filmov
tv
LeetCode 153. Find Minimum in Rotated Sorted Array (Algorithm Explained)
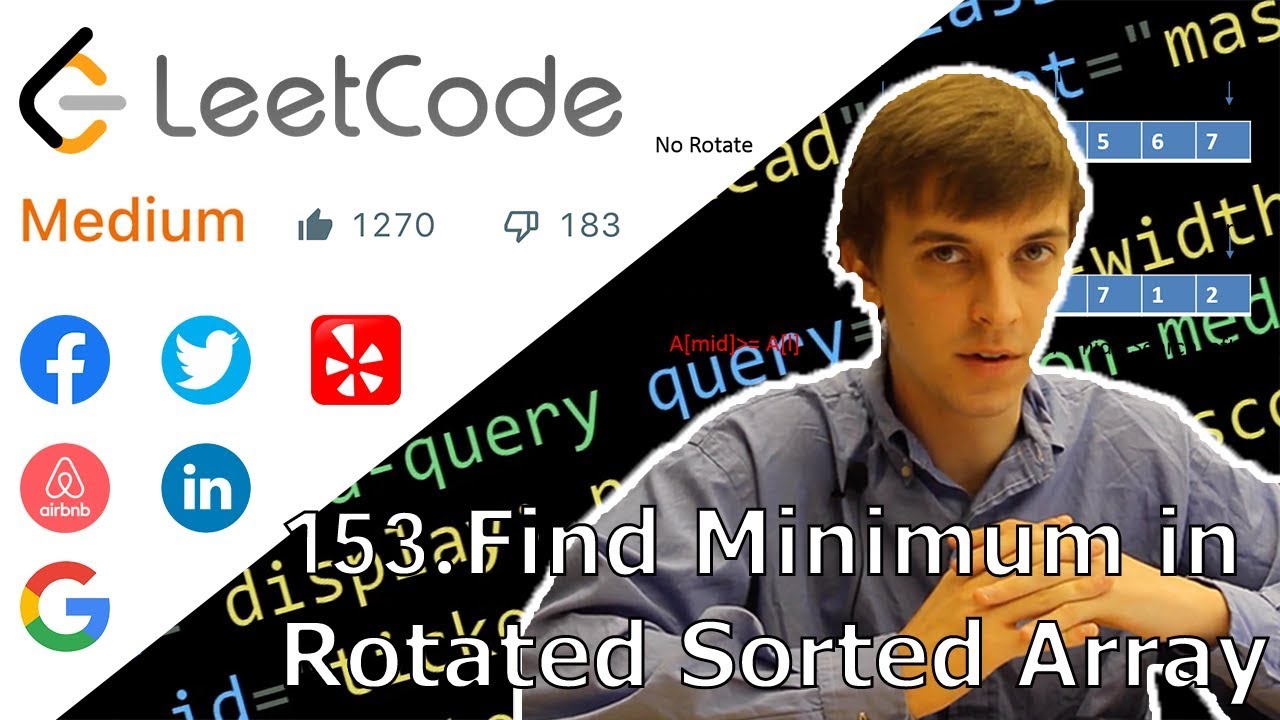
Показать описание
Preparing For Your Coding Interviews? Use These Resources
————————————————————
Other Social Media
----------------------------------------------
Show Support
------------------------------------------------------------------------------
#coding #programming #softwareengineering
Find Minimum in Rotated Sorted Array - Binary Search - Leetcode 153 - Python
LeetCode 153. Find Minimum in Rotated Sorted Array (Algorithm Explained)
Leetcode - Find Minimum in Rotated Sorted Array (Python)
Leetcode 153 - Find Minimum in Rotated Sorted Array
BS-6. Minimum in Rotated Sorted Array
Find Minimum in Rotated Sorted Array - Leetcode 153 - Binary Search (Python)
Leetcode 153 - Find Minimum in Rotated Sorted Array - Java
Microsoft Coding Interview Question - Find Minimum in Rotated Sorted Array - Leetcode 153
Solving LeetCode 153 in JavaScript (Find Minimum in Rotated Sorted Array)
Find Minimum In Rotated Sorted Array - LeetCode 153 - JavaScript
Find minimum in rotated sorted array (LeetCode 153) | Full solution with visuals | Divide & Conq...
FIND MINIMUM IN ROTATED SORTED ARRAY | LEETCODE # 153 | PYTHON BINARY SEARCH SOLUTION
Find Minimum in Rotated Sorted Array - LeetCode 153 - Binary Search
Find Minimum in Rotated Sorted Array | LeetCode 153 | C++, Java, Python3
Find Minimum in Rotated Sorted Array - LeetCode #153 with Python programming
Leetcode | 153. Find Minimum in Rotated Sorted Array | Medium | Java Solution
I Couldn't Get This Coding Question - Can You? | Find Minimum in Rotated Sorted Array - Leetcod...
[Java] Leetcode 153. Find Minimum in Rotated Sorted Array [Binary Search #1]
Find Minimum in Rotated Sorted Array| Leetcode 153 | Live coding session 🔥🔥🔥
153. Find Minimum in Rotated Sorted Array | Javascript
LeetCode 153 | Find Minimum in Rotated Sorted Array | Medium | C# Solution |
Find Minimum in Rotated Sorted Array-(Amazon, Microsoft, Morgan Stanley):Live Coding 🧑🏻💻👩🏻💻...
153. Find Minimum in Rotated Sorted Array
Leetcode 153: Find Minimum in Rotated Sorted Array
Комментарии