filmov
tv
Javascript Mock Interview | online video interview Questions & Answers
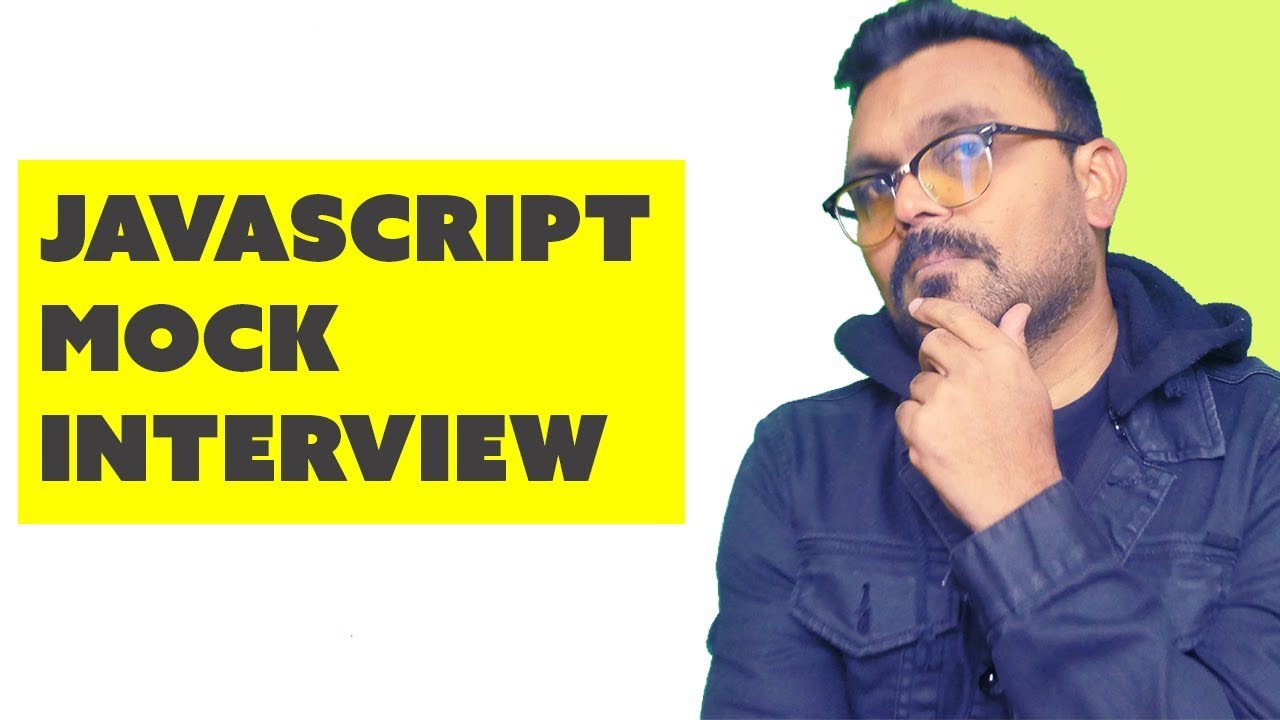
Показать описание
Online Technical Mock Interview of one of my viewers. Where I ask JavaScript Fundamental Questions , algorithm questions aand give their answers
#javaScript #interview #questions
*My Udemy Courses
Follow me for technology updates
Help me translate this video.
#javaScript #interview #questions
*My Udemy Courses
Follow me for technology updates
Help me translate this video.
JavaScript Mock Interview | Online Interview | Questions and Answers
JavaScript Mock Interview 2024 - Live Coding
Javascript Mock Interview | online video interview Questions & Answers
Mock Technical Interview - Javascript Developer Entry Level
JavaScript Mock Interview | Online Interview Questions and Answers
JavaScript Mock Interview | Interview Questions for Senior JavaScript Developers | Part 1
Must Know Javascript Interview Questions
Software Engineering Job Interview – Full Mock Interview
How to NOT Fail a Technical Interview
Fresher's Frontend Interview 🎉 | JavaScript | #reactjs (Mock) [Most Asked Questions -2023]
JavaScript Mock Interview | Online Interview Questions and Answers (Part 2)
JavaScript live interview | JavaScript interview for beginners #javascript
Javascript LIVE Coding Interview (Mock) #javascript #reactjs
Fresher's Frontend | Javascript 🎉 | ReactJs Interview (Mock) [Most Asked Questions -2022]
React JS Live Coding Interview 2023 - Cracking the Interview (Mock practice)
React.js Interview | Frontend Developer Interview | 0-3 Years | Javascript | React.js mock Interview
Top 25 JavaScript Interview Questions for Beginners
JavaScript LIVE Coding Interview Round (Mock)
JavaScript Mock Interview | Online Interview Questions and Answers (Part 4))
Front End Mock Interview | Online Interview | Questions and Answers
Google Frontend Interview With A Frontend Expert
JavaScript Mock Interview | Online Interview Questions and Answers (Part 3)
2.5 Years Experienced Best JavaScript Interview
Frontend javascript mock interview | Ep. 4
Комментарии