filmov
tv
Resolving AttributeError: Handling JSON Data with Python
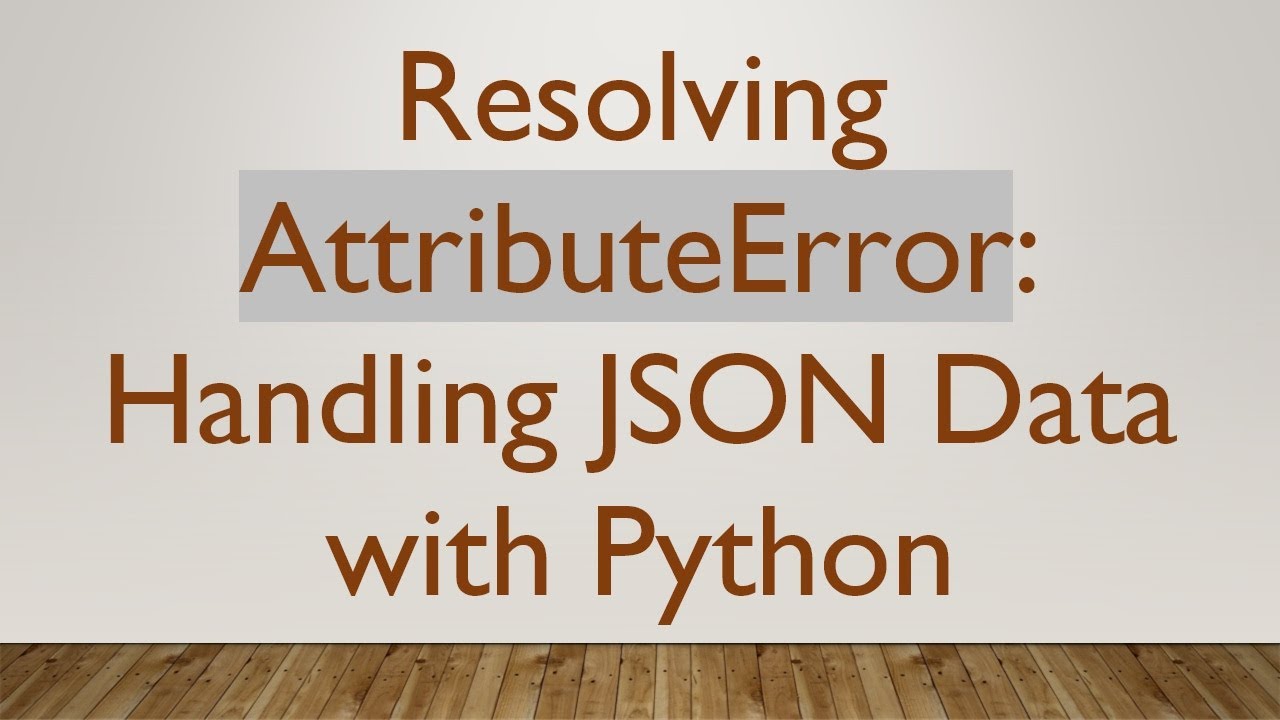
Показать описание
Encountering `AttributeError: 'list' object has no attribute 'items'` while importing JSON in Python? Learn how to fix this issue and manage your data effectively in your Django application!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: "AttributeError: 'list' object has no attribute 'items'" While importing Json
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Resolving AttributeError: Handling JSON Data with Python
When working with JSON data in Python, particularly in a Django application, you might run into an error that can halt your progress - the dreaded AttributeError: 'list' object has no attribute 'items'. This issue often surfaces when you mistakenly treat a list as a dictionary while trying to iterate through its contents. In this guide, we will explore the problem, understand why it occurs, and provide a clear and organized solution to help you avoid this error in the future.
The Problem Explained
In your code, you're trying to process a JSON file that contains a list of deleted facilities by using the .items() method on a data object that is, essentially, a list. The relevant part of your code looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
Since data_object is a list, it doesn't have the items() method which is exclusive to dictionaries. This leads to the AttributeError you encountered. This commonly occurs when JSON data is structured in a way that includes lists, as in your case where deleted facilities are gathered in a list.
Understanding the JSON Structure
To better understand the error, let’s take a look at the JSON structure you provided:
[[See Video to Reveal this Text or Code Snippet]]
Note that the deleted key contains a list of UUIDs, hence when you try to call .items() on it, the error arises.
The Solution
To resolve the AttributeError, you need to modify your code to appropriately handle the list data type. Here’s how you can correctly implement the iteration:
Revised Code
Update the loop where you're accessing the deleted field in your JSON data like so:
[[See Video to Reveal this Text or Code Snippet]]
Key Changes Made:
Remove the items() method: When iterating over a list, you only need to directly loop through the list items.
Direct iteration: The revised line simply loops through each item in data_object, which correctly aligns with the structure of your JSON.
Conclusion
By understanding the structure of your JSON data and correctly looping through it, you can effectively avoid the AttributeError: 'list' object has no attribute 'items'. Remember, in Python, lists and dictionaries behave differently, and recognizing these distinctions is essential for parsing JSON data efficiently. Implementing the solution above will enable you to correctly update the reported status of your facilities without running into errors. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: "AttributeError: 'list' object has no attribute 'items'" While importing Json
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Resolving AttributeError: Handling JSON Data with Python
When working with JSON data in Python, particularly in a Django application, you might run into an error that can halt your progress - the dreaded AttributeError: 'list' object has no attribute 'items'. This issue often surfaces when you mistakenly treat a list as a dictionary while trying to iterate through its contents. In this guide, we will explore the problem, understand why it occurs, and provide a clear and organized solution to help you avoid this error in the future.
The Problem Explained
In your code, you're trying to process a JSON file that contains a list of deleted facilities by using the .items() method on a data object that is, essentially, a list. The relevant part of your code looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
Since data_object is a list, it doesn't have the items() method which is exclusive to dictionaries. This leads to the AttributeError you encountered. This commonly occurs when JSON data is structured in a way that includes lists, as in your case where deleted facilities are gathered in a list.
Understanding the JSON Structure
To better understand the error, let’s take a look at the JSON structure you provided:
[[See Video to Reveal this Text or Code Snippet]]
Note that the deleted key contains a list of UUIDs, hence when you try to call .items() on it, the error arises.
The Solution
To resolve the AttributeError, you need to modify your code to appropriately handle the list data type. Here’s how you can correctly implement the iteration:
Revised Code
Update the loop where you're accessing the deleted field in your JSON data like so:
[[See Video to Reveal this Text or Code Snippet]]
Key Changes Made:
Remove the items() method: When iterating over a list, you only need to directly loop through the list items.
Direct iteration: The revised line simply loops through each item in data_object, which correctly aligns with the structure of your JSON.
Conclusion
By understanding the structure of your JSON data and correctly looping through it, you can effectively avoid the AttributeError: 'list' object has no attribute 'items'. Remember, in Python, lists and dictionaries behave differently, and recognizing these distinctions is essential for parsing JSON data efficiently. Implementing the solution above will enable you to correctly update the reported status of your facilities without running into errors. Happy coding!