filmov
tv
Find Second Maximum value in an Array | Animation
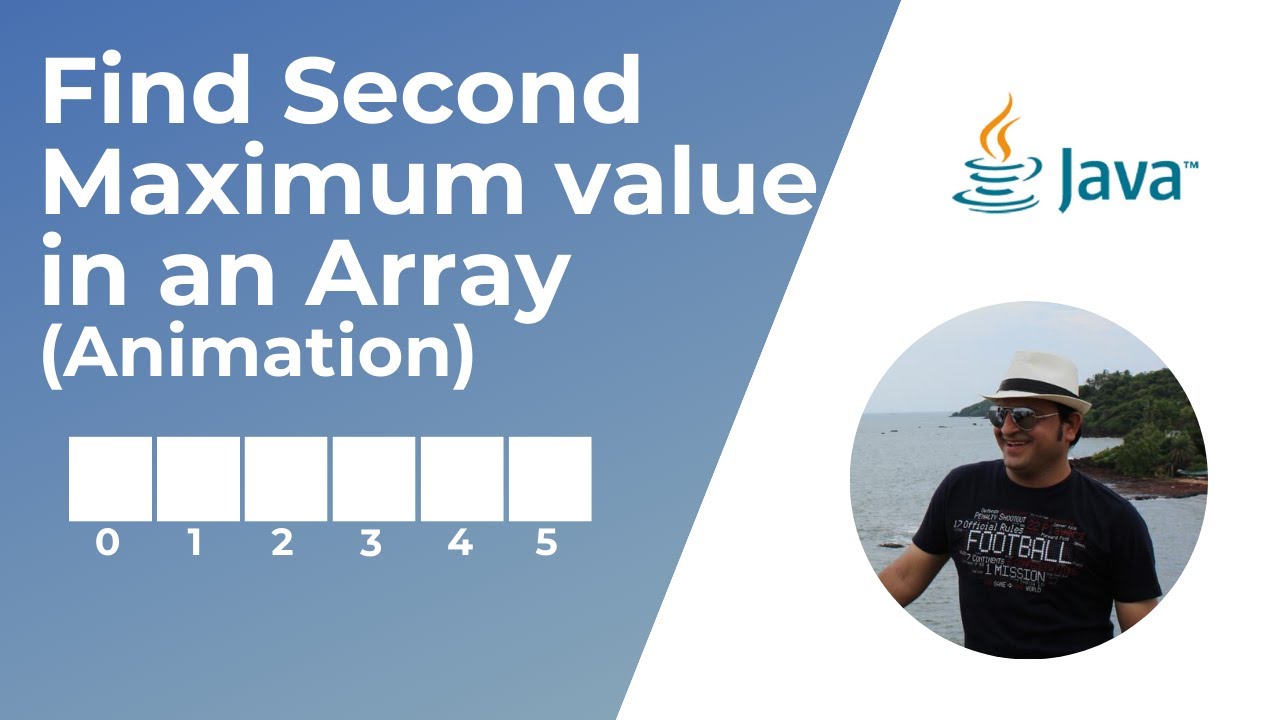
Показать описание
Watch all my playlist here:
Want to land a software engineering job in the IT industry? This course - 'Visualizing Data Structures and Algorithms' is here to help. The course walks you through multiple Java algorithms, data structures problems, and their solutions with step by step visualizations, so that you are actually learning instead of blindly memorizing solutions.
The course covers in and outs of Data Structures and Algorithms in Java. Java is used as the programming language in the course. Students familiar with Javascript, Python, C#, C++, C, etc will also get to learn concepts without any difficulty. The implementation of various Algorithms and Data Structures have been demonstrated and implemented through animated slides. It covers many interview room questions on Algorithms and Data Structures. The questions and solutions are demonstrated by -
1. Animated slide. (To make visualization of algorithms faster)
2. Coding algorithm on IDE.
The course covers topics such as -
0. Algorithm Analysis
1. Arrays
2. Matrix
3. Singly Linked List
4. Doubly Linked List
5. Circular Singly Linked List
6. Stacks
7. Queues
8. Binary Tree
9. Binary Search Tree
10. Graphs
11. Priority Queues and Heaps
12. Recursion
13. Searching
14. Sorting
15. Strings
16. Trie Data Structure
17. Dynamic Programming
and many more ...
#dsa #algorithms #coding
Комментарии