filmov
tv
Coding Interview Tutorial 24: Longest Substring without Repeating Characters [LeetCode]
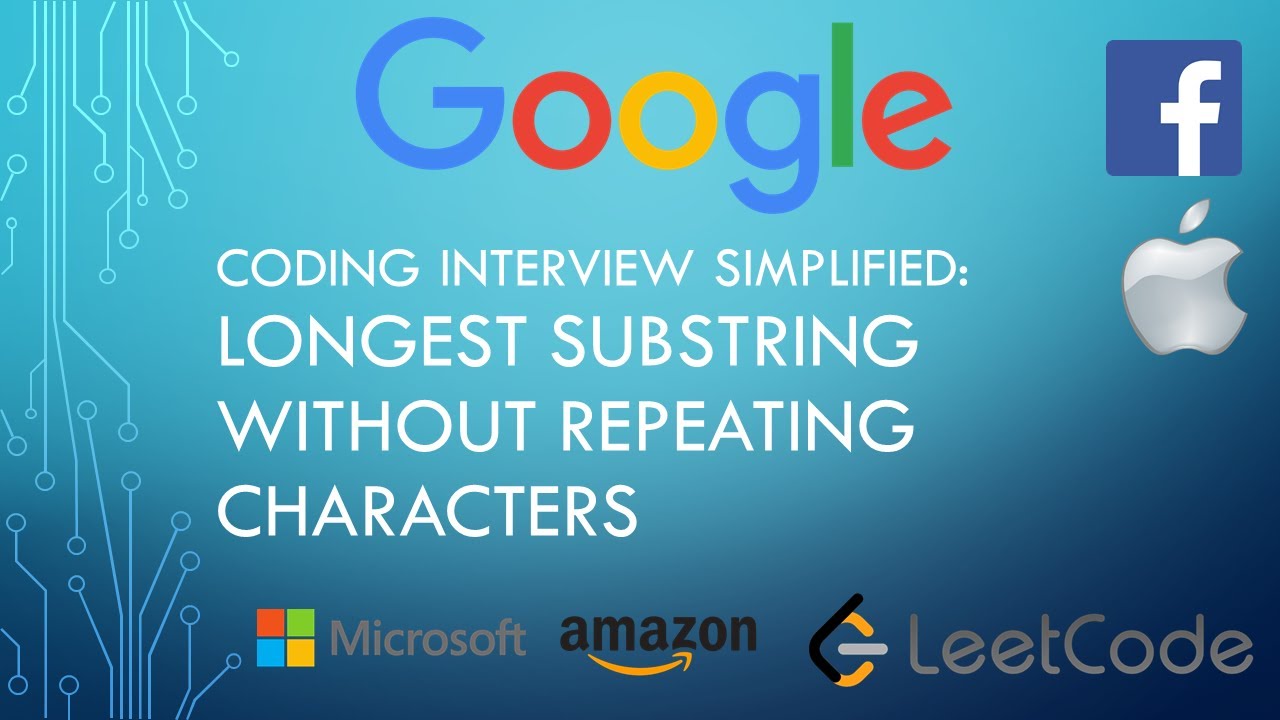
Показать описание
Learn how to find the length of the longest substring without repeating characters in O(N) time, where N is the length of the input string.
This is an important programming interview question, and we use the LeetCode platform to solve this problem.
This is an important programming interview question, and we use the LeetCode platform to solve this problem.
Coding Interview Tutorial 100 - Longest Common Prefix [LeetCode]
Top 5 Algorithms for Coding Interviews
10 Common Coding Interview Problems - Solved!
Winning Google Kickstart Round A 2020 + Facecam
Google Coding Interview Question - Longest String Chain
CODING INTERVIEW STEP BY STEP GUIDE | FOLLOW THIS ALGORITHM FOR SUCCESS IN A TECHNICAL INTERVIEW
Code With Me: 24 FAANG Interview Questions
Longest Substring Without Repeating Characters | LeetCode 3 | Coding Interview Tutorial
Coding Interview Preparation: What To Do 24 Hours Before?
Coding Interview Tutorial 25: Minimum Window Substring [LeetCode]
Programming Interview Question: Longest Increasing Subsequence nlogn
Coding Interview Fundamentals: Post-Order Traversal
Interview Question: Longest Common Substring
IQ TEST
I solved 541 Leetcode problems. But you need only 150.
I solved 541 Leetcode problems #leetcode #codinginterview
LeetCode Longest Repeating Character Replacement Solution Explained - Java
4 mistakes to avoid during coding interview preparation
How to: Work at Google — Example Coding/Engineering Interview
24 hours LEETCODE challenge! | No Sleep 😴 | Solving @NishantChahar11's SDE sheet
Programming Interview: Longest Common Subsequence Dynamic Programming
PrepBytes | Popular Coding Interview Question | Length of Longest Palindrome
Longest Substring with K Unique Characters | Programming Tutorials
Leetcode 128. Longest Consecutive Sequence | Mock Interview
Комментарии