filmov
tv
Is there Garbage Collection in C and C++?
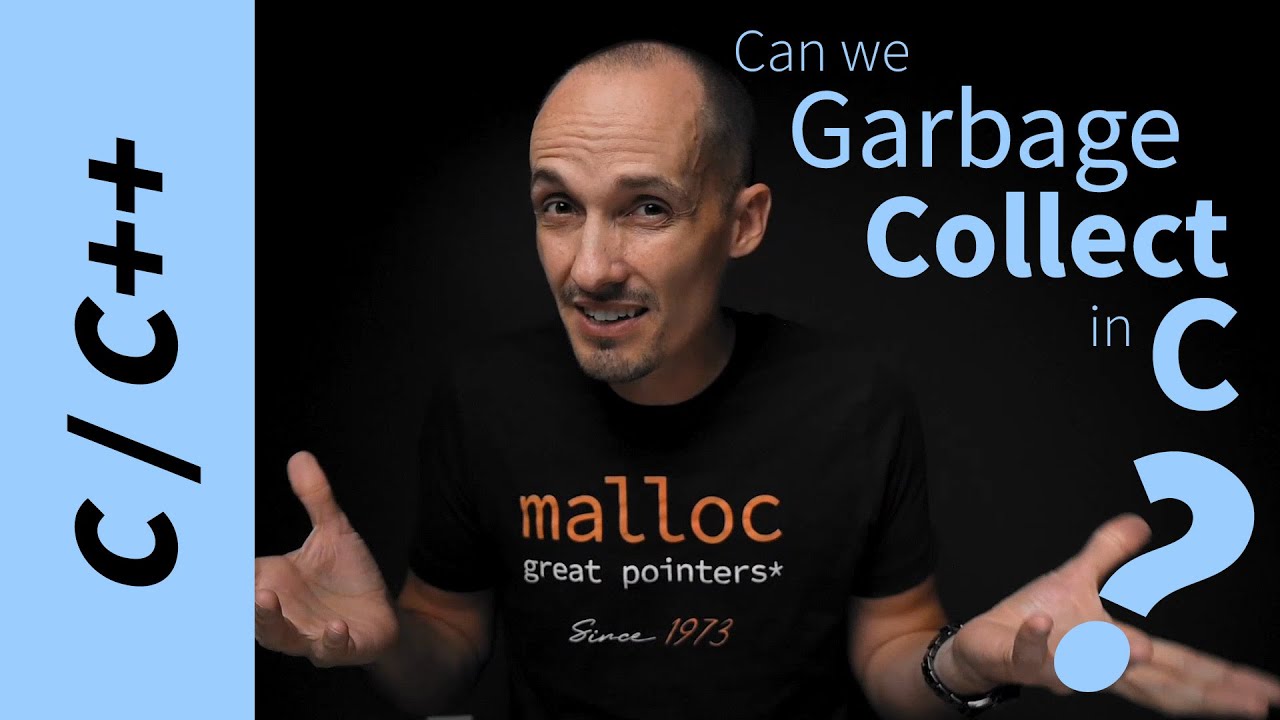
Показать описание
---
Is there Garbage Collection in C and C++? // You aren't the first person to wonder about automatic memory cleanup in C. Why don't we have garbage collectors like they do in other languages? Let's talk about it.
Related Videos:
***
Welcome! I post videos that help you learn to program and become a more confident software developer. I cover beginner-to-advanced systems topics ranging from network programming, threads, processes, operating systems, embedded systems and others. My goal is to help you get under-the-hood and better understand how computers work and how you can use them to become stronger students and more capable professional developers.
About me: I'm a computer scientist, electrical engineer, researcher, and teacher. I specialize in embedded systems, mobile computing, sensor networks, and the Internet of Things. I teach systems and networking courses at Clemson University, where I also lead the PERSIST research lab.
More about me and what I do:
To Support the Channel:
+ like, subscribe, spread the word
Is there Garbage Collection in C and C++? // You aren't the first person to wonder about automatic memory cleanup in C. Why don't we have garbage collectors like they do in other languages? Let's talk about it.
Related Videos:
***
Welcome! I post videos that help you learn to program and become a more confident software developer. I cover beginner-to-advanced systems topics ranging from network programming, threads, processes, operating systems, embedded systems and others. My goal is to help you get under-the-hood and better understand how computers work and how you can use them to become stronger students and more capable professional developers.
About me: I'm a computer scientist, electrical engineer, researcher, and teacher. I specialize in embedded systems, mobile computing, sensor networks, and the Internet of Things. I teach systems and networking courses at Clemson University, where I also lead the PERSIST research lab.
More about me and what I do:
To Support the Channel:
+ like, subscribe, spread the word
Комментарии