filmov
tv
Python Tuple for Data-Driven Engineering
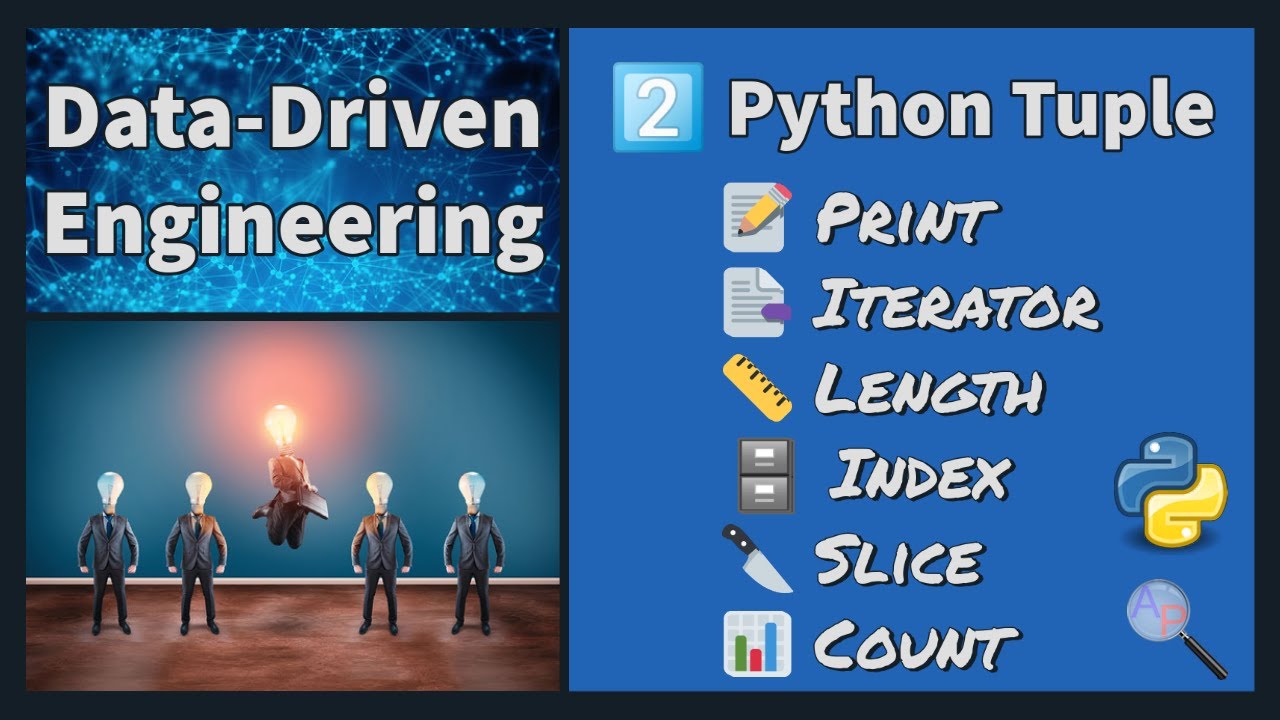
Показать описание
A tuple is a data structure in Python that is similar to a list, but it is immutable, meaning that it cannot be changed once created. This makes tuples more efficient and less prone to errors, because they cannot be modified by mistake. A tuple is one of four types that can store multiple items as a single variable. To create a tuple in Python, you use parentheses and separate the elements with commas.
my_tuple = (1, 2, 3)
You can access the elements of a tuple using indexing, just like with a list.
print(my_tuple[0]) # prints 1
You can also use slicing to access a range of elements in a tuple.
print(my_tuple[1:]) # prints (2, 3)
Tuples also support many of the same methods as lists, such as len() to get the length of the tuple, and count() to count the number of occurrences of a particular element. Overall, tuples are a useful data structure in Python because they allow you to store and manipulate data in a way that is efficient and less prone to errors.
1️⃣ Python Basics
Data-driven engineering relies on information, often stored in the form of characters (strings) and numbers (integers and floating point numbers). It is essential to import, export, and get data into the correct form so that information can be extracted. This series includes an introduction to Python Basics as foundational elements.
2️⃣ Python Tuple
Tuple (e.g. (i,x,e)) is immutable (does not change) as an efficient storage mechanism for constant sets of values.
3️⃣ Python List
List (e.g. [i,x,e]) is a mutable set of values where it is possible to add elements, remove elements, sort.
4️⃣ Python Set
Set (e.g. {i,x,e}) is a data structure that is similar to list but not sorted and has no duplicate values.
5️⃣ Python Dictionary
Dictionary (e.g. {'i':i,'x':x,'e':e}) is a data structure with a reference value based on key.
6️⃣ NumPy
NumPy expands upon the basic Python functions to create an array. Matrix and vector operations are designed as a foundation for numerical calculations.
7️⃣ Pandas
Pandas reads, cleanses, calculates, rearranges, and exports data. It is a library for working with data with common high-level functions that simplify the processing steps of analytics and informatics.
my_tuple = (1, 2, 3)
You can access the elements of a tuple using indexing, just like with a list.
print(my_tuple[0]) # prints 1
You can also use slicing to access a range of elements in a tuple.
print(my_tuple[1:]) # prints (2, 3)
Tuples also support many of the same methods as lists, such as len() to get the length of the tuple, and count() to count the number of occurrences of a particular element. Overall, tuples are a useful data structure in Python because they allow you to store and manipulate data in a way that is efficient and less prone to errors.
1️⃣ Python Basics
Data-driven engineering relies on information, often stored in the form of characters (strings) and numbers (integers and floating point numbers). It is essential to import, export, and get data into the correct form so that information can be extracted. This series includes an introduction to Python Basics as foundational elements.
2️⃣ Python Tuple
Tuple (e.g. (i,x,e)) is immutable (does not change) as an efficient storage mechanism for constant sets of values.
3️⃣ Python List
List (e.g. [i,x,e]) is a mutable set of values where it is possible to add elements, remove elements, sort.
4️⃣ Python Set
Set (e.g. {i,x,e}) is a data structure that is similar to list but not sorted and has no duplicate values.
5️⃣ Python Dictionary
Dictionary (e.g. {'i':i,'x':x,'e':e}) is a data structure with a reference value based on key.
6️⃣ NumPy
NumPy expands upon the basic Python functions to create an array. Matrix and vector operations are designed as a foundation for numerical calculations.
7️⃣ Pandas
Pandas reads, cleanses, calculates, rearranges, and exports data. It is a library for working with data with common high-level functions that simplify the processing steps of analytics and informatics.
Комментарии