filmov
tv
How to add attributes to Nodes, Edges and Graph in Python | NetworkX Tutorial - Part 03
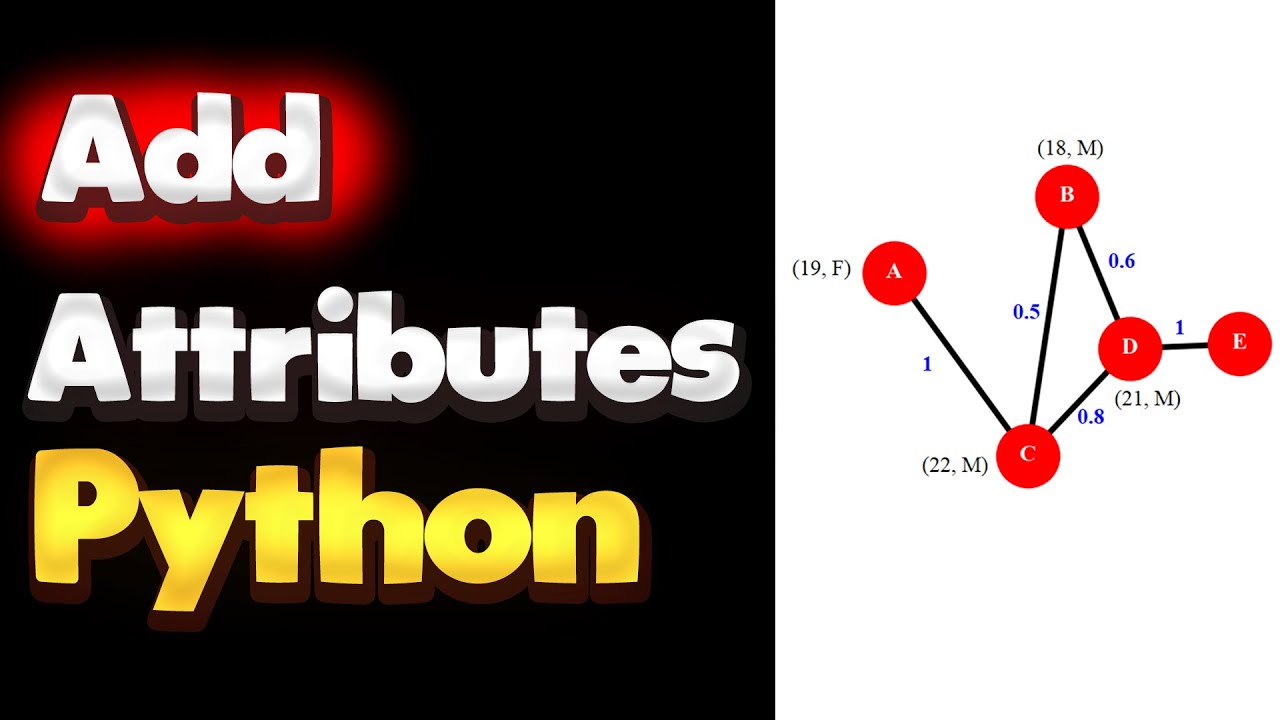
Показать описание
In this video, we will cover Attributed Graphs (Attributed Networks). We will talk about a small example of social network and we will add features (attributes) to nodes and also edges of the network/graph. Also we will add attribute to the graph (which is called graph-level attribute). So to summarize: in this video we will talk about how to add node-level features/attributes, edge-level attributes/features and also graph-level attributes/features in Python programming language using the famous Networkx package.
==================================
Networkx Tutorial Playlist:
==================================
==================================
NumPy Tutorial Playlist:
==================================
==================================
Suggested Videos:
==================================
🟥🟨 Previous Part of this Series:
How to add nodes and edges to a graph using Python NetworkX:
🟥🟨 Next Part of this Series:
Get Node Degree and Node Neighbors in Python NetworkX:
==================================
Source Code [Method 1]:
==================================
import networkx as nx
G=nx.Graph()
G.add_node("A",Age=19,Gender="F")
G.add_node("B",Age=18,Gender="M")
G.add_node("C",Age=22,Gender="M")
G.add_node("D",Age=21,Gender="M")
G.add_node("E",Age=20,Gender="F")
G.add_edge("A","C",weight=1)
G.add_edge("B","C",weight=0.5)
G.add_edge("B","D",weight=0.6)
G.add_edge("C","D",weight=0.8)
G.add_edge("D","E",weight=1)
pos={
"A":(1,5),
"B":(4.5,6.6),
"C":(3.6,1.4),
"D":(5.8,3.5),
"E":(7.9,3.6)
}
print("==============================================")
print(G.nodes["C"])
print(G.edges[("B","D")])
print("==============================================")
node_color="red",node_size=3000,
font_color="white",font_size=20,font_family="Times New Roman", font_weight="bold",
width=5)
==================================
Source Code [Method 2]:
==================================
import networkx as nx
G=nx.Graph()
G.graph["Name"]="My Graph"
G.add_nodes_from([
("A",{"Age":19,"Gender":"F"}),
("B",{"Age":18,"Gender":"M"}),
("C",{"Age":22,"Gender":"M"}),
("D",{"Age":21,"Gender":"M"}),
("E",{"Age":20,"Gender":"F"})
])
G.add_edges_from([
("A","C",{"weight":1}),
("B","C",{"weight":0.5}),
("B","D",{"weight":0.6}),
("C","D",{"weight":0.8}),
("D","E",{"weight":1})
])
pos={
"A":(1,5),
"B":(4.5,6.6),
"C":(3.6,1.4),
"D":(5.8,3.5),
"E":(7.9,3.6)
}
print("==============================================")
print(G.nodes["C"])
print(G.edges[("B","D")])
print("==============================================")
node_color="red",node_size=3000,
font_color="white",font_size=20,font_family="Times New Roman", font_weight="bold",
width=5)
==================================
Networkx Tutorial Playlist:
==================================
==================================
NumPy Tutorial Playlist:
==================================
==================================
Suggested Videos:
==================================
🟥🟨 Previous Part of this Series:
How to add nodes and edges to a graph using Python NetworkX:
🟥🟨 Next Part of this Series:
Get Node Degree and Node Neighbors in Python NetworkX:
==================================
Source Code [Method 1]:
==================================
import networkx as nx
G=nx.Graph()
G.add_node("A",Age=19,Gender="F")
G.add_node("B",Age=18,Gender="M")
G.add_node("C",Age=22,Gender="M")
G.add_node("D",Age=21,Gender="M")
G.add_node("E",Age=20,Gender="F")
G.add_edge("A","C",weight=1)
G.add_edge("B","C",weight=0.5)
G.add_edge("B","D",weight=0.6)
G.add_edge("C","D",weight=0.8)
G.add_edge("D","E",weight=1)
pos={
"A":(1,5),
"B":(4.5,6.6),
"C":(3.6,1.4),
"D":(5.8,3.5),
"E":(7.9,3.6)
}
print("==============================================")
print(G.nodes["C"])
print(G.edges[("B","D")])
print("==============================================")
node_color="red",node_size=3000,
font_color="white",font_size=20,font_family="Times New Roman", font_weight="bold",
width=5)
==================================
Source Code [Method 2]:
==================================
import networkx as nx
G=nx.Graph()
G.graph["Name"]="My Graph"
G.add_nodes_from([
("A",{"Age":19,"Gender":"F"}),
("B",{"Age":18,"Gender":"M"}),
("C",{"Age":22,"Gender":"M"}),
("D",{"Age":21,"Gender":"M"}),
("E",{"Age":20,"Gender":"F"})
])
G.add_edges_from([
("A","C",{"weight":1}),
("B","C",{"weight":0.5}),
("B","D",{"weight":0.6}),
("C","D",{"weight":0.8}),
("D","E",{"weight":1})
])
pos={
"A":(1,5),
"B":(4.5,6.6),
"C":(3.6,1.4),
"D":(5.8,3.5),
"E":(7.9,3.6)
}
print("==============================================")
print(G.nodes["C"])
print(G.edges[("B","D")])
print("==============================================")
node_color="red",node_size=3000,
font_color="white",font_size=20,font_family="Times New Roman", font_weight="bold",
width=5)
Комментарии