filmov
tv
Understanding the TypeError: 'NoneType' object not iterable in Python
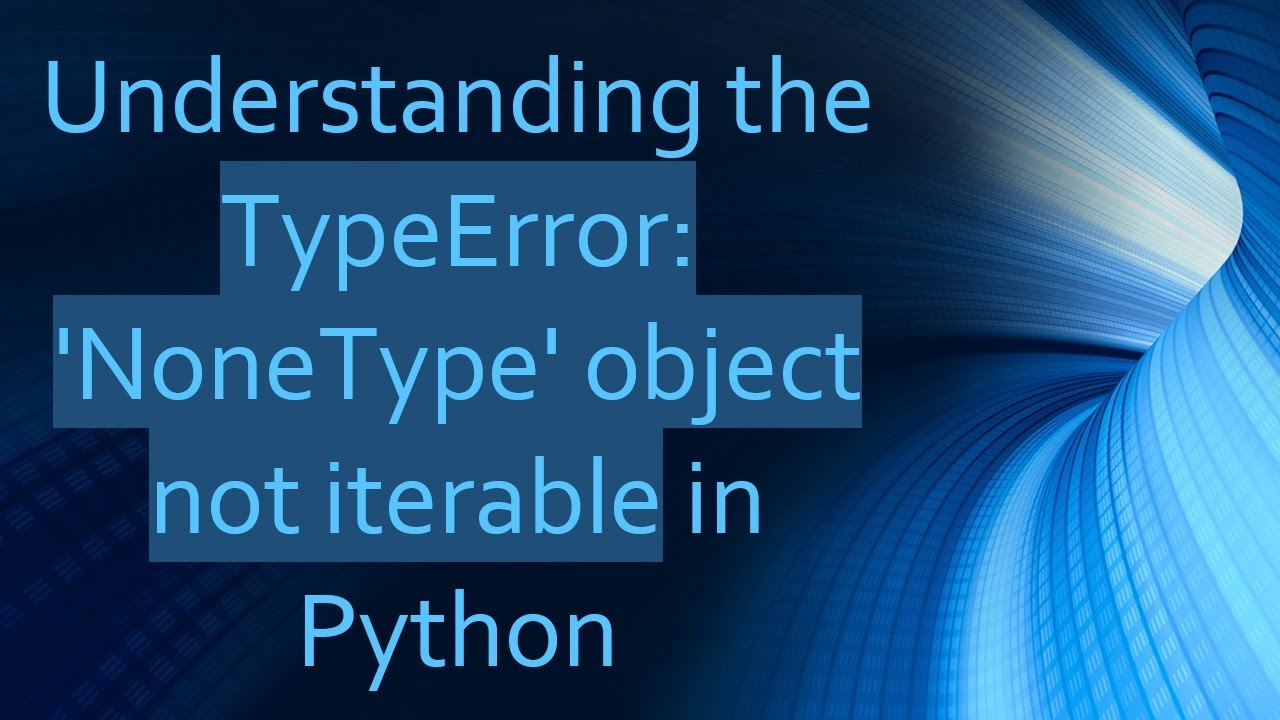
Показать описание
Learn how to troubleshoot and fix the `TypeError: 'NoneType' object not iterable` in Python. Discover the common causes and simple solutions to enhance your coding skills.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python TypeError: 'NoneType' object not iterable - What's causing it?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the TypeError: 'NoneType' object not iterable in Python
Encounters with errors are a part of any programmer's journey, and Python's TypeError: 'NoneType' object is not iterable can be particularly perplexing. This error typically indicates that you are attempting to loop through a variable that is set to None, a situation that can arise in various coding scenarios. In this post, we’ll delve into what causes this error and how to effectively fix it.
The Problem: Understanding the Error
Here’s a scenario that might seem familiar. You have a script comprised of several functions that work synergistically to process data. Upon execution, you find that the following error message appears:
[[See Video to Reveal this Text or Code Snippet]]
This message often occurs when a function mistakenly returns None, and the subsequent code attempts to iterate over that return value. Let's take a closer look at the sample code below that illustrates this problem:
[[See Video to Reveal this Text or Code Snippet]]
In the above example, during the call to process_data, if input_data is None, a TypeError occurs while trying to loop through it.
The Cause: Where It Goes Wrong
The heart of the issue lies in the get_input_data() function. If this function does not successfully return a list (or any other iterable), it defaults to returning None, leading to the error when process_data attempts to iterate over this NoneType.
Potential Causes of Returning None:
Uninitialized Variable: If input_data is not properly assigned before the return statement.
Error in Data Retrieval Logic: If there's a problem in the logic that fetches or generates the data.
The Solution: Handling NoneType
To resolve the error, a simple yet effective safeguard can be introduced in the process_data function. Here’s how you can modify the function to handle the NoneType gracefully:
[[See Video to Reveal this Text or Code Snippet]]
Key Changes Made:
Check for None: Before attempting to iterate, we check if data is None. If it is, we return an empty list to avoid the error.
Return Value Adjustment: This avoids the crash of your program and allows it to handle that situation gracefully.
Conclusion
In summary, the error message TypeError: 'NoneType' object is not iterable is a common yet fixable issue in Python. By ensuring your functions return valid iterables, and by checking for None, you can enhance the robustness of your code. With these strategies in your toolbox, you'll be better equipped to handle similar issues in your future programming endeavors.
This understanding not only aids in troubleshooting current scripts but also fosters cleaner coding practices for the future. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Python TypeError: 'NoneType' object not iterable - What's causing it?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the TypeError: 'NoneType' object not iterable in Python
Encounters with errors are a part of any programmer's journey, and Python's TypeError: 'NoneType' object is not iterable can be particularly perplexing. This error typically indicates that you are attempting to loop through a variable that is set to None, a situation that can arise in various coding scenarios. In this post, we’ll delve into what causes this error and how to effectively fix it.
The Problem: Understanding the Error
Here’s a scenario that might seem familiar. You have a script comprised of several functions that work synergistically to process data. Upon execution, you find that the following error message appears:
[[See Video to Reveal this Text or Code Snippet]]
This message often occurs when a function mistakenly returns None, and the subsequent code attempts to iterate over that return value. Let's take a closer look at the sample code below that illustrates this problem:
[[See Video to Reveal this Text or Code Snippet]]
In the above example, during the call to process_data, if input_data is None, a TypeError occurs while trying to loop through it.
The Cause: Where It Goes Wrong
The heart of the issue lies in the get_input_data() function. If this function does not successfully return a list (or any other iterable), it defaults to returning None, leading to the error when process_data attempts to iterate over this NoneType.
Potential Causes of Returning None:
Uninitialized Variable: If input_data is not properly assigned before the return statement.
Error in Data Retrieval Logic: If there's a problem in the logic that fetches or generates the data.
The Solution: Handling NoneType
To resolve the error, a simple yet effective safeguard can be introduced in the process_data function. Here’s how you can modify the function to handle the NoneType gracefully:
[[See Video to Reveal this Text or Code Snippet]]
Key Changes Made:
Check for None: Before attempting to iterate, we check if data is None. If it is, we return an empty list to avoid the error.
Return Value Adjustment: This avoids the crash of your program and allows it to handle that situation gracefully.
Conclusion
In summary, the error message TypeError: 'NoneType' object is not iterable is a common yet fixable issue in Python. By ensuring your functions return valid iterables, and by checking for None, you can enhance the robustness of your code. With these strategies in your toolbox, you'll be better equipped to handle similar issues in your future programming endeavors.
This understanding not only aids in troubleshooting current scripts but also fosters cleaner coding practices for the future. Happy coding!