be tricky to manipulate, especially when adding new values. In this post, we'll address a common issue faced by developers when trying to loop through a nested object and add values correctly. Let's break it down and find a solution that works!
The Challenge
You might come across a situation where you need to write a function to manage nested objects in JavaScript. You could see code similar to this:
[[See Video to Reveal this Text or Code Snippet]]
Upon examining the function write, you may notice that it's failing to properly add values to the settings object nested under the provided id. The error arises due to incorrect attempts to access object properties.
Understanding the Problem
In the original code, values is an object containing key-value pairs. The mistake occurs because values[x] does not return the key correctly for nested object manipulation; instead, it directly interprets the index x, which leads to an undefined value.
Common Mistake:
Accessing values[x] assumes values can be indexed like an array, which is incorrect when values is actually an object.
The Solution
To address this issue, we need to access the properties of the values object using its keys correctly. Here’s how to do it in a more effective way:
Updated Code:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of Changes:
Loop through Keys: Instead of indexing the values directly, we now loop through the keys array to access the properties correctly.
Assign Values: During the assignment, we now correctly reference the keys of values to set the corresponding values in the settings object.
What This Achieves:
With this updated approach, when calling the write function with the example parameters, you get a properly nested object with the values assigned correctly under the specified id. The console log will show you the structure of settings, which should look like this:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Navigating nested objects in JavaScript can be daunting, but with a clear understanding of how to access object properties with their keys, you can manage them effectively. By employing the solution provided, you can now confidently handle nested objects and avoid common pitfalls that come with improperly accessing object data.
By applying these principles, you can build more robust JavaScript applications that handle complex data efficiently. Happy coding!