filmov
tv
Handling Overflow and Underflow in JavaScript Stack Implementation
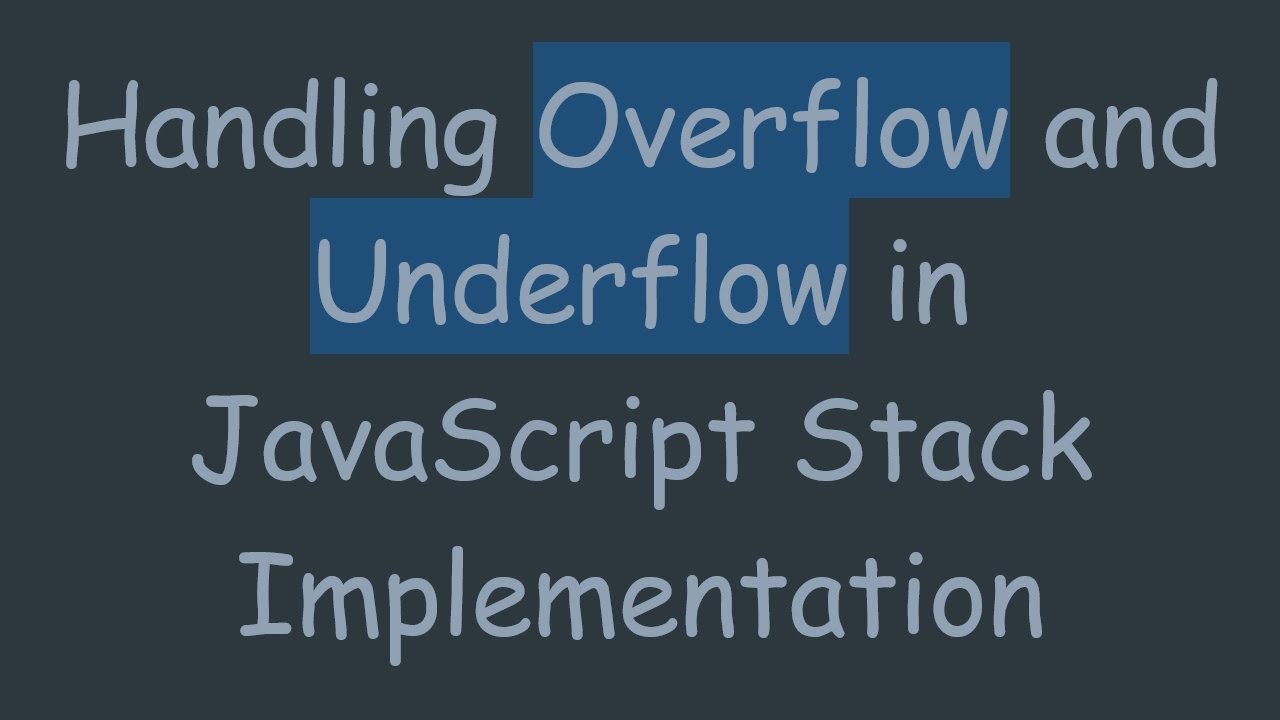
Показать описание
Learn how to effectively implement error handling for `overflow` and `underflow` in your JavaScript stack class. This guide provides a clear solution and code examples for managing stack limits.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Overflow & Underflow
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Handling Overflow and Underflow in JavaScript Stack Implementation
Creating a stack in JavaScript is a common task in programming, but what happens when we exceed the limits of our data structure? Overflow and underflow errors can lead to unexpected behavior if not properly managed. This guide explores how to handle such situations when building a stack class in JavaScript.
Understanding the Problem
In a stack data structure, we can push and pop items. However, without proper checks, we might run into issues:
Overflow occurs when we try to push an item onto the stack after it has reached its maximum capacity. In a real-world scenario, this can lead to performance issues or application crashes if not properly managed.
Underflow happens when we attempt to pop an item from an empty stack. This situation can leave developers puzzled and lead to errors in program execution.
In your current setup, you’re looking to throw errors when these conditions are met—specifically, when the maximum call stack is reached and when the stack is empty. Let's dive into how to effectively implement these checks in your JavaScript stack class.
Modifying the Stack Class
To address overflow and underflow in your Stack class, we need to modify the push and pop methods. The following sections break down these adjustments step-by-step.
Step 1: Define Your Stack Class
Begin by defining your Stack class and initializing an empty array to hold your stack items.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Implement the push Method
The push method should check if the current stack size has reached Number.MAX_SAFE_INTEGER, which is the largest number JavaScript can handle. If this limit is reached, we throw an error.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Implement the pop Method
The pop method needs a check to ensure that the stack isn't empty. If attempting to pop an item when the stack is empty, we should throw an underflow error.
[[See Video to Reveal this Text or Code Snippet]]
Finalizing the Implementation
In addition to these methods, it's a good idea to implement some utility methods like isEmpty and peek, which offer additional functionality and convenience.
[[See Video to Reveal this Text or Code Snippet]]
Complete Stack Class Implementation
Putting it all together, your modified stack class will look like this:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By implementing error handling for overflow and underflow in your stack class, you ensure your application can handle these situations gracefully. This approach improves the robustness of your code and provides clearer feedback when issues arise.
Remember, as with any implementation, testing is crucial. Make sure to test your stack under various conditions to confirm that your error handling works as expected.
If you have any questions or need further assistance with your stack implementation, feel free to reach out!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Overflow & Underflow
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Handling Overflow and Underflow in JavaScript Stack Implementation
Creating a stack in JavaScript is a common task in programming, but what happens when we exceed the limits of our data structure? Overflow and underflow errors can lead to unexpected behavior if not properly managed. This guide explores how to handle such situations when building a stack class in JavaScript.
Understanding the Problem
In a stack data structure, we can push and pop items. However, without proper checks, we might run into issues:
Overflow occurs when we try to push an item onto the stack after it has reached its maximum capacity. In a real-world scenario, this can lead to performance issues or application crashes if not properly managed.
Underflow happens when we attempt to pop an item from an empty stack. This situation can leave developers puzzled and lead to errors in program execution.
In your current setup, you’re looking to throw errors when these conditions are met—specifically, when the maximum call stack is reached and when the stack is empty. Let's dive into how to effectively implement these checks in your JavaScript stack class.
Modifying the Stack Class
To address overflow and underflow in your Stack class, we need to modify the push and pop methods. The following sections break down these adjustments step-by-step.
Step 1: Define Your Stack Class
Begin by defining your Stack class and initializing an empty array to hold your stack items.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Implement the push Method
The push method should check if the current stack size has reached Number.MAX_SAFE_INTEGER, which is the largest number JavaScript can handle. If this limit is reached, we throw an error.
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Implement the pop Method
The pop method needs a check to ensure that the stack isn't empty. If attempting to pop an item when the stack is empty, we should throw an underflow error.
[[See Video to Reveal this Text or Code Snippet]]
Finalizing the Implementation
In addition to these methods, it's a good idea to implement some utility methods like isEmpty and peek, which offer additional functionality and convenience.
[[See Video to Reveal this Text or Code Snippet]]
Complete Stack Class Implementation
Putting it all together, your modified stack class will look like this:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
By implementing error handling for overflow and underflow in your stack class, you ensure your application can handle these situations gracefully. This approach improves the robustness of your code and provides clearer feedback when issues arise.
Remember, as with any implementation, testing is crucial. Make sure to test your stack under various conditions to confirm that your error handling works as expected.
If you have any questions or need further assistance with your stack implementation, feel free to reach out!