filmov
tv
Fixing Recursion Depth Exceeded Error in Python Reverse Pair Counting Using MergeSort
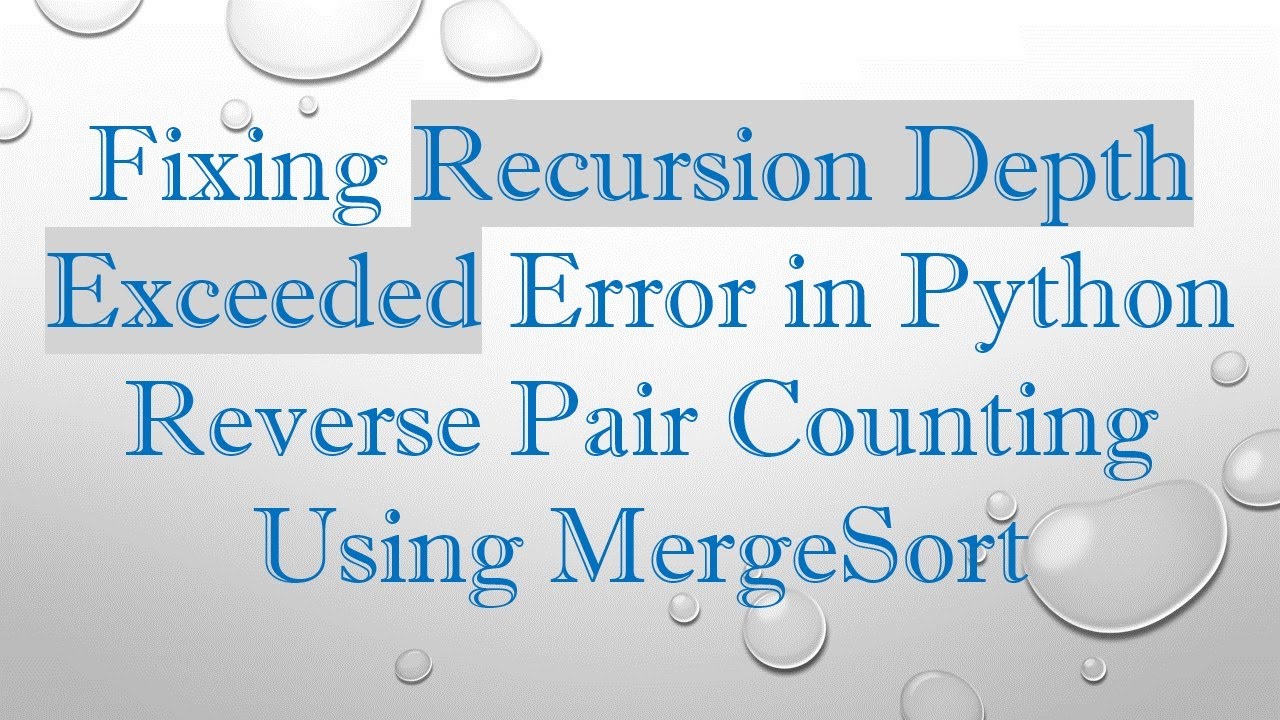
Показать описание
Learn how to solve the recursion depth issue when counting reverse pairs in Python using MergeSort. Follow clear steps and improve your code structure!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Recursion depth exceeding in a python function for counting reverse pairs
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Fixing Recursion Depth Exceeded Error in Python Reverse Pair Counting Using MergeSort
When working with algorithms, particularly those involving recursion, programmers can sometimes run into common issues. One such problem is encountering a recursion depth exceeded error. If you're trying to implement a solution for counting reverse pairs using a variation of the MergeSort algorithm in Python, you may find yourself facing this exact issue. In this guide, we’ll take a closer look at how to address this problem effectively.
What Are Reverse Pairs?
Reverse pairs are defined as pairs (i, j) such that i < j and nums[i] > 2 * nums[j]. Counting these pairs efficiently is important for many algorithms, especially those involving sorting. The MergeSort algorithm is a popular choice to implement this due to its O(n log n) complexity, making it more efficient than simpler methods that could take O(n^2) time.
The Problem at Hand
In the code example provided, the implementation of MergeSort tries to count reverse pairs by utilizing recursion. However, it hits a wall with a maximum recursion depth exceeded error specifically on this line:
[[See Video to Reveal this Text or Code Snippet]]
This indicates that the recursion is running too deep, likely due to an incorrect base case or an infinite loop within the recursive calls.
Breaking Down the Solution
1. Adjusting the Base Case
To solve the recursion depth issue, you need to modify the stopping condition for recursion. Instead of using:
[[See Video to Reveal this Text or Code Snippet]]
You should change it to:
[[See Video to Reveal this Text or Code Snippet]]
This modification ensures that the recursion halts once there's only one element left in the current slice, thus avoiding unnecessary function calls.
2. Fix the Inversion Count Calculation
The initial approach for counting inversions in the merge function contains flaws. Consider this piece of code:
[[See Video to Reveal this Text or Code Snippet]]
The loop uses range(mid) which is too broad. It should be limited to only the relevant section from left to mid. Adjust this to:
[[See Video to Reveal this Text or Code Snippet]]
This change ensures you are only iterating over the necessary elements.
3. Additional Updates in Merge Logic
The logic that determines whether an inversion exists should be better integrated into the merge process. You can simplify your inversion counting as follows:
[[See Video to Reveal this Text or Code Snippet]]
This way, every time an element from the right slice is selected, you accurately increase your revcount for all relevant inversions.
Conclusion
By implementing the changes outlined above, you should be able to avoid the recursion depth exceeded error and correctly count reverse pairs using a modified MergeSort algorithm. Optimizing your base case, refining your iteration ranges, and correctly placing your inversion counting logic will make your solution more efficient and effective. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Recursion depth exceeding in a python function for counting reverse pairs
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Fixing Recursion Depth Exceeded Error in Python Reverse Pair Counting Using MergeSort
When working with algorithms, particularly those involving recursion, programmers can sometimes run into common issues. One such problem is encountering a recursion depth exceeded error. If you're trying to implement a solution for counting reverse pairs using a variation of the MergeSort algorithm in Python, you may find yourself facing this exact issue. In this guide, we’ll take a closer look at how to address this problem effectively.
What Are Reverse Pairs?
Reverse pairs are defined as pairs (i, j) such that i < j and nums[i] > 2 * nums[j]. Counting these pairs efficiently is important for many algorithms, especially those involving sorting. The MergeSort algorithm is a popular choice to implement this due to its O(n log n) complexity, making it more efficient than simpler methods that could take O(n^2) time.
The Problem at Hand
In the code example provided, the implementation of MergeSort tries to count reverse pairs by utilizing recursion. However, it hits a wall with a maximum recursion depth exceeded error specifically on this line:
[[See Video to Reveal this Text or Code Snippet]]
This indicates that the recursion is running too deep, likely due to an incorrect base case or an infinite loop within the recursive calls.
Breaking Down the Solution
1. Adjusting the Base Case
To solve the recursion depth issue, you need to modify the stopping condition for recursion. Instead of using:
[[See Video to Reveal this Text or Code Snippet]]
You should change it to:
[[See Video to Reveal this Text or Code Snippet]]
This modification ensures that the recursion halts once there's only one element left in the current slice, thus avoiding unnecessary function calls.
2. Fix the Inversion Count Calculation
The initial approach for counting inversions in the merge function contains flaws. Consider this piece of code:
[[See Video to Reveal this Text or Code Snippet]]
The loop uses range(mid) which is too broad. It should be limited to only the relevant section from left to mid. Adjust this to:
[[See Video to Reveal this Text or Code Snippet]]
This change ensures you are only iterating over the necessary elements.
3. Additional Updates in Merge Logic
The logic that determines whether an inversion exists should be better integrated into the merge process. You can simplify your inversion counting as follows:
[[See Video to Reveal this Text or Code Snippet]]
This way, every time an element from the right slice is selected, you accurately increase your revcount for all relevant inversions.
Conclusion
By implementing the changes outlined above, you should be able to avoid the recursion depth exceeded error and correctly count reverse pairs using a modified MergeSort algorithm. Optimizing your base case, refining your iteration ranges, and correctly placing your inversion counting logic will make your solution more efficient and effective. Happy coding!