filmov
tv
Load data on page scroll using jquery
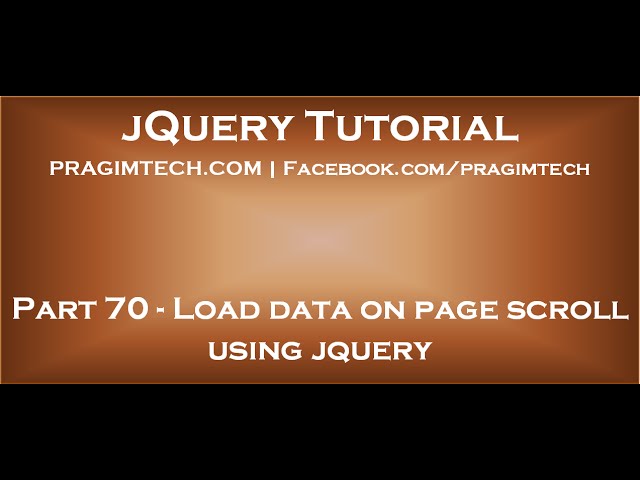
Показать описание
Link for all dot net and sql server video tutorial playlists
Link for slides, code samples and text version of the video
Healthy diet is very important both for the body and mind. If you like Aarvi Kitchen recipes, please support by sharing, subscribing and liking our YouTube channel. Hope you can help.
In this video we will discuss, how to load more data on page scroll using jQuery AJAX.
This is similar to Facebook. As you scroll down on the page more data will be loaded.
When the page is initially loaded we want to retrieve and display the first 50 rows from the database table tblEmployee. As we scroll down and when we hit the bottom of the page we want to load the next set of 50 rows.
Stored procedure
Create procedure spGetEmployees
@PageNumber int,
@PageSize int
as
Begin
Declare @StartRow int
Declare @EndRow int
Set @EndRow = @PageNumber * @PageSize;
WITH RESULT AS
(
SELECT Id, Name, Gender, Salary,
ROW_NUMBER() OVER (ORDER BY ID ASC) AS ROWNUMBER
From tblEmployee
)
SELECT *
FROM RESULT
WHERE ROWNUMBER BETWEEN @StartRow AND @EndRow
End
HTML page
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript">
$(document).ready(function () {
var currentPage = 1;
loadPageData(currentPage);
$(window).scroll(function () {
if ($(window).scrollTop() == $(document).height() - $(window).height()) {
currentPage += 1;
loadPageData(currentPage);
}
});
function loadPageData(currentPageNumber) {
$.ajax({
method: 'post',
dataType: "json",
data: { pageNumber: currentPageNumber, pageSize: 50 },
success: function (data) {
var employeeTable = $('#tblEmployee tbody');
$(data).each(function (index, emp) {
+ emp.Name + '</td><td>' + emp.Gender
+ '</td><td>' + emp.Salary + '</td></tr>');
});
},
error: function (err) {
alert(err);
}
});
}
});
</script>
</head>
<body style="font-family:Arial">
<h1>The data will be loaded on demand as you scroll down the page</h1>
<table id="tblEmployee" border="1" style="border-collapse:collapse; font-size:xx-large">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Gender</th>
<th>Salary</th>
</tr>
</thead>
<tbody></tbody>
</table>
</body>
</html>
Link for slides, code samples and text version of the video
Healthy diet is very important both for the body and mind. If you like Aarvi Kitchen recipes, please support by sharing, subscribing and liking our YouTube channel. Hope you can help.
In this video we will discuss, how to load more data on page scroll using jQuery AJAX.
This is similar to Facebook. As you scroll down on the page more data will be loaded.
When the page is initially loaded we want to retrieve and display the first 50 rows from the database table tblEmployee. As we scroll down and when we hit the bottom of the page we want to load the next set of 50 rows.
Stored procedure
Create procedure spGetEmployees
@PageNumber int,
@PageSize int
as
Begin
Declare @StartRow int
Declare @EndRow int
Set @EndRow = @PageNumber * @PageSize;
WITH RESULT AS
(
SELECT Id, Name, Gender, Salary,
ROW_NUMBER() OVER (ORDER BY ID ASC) AS ROWNUMBER
From tblEmployee
)
SELECT *
FROM RESULT
WHERE ROWNUMBER BETWEEN @StartRow AND @EndRow
End
HTML page
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript">
$(document).ready(function () {
var currentPage = 1;
loadPageData(currentPage);
$(window).scroll(function () {
if ($(window).scrollTop() == $(document).height() - $(window).height()) {
currentPage += 1;
loadPageData(currentPage);
}
});
function loadPageData(currentPageNumber) {
$.ajax({
method: 'post',
dataType: "json",
data: { pageNumber: currentPageNumber, pageSize: 50 },
success: function (data) {
var employeeTable = $('#tblEmployee tbody');
$(data).each(function (index, emp) {
+ emp.Name + '</td><td>' + emp.Gender
+ '</td><td>' + emp.Salary + '</td></tr>');
});
},
error: function (err) {
alert(err);
}
});
}
});
</script>
</head>
<body style="font-family:Arial">
<h1>The data will be loaded on demand as you scroll down the page</h1>
<table id="tblEmployee" border="1" style="border-collapse:collapse; font-size:xx-large">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Gender</th>
<th>Salary</th>
</tr>
</thead>
<tbody></tbody>
</table>
</body>
</html>
Комментарии