filmov
tv
Using a Double Sort on an Array of Objects
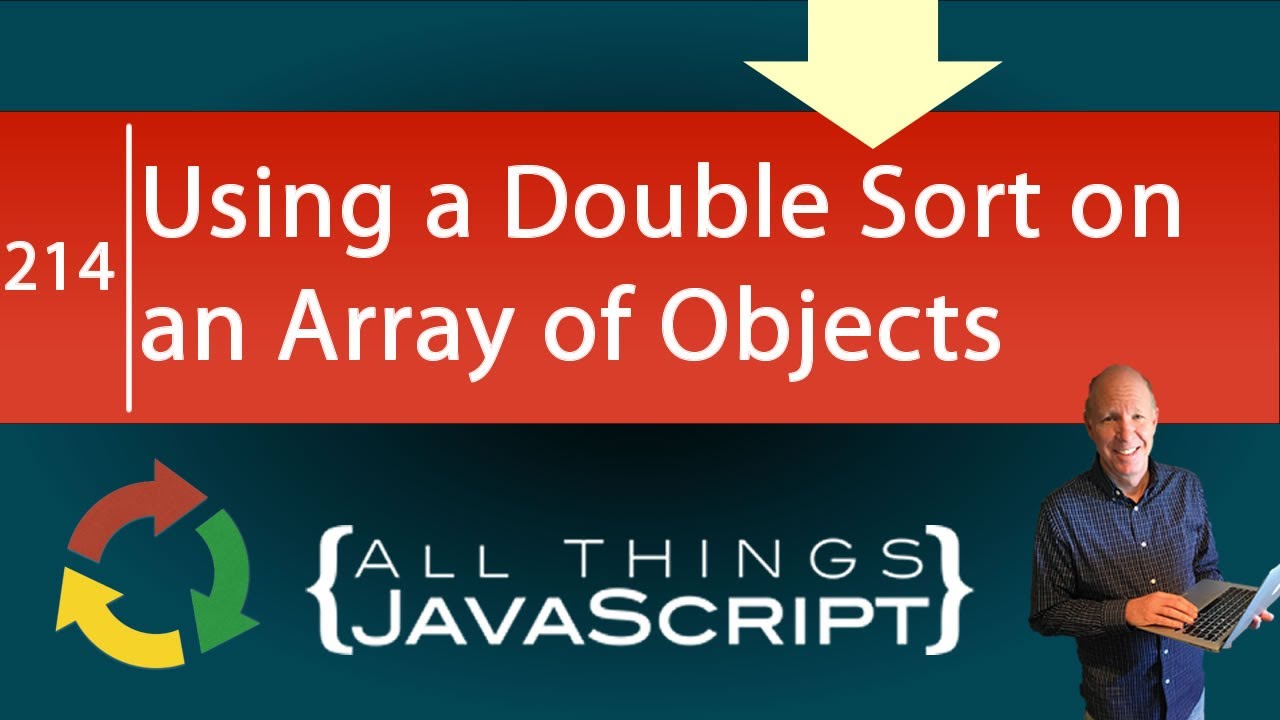
Показать описание
In this tutorial we are going to tackle another JavaScript problem. And in this problem I want to use the sort method of arrays. So we are going to look at how we can sort objects in an array, but we will be using a primary sort and a secondary sort.
Would you like to help keep this channel going?
Tutorials referred to in this video:
For more resources on JavaScript:
#javascript #AllThingsJavaScriptLLC
Would you like to help keep this channel going?
Tutorials referred to in this video:
For more resources on JavaScript:
#javascript #AllThingsJavaScriptLLC
Using a Double Sort on an Array of Objects
Double Selection Sort
Sort by two or multiple fields using java 8
Merge 2 Sorted Lists - A Fundamental Merge Sort Subroutine ('Merge Two Sorted Lists' on Le...
Double Insertion Sort
Mathematica: Double sorting by two criterions using Sort
Root Tutorial: Sort an Array (Vector) of Double and Quickly and Easily
Two Excel Dynamic Array Functions: UNIQUE and SORT
The Cleveland Guardians starters HAVE TO eat up innings in today's double-header vs. the Diamon...
Tsupetot Tutorial #1 - Stocks Sorting Technique featuring Double Sort
Sleep sort is only for two use cases
Append Two Number Columns and Sort. Excel Magic Trick 1682.
75. Sort Colors | LeetCode Medium | Python Solution | Array, Two Pointers, Sorting
Sort Numbers By Using Two Stacks | Interview Question 1
SORT Function in Excel-Two Examples Sort Data Automatically and Combine the Sort and Unique Function
How to Sort and Synchronize two list together and align two set of Data in Microsoft Excel
Sorting Arrays is SUPER Easy in PHP Using These Two Methods - sort() and rsort() Explained #shorts
Como viskestykkeholder 60 cm, Sort DOUBLE
How to sort multiple Excel fields + functions & double space a table...
Merge Two Arrays and sort them in Java
Using the Linux sort command with two sort keys
Sort two arrays using Merge Sort
Complexity of Double Selection Sort
How to sort by two fields in Java using Comparable interface
Комментарии