filmov
tv
Intro to API in Fusion 360 Part 7 - Creating a Script to Make a Sketch Profile #Fusion360 #API
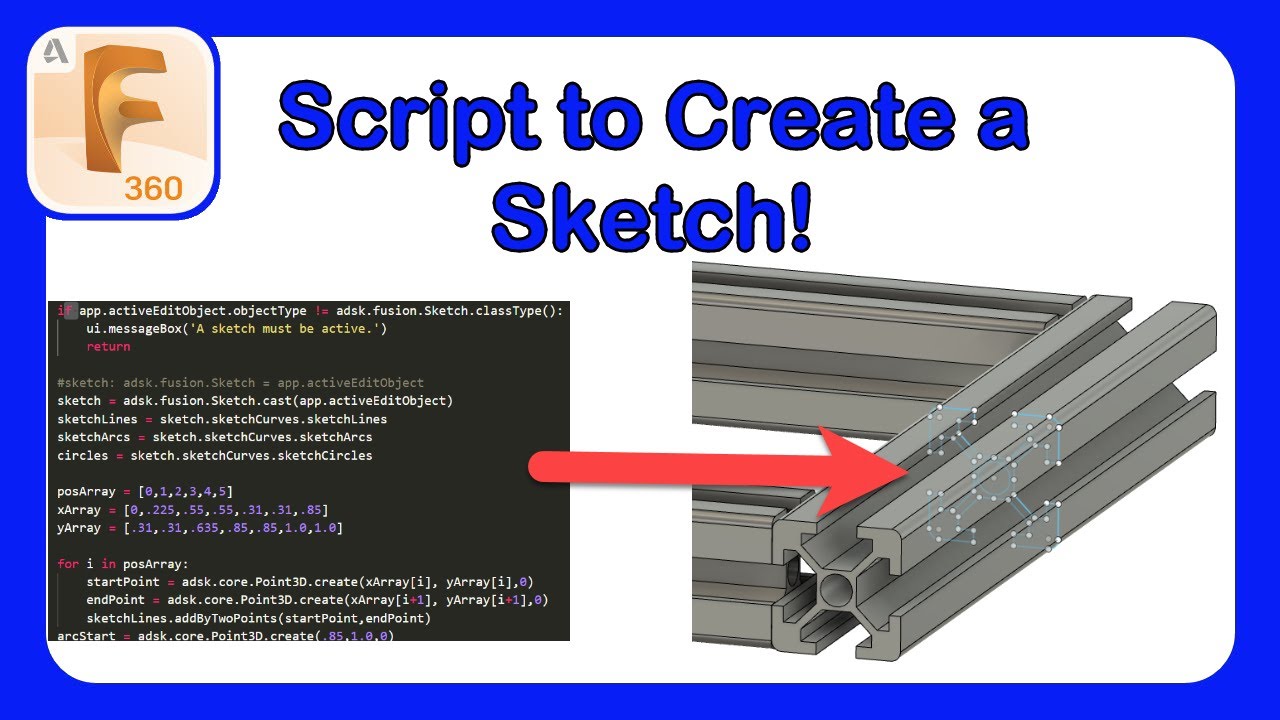
Показать описание
In this video we will continue to learn the Fusion 360 API using Python by creating a script that will make a 2020Aluminum extrusion profile that we can use for an extrude or sweep feature.
We will be covering the Object Model again as well as diving into some useful code like Arrays, For loops and If statements. The end result will be a script that will create a 2020 extrusion in a sketch currently being edited.
Here is the AL 2020 sketch used in the video. (note sometimes these don't work when they only contain sketches but you don't need it)
Here are some helpful links to the Fusion help file on API
And here are the chapters:
00:00 Intro
00:15 2020 Profile Review
04:31 Getting Started With A New Script
05:14 Reviewing the default Script
07:06 Writing a Script to Create a Sketch
22:18 Writing a Script to add Lines and Rectangles
31:32 Writing a Script to access to a current edit sketch
42:45 Writing a new Script to create a 2020 Extrusion Profile
01:21:44 Recap
We will be covering the Object Model again as well as diving into some useful code like Arrays, For loops and If statements. The end result will be a script that will create a 2020 extrusion in a sketch currently being edited.
Here is the AL 2020 sketch used in the video. (note sometimes these don't work when they only contain sketches but you don't need it)
Here are some helpful links to the Fusion help file on API
And here are the chapters:
00:00 Intro
00:15 2020 Profile Review
04:31 Getting Started With A New Script
05:14 Reviewing the default Script
07:06 Writing a Script to Create a Sketch
22:18 Writing a Script to add Lines and Rectangles
31:32 Writing a Script to access to a current edit sketch
42:45 Writing a new Script to create a 2020 Extrusion Profile
01:21:44 Recap
Intro to API in Fusion 360 Part 1 - Variables Objects and Classes #Fusion360API #Python
Intro to API in Fusion 360 Part 7 - Creating a Script to Make a Sketch Profile #Fusion360 #API
Intro to API in Fusion 360 Part 2 - Getting A User Parameters Info with a Script #Fusion360 #Python
Intro to API in Fusion 360 Part 8 - Making a Part Configurator from CSV Data #Fusion360API #Python
Intro to API in Fusion 360 Part 11 - Control a User Parameters with a Slider Dialog #fusion360 #api
Intro to API in Fusion 360 Part 3 - Creating a New User Parameter with Code #Fusion360API #Python
Intro to API in Fusion 360 Part 10 - Creating Custom Functions and Passing Variables #Fusion360
Fusion 360 API: Course Introduction
Use JWT token to call Oracle Fusion REST API in Oracle Integration 3, without IDCS, OIC 3
Intro to API in Fusion 360 Part 5 - Turning User Input into a New Parameter #Fusion360API #Python
Intro to API in Fusion 360 Part 6 - Create a User Parameter Template Script #Fusion360API #Python
Forge and Fusion 360 Hackathon - Intro to Fusion 360 API
Getting Started with Python Scripting and the Fusion API
Intro to API in Fusion 360 Part 4 - Getting User Input and Formatting It #Fusion360API #Python
Intro to API in Fusion 360 Part 9 - How to Handle Message and Input Box Events and Optional Argument
Using ChatGPT and Fusion 360 API to Create a Script | How To Make A Script for Fusion with GPT3.5
Get started with Fusion 360 API
Fusion 360 Online Hackathon Session 2: Introduction to the API
Fusion 360 API: Creating Scripts
Fusion 360 API: Command Definitions
Fusion 360 API: Parameters
Fusion 360: Et l'API?
Autodesk Fusion 360 API
Fusion 360 API: User Selections
Комментарии