filmov
tv
Write a Program to find the sum of values located at Memory location in 8051 Microcontroller.
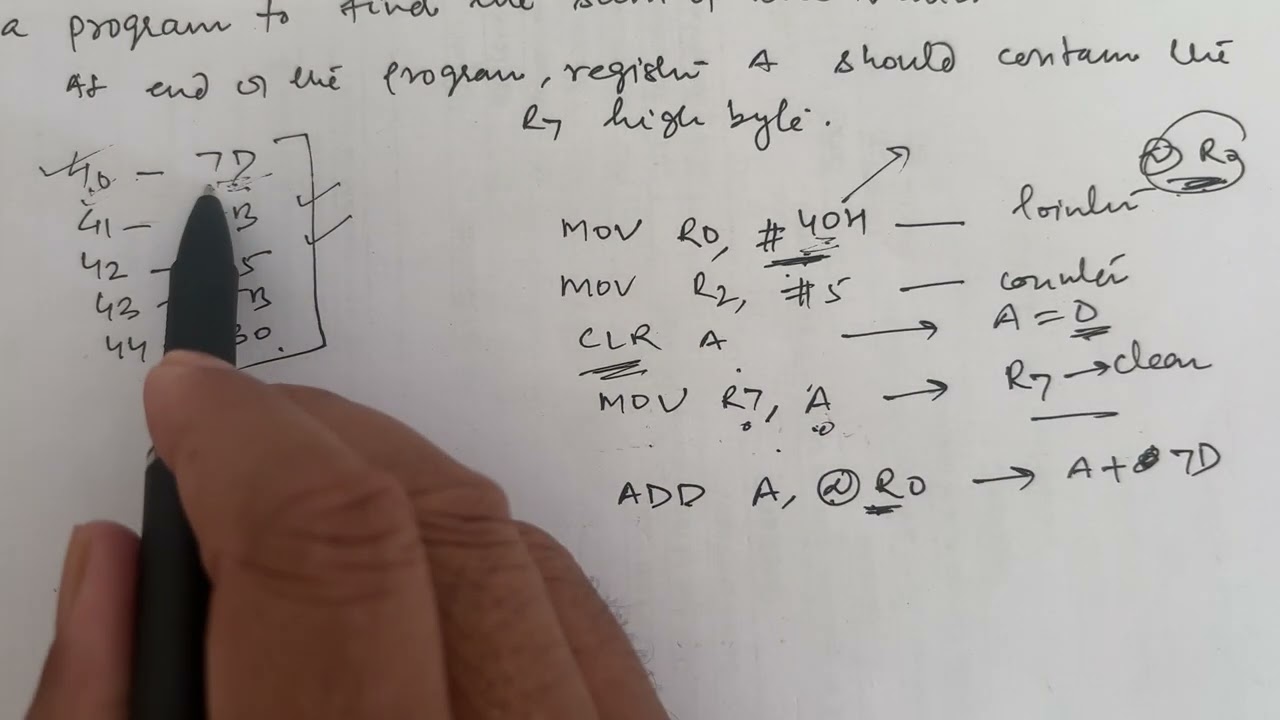
Показать описание
#co #4yeardegree #academic #microcontroller_programming
#8051Microcontroller
Write a program to find the sum of values located at memory location and store the output in Register. Lower bits in different register and higher bits in different register.
Sure, I'll provide an assembly program for the 8051 microcontroller that sums the values stored in a series of memory locations. The example will assume the values are stored in consecutive memory locations starting from a base address, and the number of values to sum is specified.
Here's an assembly program to achieve this:
; Program to find the sum of values located at memory locations in 8051 Microcontroller
ORG 0H ; Origin, sets the program starting address to 0
MOV DPTR, #300H ; Load the base address of the memory locations into DPTR (e.g., 300H)
MOV R2, #10 ; Load the number of values to sum into R2 (e.g., summing 10 values)
MOV A, #00H ; Clear accumulator to hold the sum
SUM_LOOP: ; Loop label
MOVX A, @DPTR ; Move the value at the address in DPTR to accumulator
ADD A, B ; Add the value in B to accumulator
INC DPTR ; Increment DPTR to point to the next memory location
DJNZ R2, SUM_LOOP ; Decrement R2, if it's not zero, jump to SUM_LOOP
END_SUM: ; End of the program
NOP ; No operation, used to end the program cleanly
END ; End directive
Explanation:
ORG 0H: Sets the origin of the program to address 0H.
MOV DPTR, #300H: Loads the Data Pointer (DPTR) with the starting address (300H in this case).
MOV R2, #10: Loads the number of values to sum (e.g., 10 values) into register R2.
MOV A, #00H: Clears the accumulator (A) to start with a sum of 0.
SUM_LOOP: Label for the loop.
MOVX A, @DPTR: Moves the value from the external memory location pointed to by DPTR into the accumulator.
ADD A, B: Adds the value in register B to the accumulator.
INC DPTR: Increments DPTR to point to the next memory location.
DJNZ R2, SUM_LOOP: Decrements R2 and jumps to SUM_LOOP if R2 is not zero.
END_SUM: Label marking the end of the summing loop.
NOP: No operation, a placeholder for ending the program cleanly.
END: End of the program directive.
Notes:
This example assumes that the external memory is mapped and accessible using the DPTR and MOVX instructions.
You may need to adjust the base address (300H) and the number of values (10) as per your specific requirements.
The sum result will be stored in the accumulator (A).
Feel free to modify the program according to your specific needs and memory layout.
#8051Microcontroller
Write a program to find the sum of values located at memory location and store the output in Register. Lower bits in different register and higher bits in different register.
Sure, I'll provide an assembly program for the 8051 microcontroller that sums the values stored in a series of memory locations. The example will assume the values are stored in consecutive memory locations starting from a base address, and the number of values to sum is specified.
Here's an assembly program to achieve this:
; Program to find the sum of values located at memory locations in 8051 Microcontroller
ORG 0H ; Origin, sets the program starting address to 0
MOV DPTR, #300H ; Load the base address of the memory locations into DPTR (e.g., 300H)
MOV R2, #10 ; Load the number of values to sum into R2 (e.g., summing 10 values)
MOV A, #00H ; Clear accumulator to hold the sum
SUM_LOOP: ; Loop label
MOVX A, @DPTR ; Move the value at the address in DPTR to accumulator
ADD A, B ; Add the value in B to accumulator
INC DPTR ; Increment DPTR to point to the next memory location
DJNZ R2, SUM_LOOP ; Decrement R2, if it's not zero, jump to SUM_LOOP
END_SUM: ; End of the program
NOP ; No operation, used to end the program cleanly
END ; End directive
Explanation:
ORG 0H: Sets the origin of the program to address 0H.
MOV DPTR, #300H: Loads the Data Pointer (DPTR) with the starting address (300H in this case).
MOV R2, #10: Loads the number of values to sum (e.g., 10 values) into register R2.
MOV A, #00H: Clears the accumulator (A) to start with a sum of 0.
SUM_LOOP: Label for the loop.
MOVX A, @DPTR: Moves the value from the external memory location pointed to by DPTR into the accumulator.
ADD A, B: Adds the value in register B to the accumulator.
INC DPTR: Increments DPTR to point to the next memory location.
DJNZ R2, SUM_LOOP: Decrements R2 and jumps to SUM_LOOP if R2 is not zero.
END_SUM: Label marking the end of the summing loop.
NOP: No operation, a placeholder for ending the program cleanly.
END: End of the program directive.
Notes:
This example assumes that the external memory is mapped and accessible using the DPTR and MOVX instructions.
You may need to adjust the base address (300H) and the number of values (10) as per your specific requirements.
The sum result will be stored in the accumulator (A).
Feel free to modify the program according to your specific needs and memory layout.