filmov
tv
Math Quiz Game - Python Project
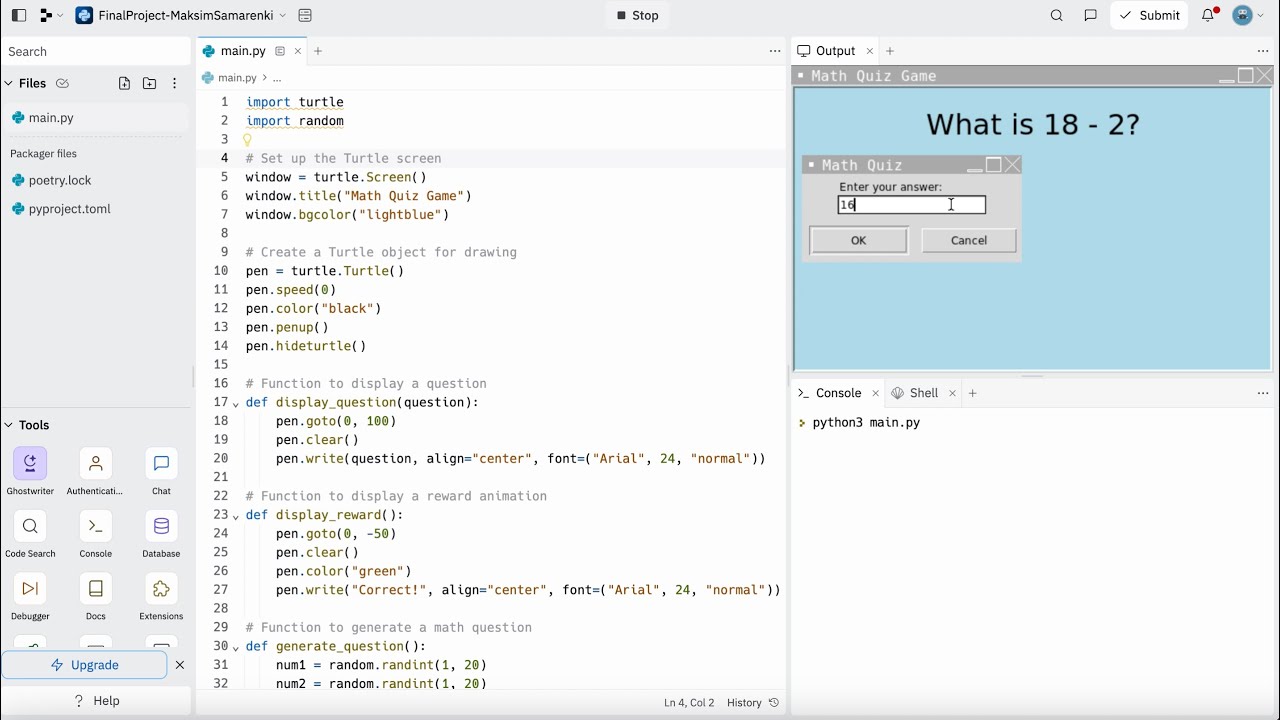
Показать описание
#python #pythonprojects #pythonprogramming #pythonproject #pythonforbeginners #pythongame #pythonquizgame #mathquizgame
Math Quiz Game - Python Project by Maksim Samarenkin.
Math Quiz Game - Python Project by Maksim Samarenkin.
Python Math Quiz
Create a QUIZ GAME with Python 💯
Python Maths Quiz Generator
Create Math Game Using Python
Build a Math Game Quiz | Python Tutorial for Beginners
How To Program a Maths Quiz in Python | Explained For Beginners
How To Create Math Game For Kids With Python (Tkinter)
Writing a Simple Maths Quiz in Python
Math Quiz Game using Python | Python Exercises #12
how to make a math quiz game project using python|step by step tutorial for beginner
Math Quiz Game - Python Project
Learn Python with ChatGPT | Math Quiz Game
Python Programming - Creating A Maths Quiz
Maths Quiz program in python using Tkinter
Building a Quiz App with Python and Tkinter | Tutorial
Python Math Quiz | Python tutorial for Beginners
Basic Python Math Quiz
Normal People VS Programmers #coding #python #programming #easy #funny #short
MathQuiz Game In Python! | No Talking | Beginner Friendly | Part 1
Python Mini-Projects - a Maths Quiz Game. Introduction
5.11. (Part 1) Math Quiz - Python
Python-based math quiz game
Python Maths Quiz Help
Adding Score in Math Quiz | Math Quiz Python Tkinter - 7
Комментарии