filmov
tv
Mastering JavaScript Regex: Validating Folder Names with Ease
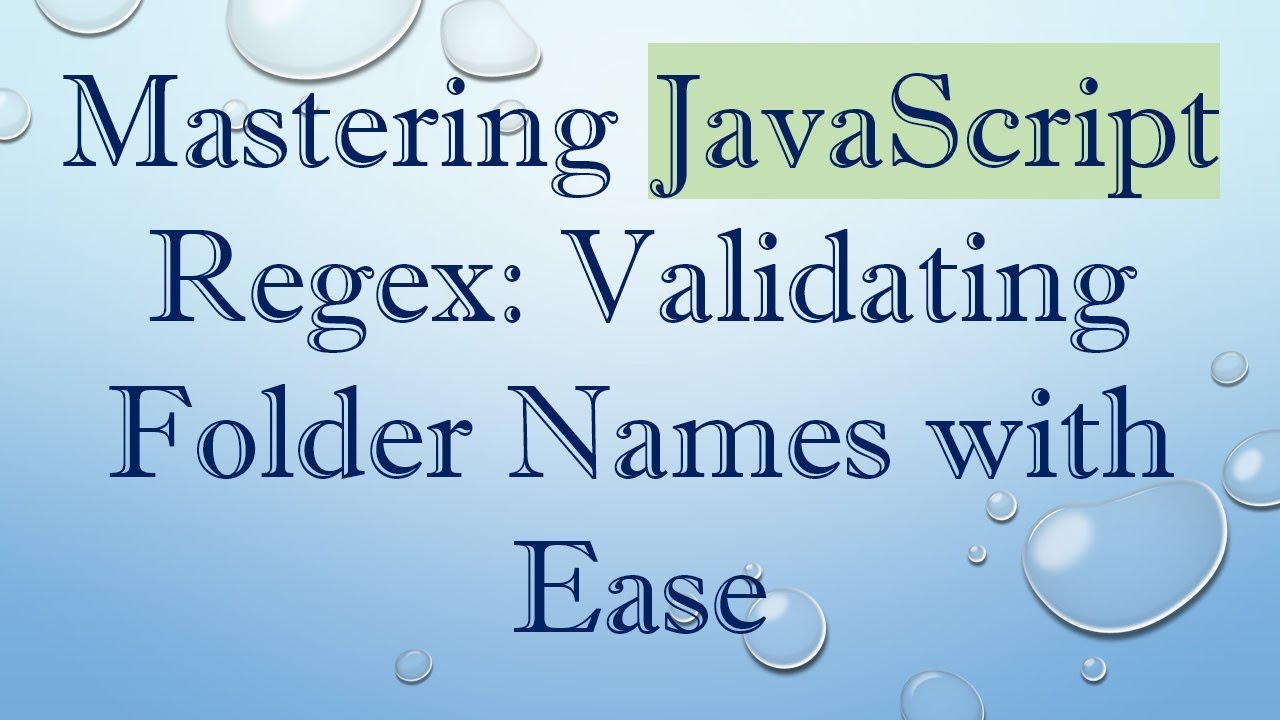
Показать описание
Discover how to craft a regex in JavaScript that validates folder names with specific rules, ensuring only valid characters and length constraints are accepted.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: JavaScript regex issue for folders names
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering JavaScript Regex for Folder Names: A Step-by-Step Guide
In today's tech-savvy world, coding often requires data validation, particularly when dealing with user inputs such as folder names. A common challenge is ensuring that folder names conform to specific rules: they should be alphanumeric and may include hyphens, underscores, and spaces, with constraints on formatting and length. In this guide, we will tackle a scenario where one attempts to create a regex in JavaScript to validate folder names, and we will identify and correct the issues in the initial code.
The Problem: Incorrect Regex Implementation
When you attempt to validate a folder name with a regex, a well-structured pattern is necessary. A user recently expressed frustration while trying to write such a regex and shared their attempt:
[[See Video to Reveal this Text or Code Snippet]]
Despite the intention, the regex always returns yes, which indicates that it is not functioning as expected. Let's break down the solution to fix this problem.
The Solution: Crafting the Right Regex
Understanding the Issues
Unnecessary Quantifier: The + quantifier in the initial regex pattern is included incorrectly. In context, it matches one or more occurrences of an alphanumeric character right at the start, but due to the nested character class, it's redundant. This leads to unexpected behavior in validation.
Length Constraint Adjustments: The maximum character limit is set to 20, but due to the way the regex is structured, the first character of the class is included in that count. Therefore, subsequent acceptable characters should only account for the remaining 19.
Revised Regex Pattern
To appropriately validate folder names, here's an improved regex pattern:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Regex
^: Asserts the start of the line. This ensures that the pattern begins matching from the very first character.
[a-zA-Z0-9]: This ensures that the first character is either a letter (uppercase or lowercase) or a digit — crucial for our folder name validation.
[a-zA-Z0-9_ -]{0,19}: This allows any alphanumeric character, underscore, space, or hyphen; and it restricts the total length to a maximum of 20 characters. Since the first character is already counted, we can only have 19 more characters following it.
$: Asserts the end of the line, making sure nothing beyond the defined structure is appended to the folder name.
Conclusion
Validating folder names doesn't have to be complicated once you grasp the basics of regex in JavaScript. By understanding the nuances of character classes and quantifiers, you can write a regex that suits your specific needs. With the adjustments provided, your folder name validation will work flawlessly. Remember to test your regex thoroughly with various inputs to ensure its robustness!
If you encounter any further questions about regex or JavaScript, feel free to ask. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: JavaScript regex issue for folders names
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering JavaScript Regex for Folder Names: A Step-by-Step Guide
In today's tech-savvy world, coding often requires data validation, particularly when dealing with user inputs such as folder names. A common challenge is ensuring that folder names conform to specific rules: they should be alphanumeric and may include hyphens, underscores, and spaces, with constraints on formatting and length. In this guide, we will tackle a scenario where one attempts to create a regex in JavaScript to validate folder names, and we will identify and correct the issues in the initial code.
The Problem: Incorrect Regex Implementation
When you attempt to validate a folder name with a regex, a well-structured pattern is necessary. A user recently expressed frustration while trying to write such a regex and shared their attempt:
[[See Video to Reveal this Text or Code Snippet]]
Despite the intention, the regex always returns yes, which indicates that it is not functioning as expected. Let's break down the solution to fix this problem.
The Solution: Crafting the Right Regex
Understanding the Issues
Unnecessary Quantifier: The + quantifier in the initial regex pattern is included incorrectly. In context, it matches one or more occurrences of an alphanumeric character right at the start, but due to the nested character class, it's redundant. This leads to unexpected behavior in validation.
Length Constraint Adjustments: The maximum character limit is set to 20, but due to the way the regex is structured, the first character of the class is included in that count. Therefore, subsequent acceptable characters should only account for the remaining 19.
Revised Regex Pattern
To appropriately validate folder names, here's an improved regex pattern:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Regex
^: Asserts the start of the line. This ensures that the pattern begins matching from the very first character.
[a-zA-Z0-9]: This ensures that the first character is either a letter (uppercase or lowercase) or a digit — crucial for our folder name validation.
[a-zA-Z0-9_ -]{0,19}: This allows any alphanumeric character, underscore, space, or hyphen; and it restricts the total length to a maximum of 20 characters. Since the first character is already counted, we can only have 19 more characters following it.
$: Asserts the end of the line, making sure nothing beyond the defined structure is appended to the folder name.
Conclusion
Validating folder names doesn't have to be complicated once you grasp the basics of regex in JavaScript. By understanding the nuances of character classes and quantifiers, you can write a regex that suits your specific needs. With the adjustments provided, your folder name validation will work flawlessly. Remember to test your regex thoroughly with various inputs to ensure its robustness!
If you encounter any further questions about regex or JavaScript, feel free to ask. Happy coding!