filmov
tv
Leetcode Solution - 1.0 Two Sum | Javascript
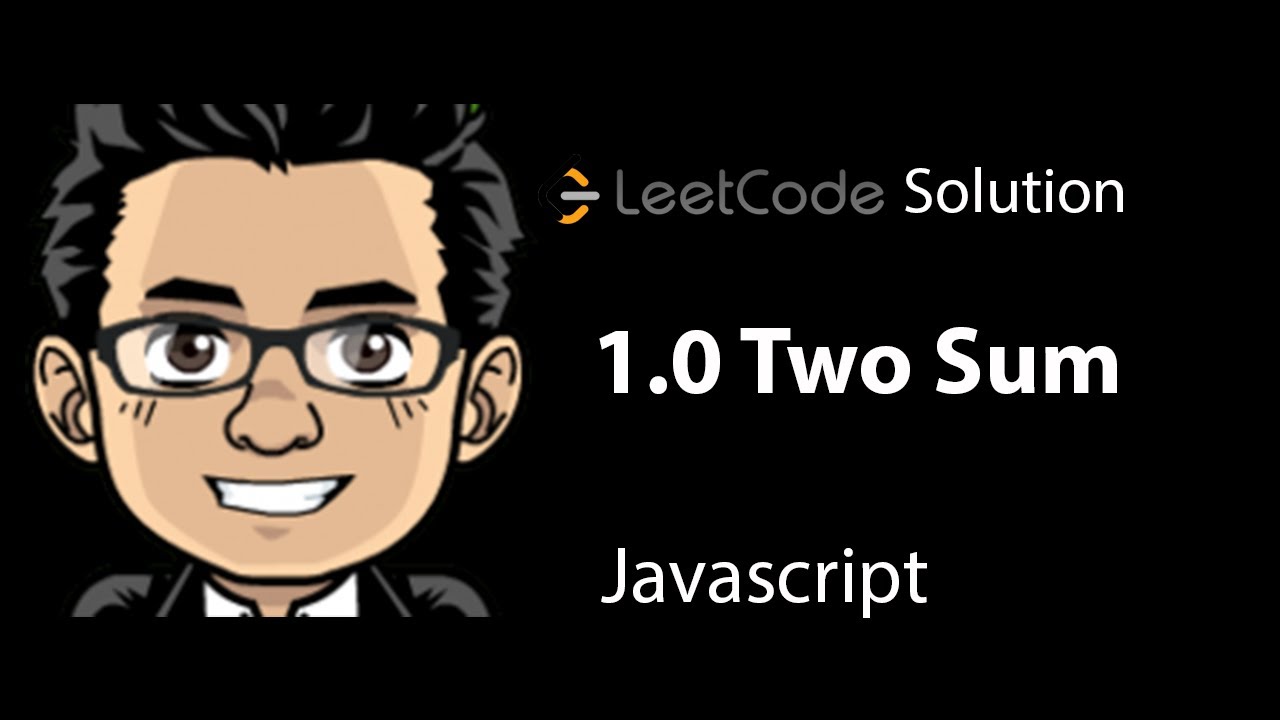
Показать описание
This is a detail Solution to the Leetcode question Two Sum written in Javascript.
Two Sum - Leetcode 1 - HashMap - Python
TWO SUM II - Amazon Coding Interview Question - Leetcode 167 - Python
LeetCode Exercise in Java Tutorial - Two Sum FAST Solution
Trapping Rain Water - Google Interview Question - Leetcode 42
LeetCode 1 - Two Sum - Java Solution 0(N) BEATS 99%
Sort an array of 0's 1's & 2's | Intuition of Algo🔥 | C++ Java Python | Brute-Bet...
Subarray Sum Equals K - Prefix Sums - Leetcode 560 - Python
Majority Element I | Brute-Better-Optimal | Moore's Voting Algorithm | Intuition 🔥|Brute to Opt...
Total Unique Ways To Make Change - Dynamic Programming ('Coin Change 2' on LeetCode)
Sum of Two Integers - Leetcode 371 - Java
Container with Most Water - Leetcode 11 - Python
Add Two Numbers - Leetcode 2 - Python
Merge Sorted Array - Leetcode 88 - Python
3Sum - Leetcode 15 - Python
Always solve a weird leetcode Problem! 🤬😭 | Programming Tips
Climbing Stairs - Dynamic Programming - Leetcode 70 - Python
Coin Change 2 - Dynamic Programming Unbounded Knapsack - Leetcode 518 - Python
Median of Two Sorted Arrays - Binary Search - Leetcode 4
Move Zeroes - Leetcode 283 - Python
Sliding Window: Best Time to Buy and Sell Stock - Leetcode 121 - Python
Find Minimum in Rotated Sorted Array - Binary Search - Leetcode 153 - Python
0/1 Knapsack problem | Dynamic Programming
Sort Colors - Quicksort Partition - Leetcode 75 - Python
Longest Common Subsequence - Dynamic Programming - Leetcode 1143
Комментарии