filmov
tv
Mastering TypeScript: How to Effectively Work with Variables of Different Data Types in TypeScript
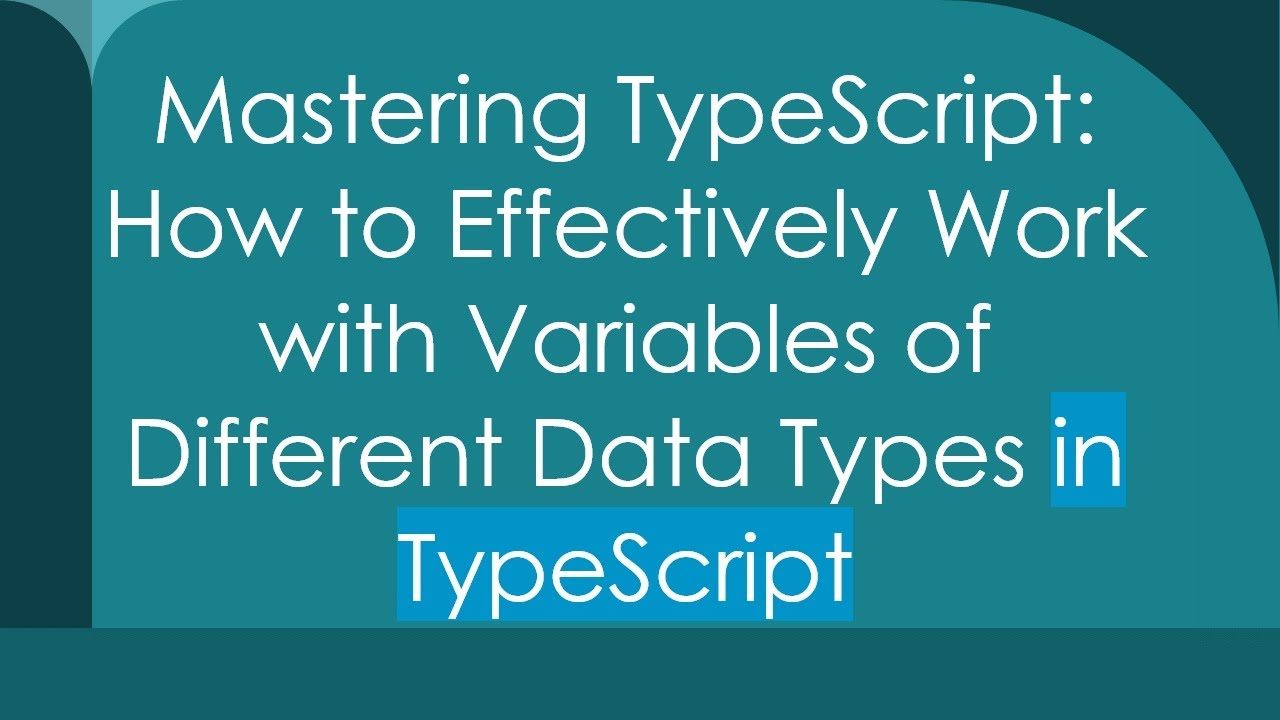
Показать описание
Discover how to handle variables with multiple data types in TypeScript. Learn effective techniques for type safety and data structure manipulation.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to work with variable with different datatype in Typescript?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering TypeScript: How to Effectively Work with Variables of Different Data Types in TypeScript
TypeScript is known for its strong typing, which means it allows developers to define types for their variables, making the code more predictable and less error-prone. However, working with variables that can hold multiple data types can sometimes be confusing. In this post, we’ll dive into a specific scenario: handling a variable that may either be an array of strings or an object with string arrays as properties. We'll explore strategies to avoid TypeScript errors and to ensure that our operations are type-safe.
Understanding the Problem
Consider the following variable declaration:
[[See Video to Reveal this Text or Code Snippet]]
This variable, campaigns, can be either:
An array of strings (string[])
An object where each property is an array of strings (e.g., { [key: string]: string[] })
When manipulating campaigns, if we know for sure that it’s an array, we might try to push a string into it like so:
[[See Video to Reveal this Text or Code Snippet]]
However, TypeScript throws an error, stating that we cannot use .push() on an object. The challenge here is determining how to let TypeScript understand that we are indeed working with an array at that point in our logic.
Solution Strategies
To solve the problem, we can utilize two powerful techniques in TypeScript: type checking and type guards.
Here is how you can implement it:
[[See Video to Reveal this Text or Code Snippet]]
Creating a Custom Type Checking Function
You may also opt to create a more specific type checking function. This method is particularly useful if you want a more reusable solution across your codebase.
Here's a custom function that checks if the variable is an array of strings:
[[See Video to Reveal this Text or Code Snippet]]
With this function, you can enhance your type-checking process as follows:
[[See Video to Reveal this Text or Code Snippet]]
What Does is Mean?
In the function above, the is keyword is a type predicate that tells TypeScript that when the function returns true, the arr variable should be treated as a string[]. This provides great clarity and control over how TypeScript handles the variable type, enhancing code safety and maintainability.
Conclusion
Embracing TypeScript’s features will help you build more robust applications and develop as a programmer. So, next time you find yourself dodging TypeScript errors when manipulating mixed-type variables, remember these techniques to conquer those challenges effectively!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to work with variable with different datatype in Typescript?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Mastering TypeScript: How to Effectively Work with Variables of Different Data Types in TypeScript
TypeScript is known for its strong typing, which means it allows developers to define types for their variables, making the code more predictable and less error-prone. However, working with variables that can hold multiple data types can sometimes be confusing. In this post, we’ll dive into a specific scenario: handling a variable that may either be an array of strings or an object with string arrays as properties. We'll explore strategies to avoid TypeScript errors and to ensure that our operations are type-safe.
Understanding the Problem
Consider the following variable declaration:
[[See Video to Reveal this Text or Code Snippet]]
This variable, campaigns, can be either:
An array of strings (string[])
An object where each property is an array of strings (e.g., { [key: string]: string[] })
When manipulating campaigns, if we know for sure that it’s an array, we might try to push a string into it like so:
[[See Video to Reveal this Text or Code Snippet]]
However, TypeScript throws an error, stating that we cannot use .push() on an object. The challenge here is determining how to let TypeScript understand that we are indeed working with an array at that point in our logic.
Solution Strategies
To solve the problem, we can utilize two powerful techniques in TypeScript: type checking and type guards.
Here is how you can implement it:
[[See Video to Reveal this Text or Code Snippet]]
Creating a Custom Type Checking Function
You may also opt to create a more specific type checking function. This method is particularly useful if you want a more reusable solution across your codebase.
Here's a custom function that checks if the variable is an array of strings:
[[See Video to Reveal this Text or Code Snippet]]
With this function, you can enhance your type-checking process as follows:
[[See Video to Reveal this Text or Code Snippet]]
What Does is Mean?
In the function above, the is keyword is a type predicate that tells TypeScript that when the function returns true, the arr variable should be treated as a string[]. This provides great clarity and control over how TypeScript handles the variable type, enhancing code safety and maintainability.
Conclusion
Embracing TypeScript’s features will help you build more robust applications and develop as a programmer. So, next time you find yourself dodging TypeScript errors when manipulating mixed-type variables, remember these techniques to conquer those challenges effectively!