filmov
tv
Check If a String Contains All Binary Codes of Size K | Leetcode 1461
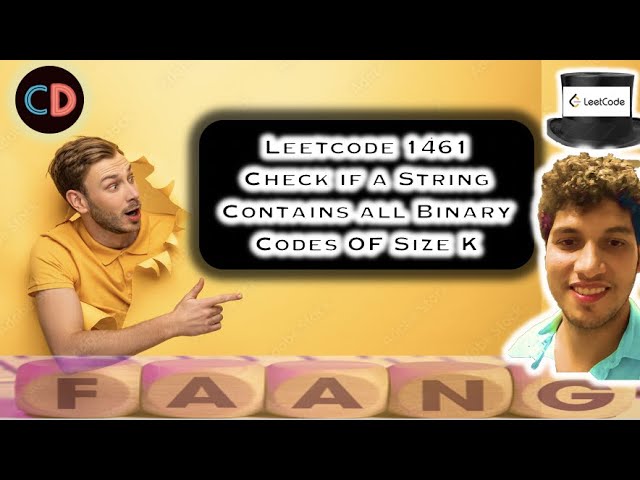
Показать описание
1) 0:00 Explaining the problem out loud
2) 1:10 Brute Force
3) 3:10 Optimised Approach
4) 7:15 Coding it up
5) 9:00 Complexity analysis
Check if a String Contains all Binary Codes of Size K - Leetcode 1461 - Python
How to search for a string or check if cell contains a specific text
How to check if a string contains a specific word in PHP | PHP Tutorial
How to check if a string contains a substring in JavaScript
How to check whether a string contains other String or not?
How to use regex to check if a javascript string contains a pattern #shorts
Regular Expressions - How to Check if a String Contains a Number in Python
Check if a string contains only digits | C# Coding | NerdHouseGeeks.com
Working with dictionaries in Python part 293
How To check if string contains a substring - Python
Check If a String Contains a Substring in Bash
Code 61: Check if a string contains only numbers | isnumeric() in Python | 365 Days of Code
How to check if a string contains a substring in Python | Python check if string contain a substring
Check If a String Contains All Binary Codes of Size K | Leetcode 1461
JavaScript String Contains: How to check a string exists in another
Leetcode 1461. Check If a String Contains All Binary Codes of Size K
Check if string contains both letter and number
Check If a String Contains All Binary Codes of Size K | Leetcode 1461 | String
Check If A String Contains A Substring In Python
Check if a string contains numeric value or character in Python-Python programming for beginners
Check If String Contains Any Letters from Alphabet in Python (Example) | any() & isalpha() Funct...
Check If a String Contains Any Digits Using Java
Python How to Check if string contains substring
javascript - Check if string contains only digits
Комментарии