filmov
tv
Javascript Quiz Time | #quiz #quiztime #javascript #javaprogramming #codinglife #javascriptinterview
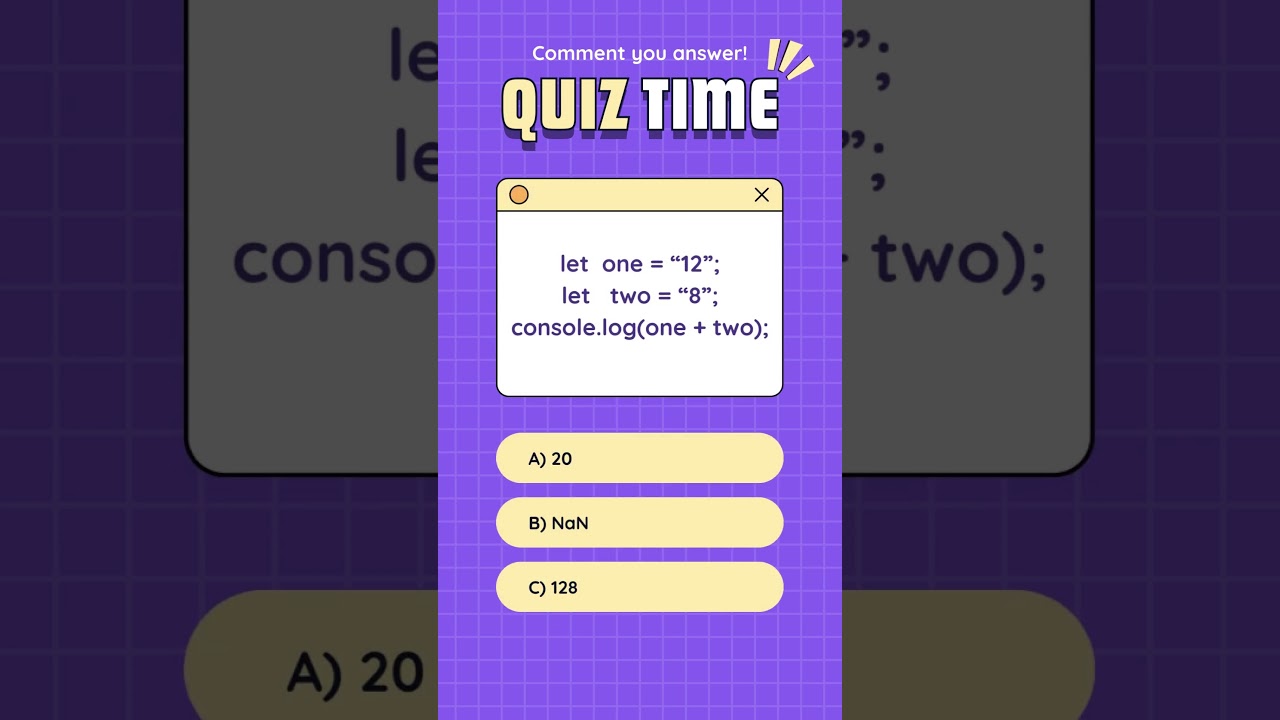
Показать описание
Introduction
JavaScript is one of the most widely-used programming languages in the world, primarily known for its role in web development. Its evolution from a simple scripting language to a powerful, versatile tool has transformed the way we interact with the web. This essay will explore the history, core features, and modern applications of JavaScript, providing an in-depth understanding of its significance in the tech industry.
History of JavaScript
The Birth of JavaScript
JavaScript was created in 1995 by Brendan Eich while working at Netscape Communications.
Initially, it was developed in just 10 days and was called Mocha, later renamed to LiveScript, and finally JavaScript.
JavaScript was designed to be a lightweight scripting language that could be used alongside Java, providing interactivity to web pages.
Early Adoption and Standardization
JavaScript was quickly adopted by web developers due to its ability to enhance user interaction on web pages.
Microsoft developed its own version, JScript, for Internet Explorer, leading to compatibility issues.
To standardize the language, ECMAScript was developed by ECMA International in 1997, based on JavaScript.
Evolution Through the Years
ECMAScript 3 (1999) brought important features like regular expressions and better string handling.
ECMAScript 5 (2009) introduced strict mode, JSON support, and more robust error handling.
ECMAScript 6 (2015), also known as ES6 or ECMAScript 2015, was a landmark update, bringing modern features like arrow functions, classes, and modules.
Core Concepts and Features of JavaScript
JavaScript Syntax
JavaScript uses C-style syntax, making it familiar to many developers.
Variables can be declared using var, let, and const, each with different scoping rules.
JavaScript supports dynamic typing, where variables can hold any data type and types can change at runtime.
Data Types
Primitive data types include Number, String, Boolean, Undefined, Null, Symbol, and BigInt.
Non-primitive types include objects, arrays, and functions.
Functions and Closures
Functions are first-class objects in JavaScript, meaning they can be stored in variables, passed as arguments, and returned from other functions.
Closures allow functions to access variables from their parent scope, even after the parent function has completed execution.
Prototypal Inheritance
JavaScript uses prototypal inheritance, where objects can inherit properties and methods from a prototype object.
This differs from classical inheritance found in languages like Java or C++, where classes inherit from other classes.
Asynchronous Programming
JavaScript’s single-threaded nature led to the development of asynchronous programming techniques.
Callbacks, Promises, and the async/await syntax are key features for handling asynchronous operations, such as API calls or file I/O.
Event Loop and Concurrency
JavaScript's event loop is a critical concept, allowing it to handle asynchronous operations in a non-blocking manner.
The event loop manages the execution of code, event handling, and executing queued tasks.
JavaScript in Web Development
Client-Side JavaScript
JavaScript is primarily known for its use on the client side, running in the browser to enhance user interfaces.
Popular use cases include form validation, interactive elements (e.g., sliders, modals), and dynamic content loading.
JavaScript Frameworks and Libraries
JavaScript's ecosystem includes numerous frameworks and libraries that simplify and enhance development.
jQuery was one of the earliest libraries to gain popularity, simplifying DOM manipulation and AJAX requests.
React (by Facebook) focuses on building UI components with a virtual DOM for efficient rendering.
Angular (by Google) is a full-fledged MVC framework offering two-way data binding and dependency injection.
It provides a non-blocking, event-driven architecture, making it ideal for building scalable network applications.
APIs and AJAX
JavaScript is essential for making asynchronous HTTP requests to servers using technologies like AJAX (Asynchronous JavaScript and XML).
Fetch API and Axios have become the standard for making HTTP requests, replacing the older XMLHttpRequest.
RESTful APIs and GraphQL have become popular choices for interacting with back-end services.
Modern JavaScript Development Practices
Modular JavaScript
JavaScript is one of the most widely-used programming languages in the world, primarily known for its role in web development. Its evolution from a simple scripting language to a powerful, versatile tool has transformed the way we interact with the web. This essay will explore the history, core features, and modern applications of JavaScript, providing an in-depth understanding of its significance in the tech industry.
History of JavaScript
The Birth of JavaScript
JavaScript was created in 1995 by Brendan Eich while working at Netscape Communications.
Initially, it was developed in just 10 days and was called Mocha, later renamed to LiveScript, and finally JavaScript.
JavaScript was designed to be a lightweight scripting language that could be used alongside Java, providing interactivity to web pages.
Early Adoption and Standardization
JavaScript was quickly adopted by web developers due to its ability to enhance user interaction on web pages.
Microsoft developed its own version, JScript, for Internet Explorer, leading to compatibility issues.
To standardize the language, ECMAScript was developed by ECMA International in 1997, based on JavaScript.
Evolution Through the Years
ECMAScript 3 (1999) brought important features like regular expressions and better string handling.
ECMAScript 5 (2009) introduced strict mode, JSON support, and more robust error handling.
ECMAScript 6 (2015), also known as ES6 or ECMAScript 2015, was a landmark update, bringing modern features like arrow functions, classes, and modules.
Core Concepts and Features of JavaScript
JavaScript Syntax
JavaScript uses C-style syntax, making it familiar to many developers.
Variables can be declared using var, let, and const, each with different scoping rules.
JavaScript supports dynamic typing, where variables can hold any data type and types can change at runtime.
Data Types
Primitive data types include Number, String, Boolean, Undefined, Null, Symbol, and BigInt.
Non-primitive types include objects, arrays, and functions.
Functions and Closures
Functions are first-class objects in JavaScript, meaning they can be stored in variables, passed as arguments, and returned from other functions.
Closures allow functions to access variables from their parent scope, even after the parent function has completed execution.
Prototypal Inheritance
JavaScript uses prototypal inheritance, where objects can inherit properties and methods from a prototype object.
This differs from classical inheritance found in languages like Java or C++, where classes inherit from other classes.
Asynchronous Programming
JavaScript’s single-threaded nature led to the development of asynchronous programming techniques.
Callbacks, Promises, and the async/await syntax are key features for handling asynchronous operations, such as API calls or file I/O.
Event Loop and Concurrency
JavaScript's event loop is a critical concept, allowing it to handle asynchronous operations in a non-blocking manner.
The event loop manages the execution of code, event handling, and executing queued tasks.
JavaScript in Web Development
Client-Side JavaScript
JavaScript is primarily known for its use on the client side, running in the browser to enhance user interfaces.
Popular use cases include form validation, interactive elements (e.g., sliders, modals), and dynamic content loading.
JavaScript Frameworks and Libraries
JavaScript's ecosystem includes numerous frameworks and libraries that simplify and enhance development.
jQuery was one of the earliest libraries to gain popularity, simplifying DOM manipulation and AJAX requests.
React (by Facebook) focuses on building UI components with a virtual DOM for efficient rendering.
Angular (by Google) is a full-fledged MVC framework offering two-way data binding and dependency injection.
It provides a non-blocking, event-driven architecture, making it ideal for building scalable network applications.
APIs and AJAX
JavaScript is essential for making asynchronous HTTP requests to servers using technologies like AJAX (Asynchronous JavaScript and XML).
Fetch API and Axios have become the standard for making HTTP requests, replacing the older XMLHttpRequest.
RESTful APIs and GraphQL have become popular choices for interacting with back-end services.
Modern JavaScript Development Practices
Modular JavaScript