filmov
tv
Goodbye, useEffect: David Khourshid
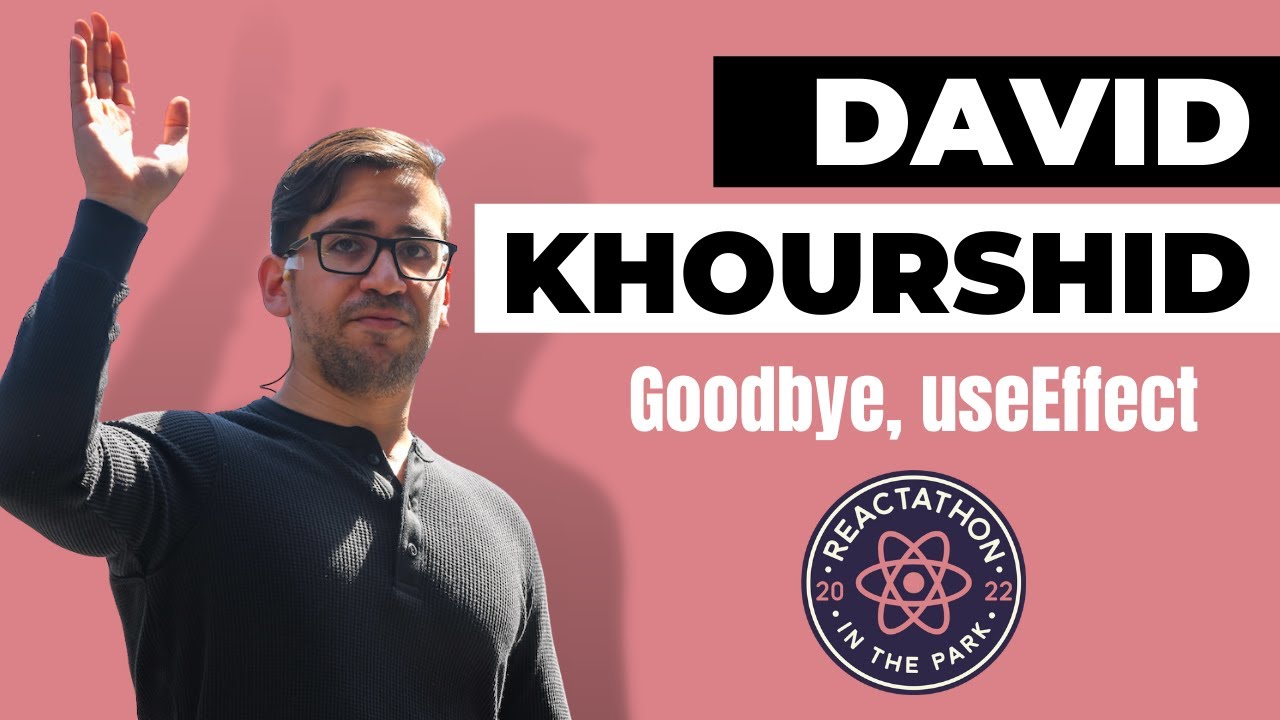
Показать описание
Goodbye, useEffect
From fetching data to fighting with imperative APIs, side effects are one of the biggest sources of frustration in web app development. And let’s be honest, putting everything in useEffect hooks doesn’t help much. Thankfully, there is a science (well, math) to side effects, formalized in state machines and statecharts, that can help us visually model and understand how to declaratively orchestrate effects, no matter how complex they get. In this talk, we’ll ditch the useEffect hook and discover how these computer science principles can be used to simplify effects in our React apps.
Aboud David Khourshid
David is a software engineer who loves playing piano and is passionate about animations, state machines, cutting-edge user interfaces, and open-source. Previously at Microsoft, he is now the founder of Stately, a startup focused on making even the most complex application logic visual and accessible to developers and non-developers alike.
Goodbye, useEffect - David Khourshid
Goodbye, useEffect: David Khourshid
David Khourshid @ ReactNext 22 - Goodbye, useEffect
Adiós, useEffect: Managing Effects More Effectively - DAVID KHOURSHID
Mastering useEffect: Avoid Using useEffect!
Learn React Hooks: useEffect - Simply Explained!
useEffect in 60 seconds #shorts #reactjs
Why I Don’t Use useEffect In My React Components
Mastering useEffect: Beware of depending on values you set
Stop Making This Mistake with the useEffect React Hook! #shorts
Using useEffect Effectively – David Khourshid, React Advanced London 2022
React's useEffect hook always confuses me
Fix React useEffect running twice in React 18
Mastering useEffect: Avoid Race Conditions
Mastering React's useEffect
In Defense Of useEffect - React's Most Dangerous Hook
React - Come usare useState & useEffect
How Does The useEffect Hook Work In React JS?
React useEffect tutorial (react hooks)
Episode 14: David Khourshid
useEffect/ReactJS - Poradnik
useWat - David Khourshid - React Rally 2023
useEffect in React Typescript
Mind Reading with Adaptive and Intelligent UIs in React — David Khourshid
Комментарии