filmov
tv
Learn JavaScript STATIC keyword in 8 minutes! ⚡
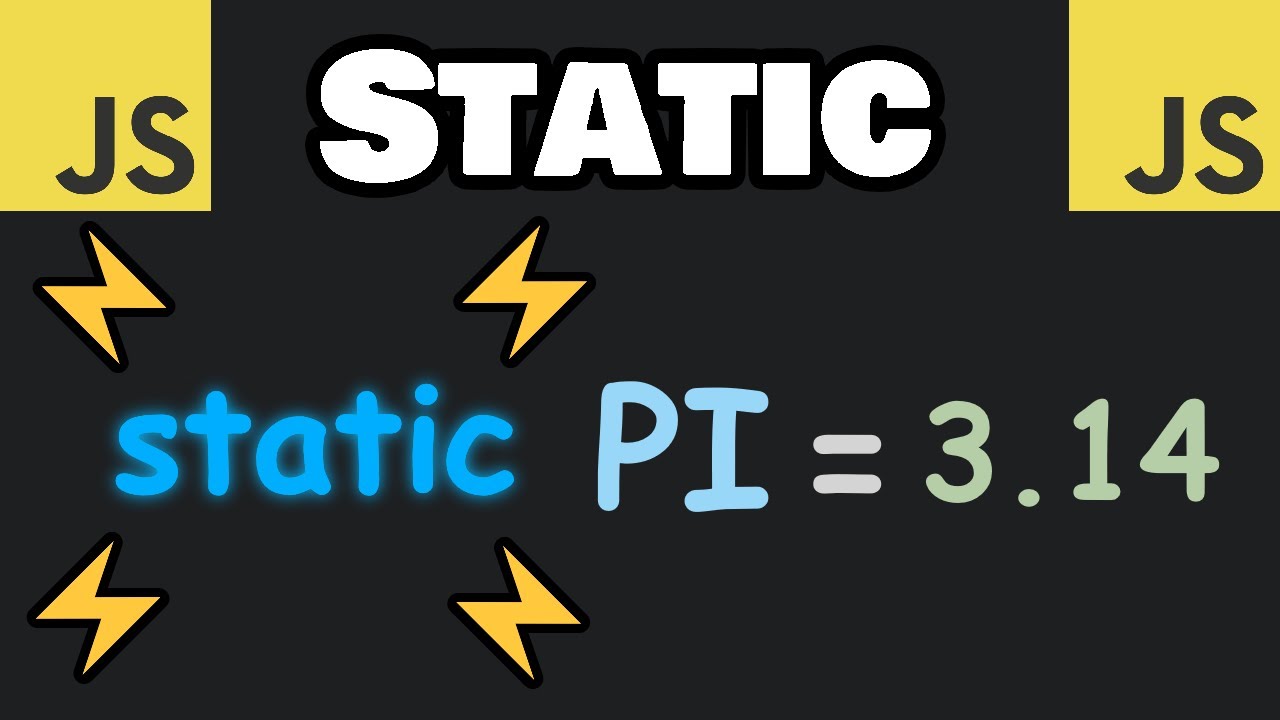
Показать описание
// static = keyword that defines properties or methods that belong
// to a class itself rather than the objects created
// from that class (class owns anything static, not the objects)
// ------------ EXAMPLE 1 ------------
class MathUtil{
static PI = 3.14159;
static getDiameter(radius){
return radius * 2;
}
static getCircumference(radius){
return 2 * this.PI * radius;
}
static getArea(radius){
return this.PI * radius * radius;
}
}
// ------------ EXAMPLE 2 ------------
class User{
static userCount = 0;
constructor(username){
}
static getUserCount(){
}
sayHello(){
}
}
const user1 = new User("Spongebob");
const user2 = new User("Patrick");
const user3 = new User("Sandy");
// to a class itself rather than the objects created
// from that class (class owns anything static, not the objects)
// ------------ EXAMPLE 1 ------------
class MathUtil{
static PI = 3.14159;
static getDiameter(radius){
return radius * 2;
}
static getCircumference(radius){
return 2 * this.PI * radius;
}
static getArea(radius){
return this.PI * radius * radius;
}
}
// ------------ EXAMPLE 2 ------------
class User{
static userCount = 0;
constructor(username){
}
static getUserCount(){
}
sayHello(){
}
}
const user1 = new User("Spongebob");
const user2 = new User("Patrick");
const user3 = new User("Sandy");
Learn JavaScript STATIC keyword in 8 minutes! ⚡
Learn Javascript | Static Methods
JavaScript Classes Static Methods
What are Static methods in JavaScript
Static Method in JavaScript | JavaScript Tutorial in Hindi #81
Part 15- OOPS - static keyword in JavaScript
Everything a programmer needs to know about the STATIC keyword in JavaScript
Learn JavaScript Episode #22: Class Constructor, Instance Variables, and Static Variables
Learn JavaScript CLASSES in 6 minutes! 🏭
Learn JavaScript Static 🛠️(JavaScript tutorial #15)
Learn JavaScript OOP in Arabic #21 - Class Static Properties And Methods
#4.6 Java Tutorial | Static Keyword
What is Static Variable & Static Methods - OOPS #3 | JavaScript | Protractor | | LetCode
static methods in javascript - shorts
JavaScript - static keyword, static properties and static methods
How to Use Static Methods in Javascript ES6 - 16
Javascript : Class Static methods on objects
Static Variable IN JAVA #shorts #study #javascript
'Mastering the 'Static' Keyword in JavaScript Classes: A Deeper Dive' |Core Conc...
JS part 18- OOPs :Static keyword
Static Keyword - Dart Programming
static keyword in javascript classes courses #javascript #js #webdeveloper
JavaScript Tutorial for Beginners: Learn JavaScript in 1 Hour
JavaScript Class Inheritance | Static And Private Methods In JavaScript
Комментарии