filmov
tv
How to Properly Convert String Values to Integers in JavaScript Arrays
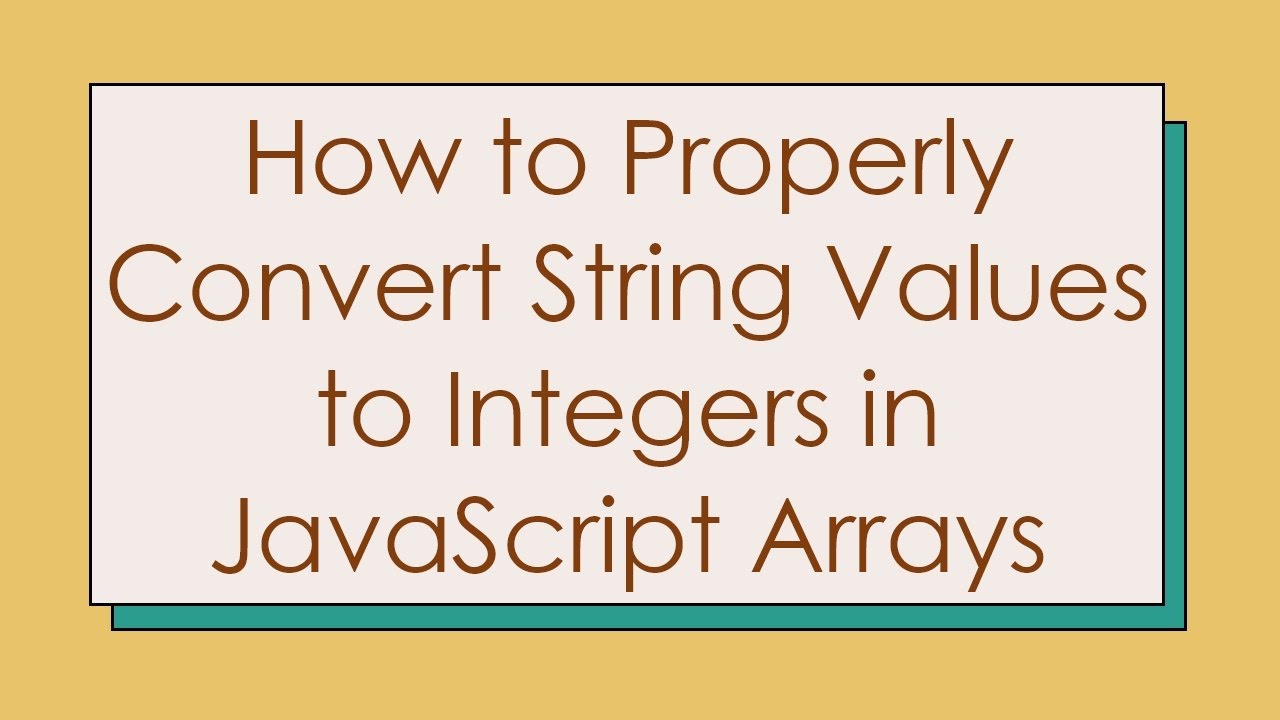
Показать описание
Discover a simple solution to convert string values to integers in JavaScript arrays. Learn how to clean up your data for better handling and processing.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: JS - add ParseInt value to array
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Properly Convert String Values to Integers in JavaScript Arrays
Working with data received from backends can sometimes feel like navigating a maze, especially when the data includes unexpected types—like strings representing numbers. If you're facing a situation where you need to convert string values to integers in an array, you've come to the right place! Let’s dive into the problem and explore a straightforward solution.
The Problem: Converting Strings to Integers
Imagine you have a JSON object containing information about cars, with their respective values stored as strings. Here's what the problematic JSON looks like:
[[See Video to Reveal this Text or Code Snippet]]
While this data is perfectly valid, your application likely expects these values as integers. When trying to convert them using a looping method, you might encounter issues where the wrong values end up in your array, or worse, duplicate entries. For example, consider the following erroneous attempt at conversion:
[[See Video to Reveal this Text or Code Snippet]]
This code snippet has fundamental issues and results in incorrect data because of how it processes the array. What we need is a streamlined approach that simplifies the conversion process.
The Solution: Using map() to Convert Values
The simplest and most effective way to convert an array of strings to numbers in JavaScript is by using the map() function. This method allows you to apply a conversion function to each element of the array, in one tidy operation! Let’s look at how this can be done.
Step-by-Step Implementation
Declare your data: Start with the original JSON data.
Use .map(Number): This will apply the Number function to each element of the array, converting strings to integers.
Here’s the revised code snippet:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
const data: This defines your initial data structure.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By using the map() method combined with the Number function, you can quickly and efficiently convert string-based numeric values to actual integers in your JavaScript arrays. This simple approach prevents the issues found in more complex looping structures, leading to cleaner and more maintainable code.
Feel free to try this out with your own data, and say goodbye to the headaches of mismanaged data types in your applications! If you have any questions or further issues, don't hesitate to ask.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: JS - add ParseInt value to array
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Properly Convert String Values to Integers in JavaScript Arrays
Working with data received from backends can sometimes feel like navigating a maze, especially when the data includes unexpected types—like strings representing numbers. If you're facing a situation where you need to convert string values to integers in an array, you've come to the right place! Let’s dive into the problem and explore a straightforward solution.
The Problem: Converting Strings to Integers
Imagine you have a JSON object containing information about cars, with their respective values stored as strings. Here's what the problematic JSON looks like:
[[See Video to Reveal this Text or Code Snippet]]
While this data is perfectly valid, your application likely expects these values as integers. When trying to convert them using a looping method, you might encounter issues where the wrong values end up in your array, or worse, duplicate entries. For example, consider the following erroneous attempt at conversion:
[[See Video to Reveal this Text or Code Snippet]]
This code snippet has fundamental issues and results in incorrect data because of how it processes the array. What we need is a streamlined approach that simplifies the conversion process.
The Solution: Using map() to Convert Values
The simplest and most effective way to convert an array of strings to numbers in JavaScript is by using the map() function. This method allows you to apply a conversion function to each element of the array, in one tidy operation! Let’s look at how this can be done.
Step-by-Step Implementation
Declare your data: Start with the original JSON data.
Use .map(Number): This will apply the Number function to each element of the array, converting strings to integers.
Here’s the revised code snippet:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
const data: This defines your initial data structure.
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By using the map() method combined with the Number function, you can quickly and efficiently convert string-based numeric values to actual integers in your JavaScript arrays. This simple approach prevents the issues found in more complex looping structures, leading to cleaner and more maintainable code.
Feel free to try this out with your own data, and say goodbye to the headaches of mismanaged data types in your applications! If you have any questions or further issues, don't hesitate to ask.